Implementing Undos and Redos with Command Design Pattern
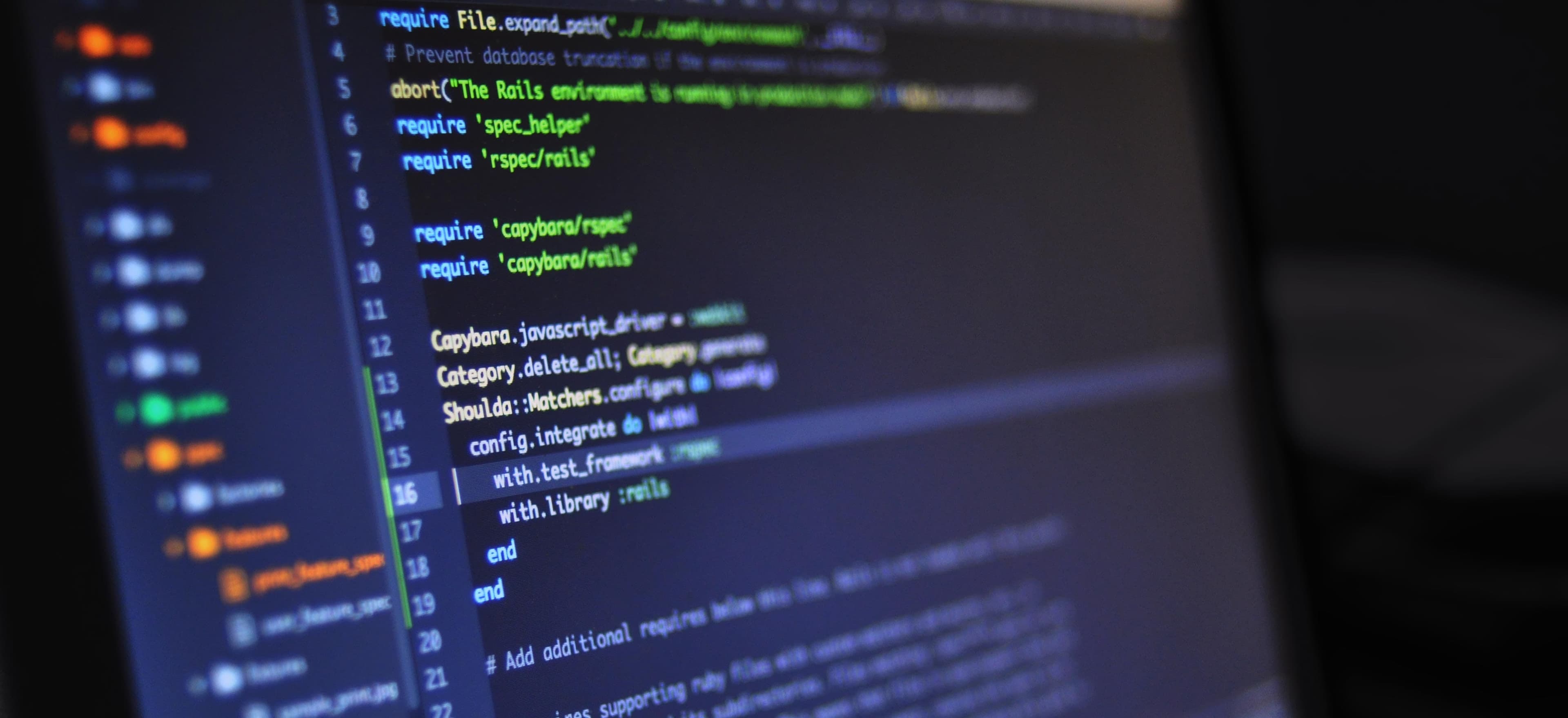
- Published on
Adding Undo and Redo Functionality to Java Applications Using Command Design Pattern
As developers, we often face the challenge of implementing undo and redo functionality in our applications. This becomes particularly challenging when the application involves complex user interactions, such as graphic editors, text editors, or any software with a high level of interactivity. The Command Design Pattern offers a robust solution to this issue by decoupling the invoker of a command from the object that knows how to perform it.
In this article, we will explore how to integrate undo and redo functionality into a Java application using the Command Design Pattern. We will start by understanding the Command Design Pattern and then dive into the implementation of undo and redo functionality in a Java application.
Understanding the Command Design Pattern
The Command Design Pattern is a behavioral design pattern that encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. It promotes loose coupling between the invoker and the receiver of a request, thus enabling the separation of concerns and facilitating a higher degree of flexibility in the codebase.
In the context of implementing undo and redo functionality, the Command Design Pattern allows us to encapsulate each user action (command) as an object, which can be stored, executed, and undone as required.
Implementing Undo and Redo Functionality in Java
Step 1: Define the Command Interface
// Command interface
public interface Command {
void execute();
void undo();
}
In the above code snippet, we have defined a Command
interface with execute()
and undo()
methods. Any operation that needs to support undo and redo functionality must implement this interface.
Step 2: Implement Concrete Command Classes
Now, let's consider a hypothetical scenario where we have a drawing application that allows users to draw shapes on a canvas. We'll create two concrete command classes: DrawCommand
and EraseCommand
.
// Concrete command - DrawCommand
public class DrawCommand implements Command {
private Shape shape; // Assume Shape class exists
public DrawCommand(Shape shape) {
this.shape = shape;
}
@Override
public void execute() {
shape.draw();
}
@Override
public void undo() {
shape.erase();
}
}
// Concrete command - EraseCommand
public class EraseCommand implements Command {
private Shape shape; // Assume Shape class exists
public EraseCommand(Shape shape) {
this.shape = shape;
}
@Override
public void execute() {
shape.erase();
}
@Override
public void undo() {
shape.draw();
}
}
In the DrawCommand
and EraseCommand
classes, we've implemented the execute()
and undo()
methods specific to drawing and erasing operations. This separation of concerns aligns with the principles of the Command Design Pattern.
Step 3: Implement the Invoker
// Invoker
public class Invoker {
private Command command;
public void setCommand(Command command) {
this.command = command;
}
public void executeCommand() {
command.execute();
}
public void undoCommand() {
command.undo();
}
}
The Invoker
class is responsible for invoking the commands. It holds a reference to a Command
object and provides methods to execute and undo the command.
Step 4: Client Usage
Now, let's see how the client code would utilize these classes to achieve undo and redo functionality.
public class Client {
public static void main(String[] args) {
// Sample usage
Shape circle = new Circle(); // Assume Circle class exists
Command drawCommand = new DrawCommand(circle);
Command eraseCommand = new EraseCommand(circle);
Invoker invoker = new Invoker();
// User action - draw
invoker.setCommand(drawCommand);
invoker.executeCommand();
// User action - erase
invoker.setCommand(eraseCommand);
invoker.executeCommand();
// Undo the last action (erase)
invoker.undoCommand();
}
}
In the Client
class, we've instantiated the necessary objects and performed a sequence of actions using the invoker. The ability to undo the last action is provided through the undoCommand()
method of the Invoker
class.
Incorporating the Memento Pattern for Efficient Undo/Redo Management
To make the undo and redo functionality more robust and efficient, the Memento Design Pattern can be integrated with the Command Design Pattern. The Memento pattern captures and externalizes an object's internal state without violating encapsulation, allowing the object to be restored to this state later.
By storing the state of the shape in the execute()
method and restoring it in the undo()
method, the Memento pattern enhances the undo and redo functionality in a way that provides a more comprehensive history of state changes.
Final Thoughts
In this article, we explored the implementation of undo and redo functionality in Java applications using the Command Design Pattern. By encapsulating user actions as objects and decoupling the invoker from the receiver, the Command Design Pattern allows for flexible and robust undo and redo management. Additionally, we discussed how integrating the Memento Design Pattern can further enhance the efficiency and granularity of undo and redo operations.
Implementing undo and redo functionality is crucial in user-centric applications, and the Command Design Pattern provides a powerful framework for achieving this in a clean and maintainable manner.
Integrating these design patterns not only offers a solution to the immediate problem but also enhances the overall design and extensibility of the codebase, making it an invaluable addition to the developer's toolkit.
By adopting the Command Design Pattern and considering the integration of the Memento Pattern, developers can ensure that their Java applications provide a seamless and intuitive user experience, complete with robust undo and redo functionality.
So, the next time you're faced with the challenge of implementing undo and redo functionality, remember the Command Design Pattern and its potential to revolutionize the way user interactions are managed in your Java applications.
For further reading on the Command Design Pattern and related design patterns in Java, check out this comprehensive guide from Baeldung.
That's all for now! Happy coding!