Optimizing Asynchronous SQL Execution with jOOQ
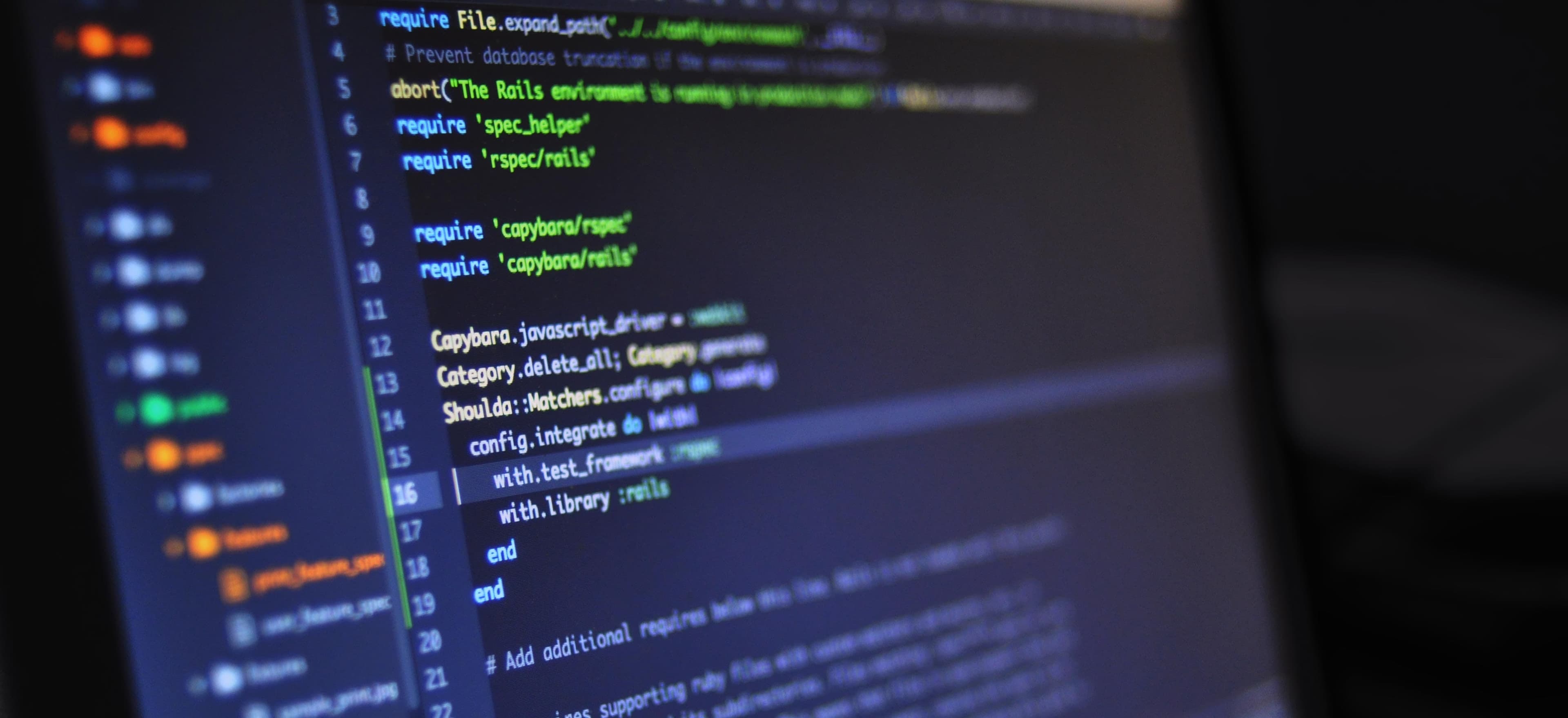
- Published on
Optimizing Asynchronous SQL Execution with jOOQ
In today's fast-paced, data-driven world, optimizing database operations is essential for the overall performance of an application. Asynchronous execution of SQL queries can significantly improve the responsiveness and scalability of an application by allowing it to continue processing other tasks while waiting for the database to respond. In Java, jOOQ provides a powerful toolkit for building type-safe SQL queries and interacting with databases. In this article, we will explore how to optimize asynchronous SQL execution using jOOQ.
Understanding Asynchronous Execution
Asynchronous execution allows a program to continue its operation while waiting for a potentially time-consuming process to complete. In the context of database operations, this means that a program can initiate a query and continue with other tasks, checking back later for the results. This is particularly valuable in scenarios where multiple queries need to be executed concurrently or when interfacing with slow or high-latency databases.
Leveraging CompletableFuture for Asynchronous Execution
In Java, the CompletableFuture
class provides a powerful tool for asynchronous programming. Leveraging CompletableFuture
with jOOQ allows for executing SQL queries asynchronously and handling the results when they are available. This can be especially beneficial in scenarios where multiple database operations need to be performed concurrently.
Let's consider an example where we want to asynchronously execute a simple SQL query using jOOQ:
import static org.jooq.impl.DSL.*;
// Using jOOQ to create a simple SQL query
String sql = select(field("id"), field("name"))
.from(table("users"))
.where(field("id").eq(1))
.getSQL();
// Creating a CompletableFuture for the asynchronous execution
CompletableFuture<Result<Record>> future = CompletableFuture.supplyAsync(() ->
DSL.using(dataSource, SQLDialect.POSTGRES).fetch(sql)
);
In the above example, CompletableFuture.supplyAsync
is used to asynchronously execute the SQL query. The fetch
method returns the results of the query wrapped in a Result
object.
Handling Asynchronous Results
Once the asynchronous SQL execution is initiated, we need to handle the results when they become available. CompletableFuture
provides methods like thenApply
, thenAccept
, and thenCompose
for processing the asynchronous results. For example:
// Handling the asynchronous result
future.thenAccept(result -> {
result.forEach(record -> {
int id = record.getValue("id", int.class);
String name = record.getValue("name", String.class);
System.out.println("User ID: " + id + ", Name: " + name);
});
});
In the above code, the thenAccept
method is used to process the Result
object when it becomes available. This allows us to iterate over the records and handle them as needed.
Optimizing Asynchronous Execution with jOOQ Configuration
jOOQ provides configuration options for optimizing asynchronous execution. For example, the ExecutorProvider
interface allows for customizing the executor used for asynchronous operations. By providing a custom executor, it is possible to control the threading model and resource usage of asynchronous SQL execution.
// Customizing the executor for asynchronous operations
public class CustomExecutorProvider implements ExecutorProvider {
@Override
public Executor executor(Configuration configuration) {
return Executors.newFixedThreadPool(10);
}
}
// Setting the custom executor provider in jOOQ configuration
Settings settings = new Settings().withExecutorProvider(new CustomExecutorProvider());
DSLContext dsl = DSL.using(dataSource, SQLDialect.POSTGRES, settings);
In the above example, a custom ExecutorProvider
is defined to provide a fixed thread pool for asynchronous operations. This allows for better control over the concurrency of asynchronous SQL execution.
Leveraging Reactive Streams for Asynchronous Database Interaction
Reactive Streams provide a way to handle asynchronous data sequences with non-blocking back pressure. jOOQ also offers support for integrating with Reactive Streams, allowing for efficient handling of asynchronous database interactions.
// Using jOOQ with Reactive Streams
DSL.using(dataSource, SQLDialect.POSTGRES)
.select(field("id"), field("name"))
.from(table("users"))
.fetchStream()
.forEach(record -> {
int id = record.getValue("id", int.class);
String name = record.getValue("name", String.class);
System.out.println("User ID: " + id + ", Name: " + name);
});
In the above example, fetchStream
is used to fetch the results as a Reactive Streams publisher. This allows for efficient handling of asynchronous database interactions with back pressure support.
A Final Look
Optimizing asynchronous SQL execution with jOOQ can greatly enhance the performance and scalability of Java applications. By leveraging CompletableFuture
, configuring custom executors, and integrating with Reactive Streams, developers can efficiently handle asynchronous database interactions. Understanding and implementing these techniques can lead to highly responsive and scalable database operations, ultimately improving the overall user experience.
In conclusion, jOOQ provides powerful capabilities for optimizing asynchronous SQL execution, and when used effectively, it can elevate the performance of database interactions in Java applications.
To learn more about jOOQ and its features for asynchronous execution, visit the official jOOQ website.
Remember, optimizing asynchronous SQL execution is just one aspect of building high-performance applications. Employing best practices, continual testing, and staying updated with the latest developments will contribute to the overall success of your projects.