Calculating Unique Digits in Java Numbers
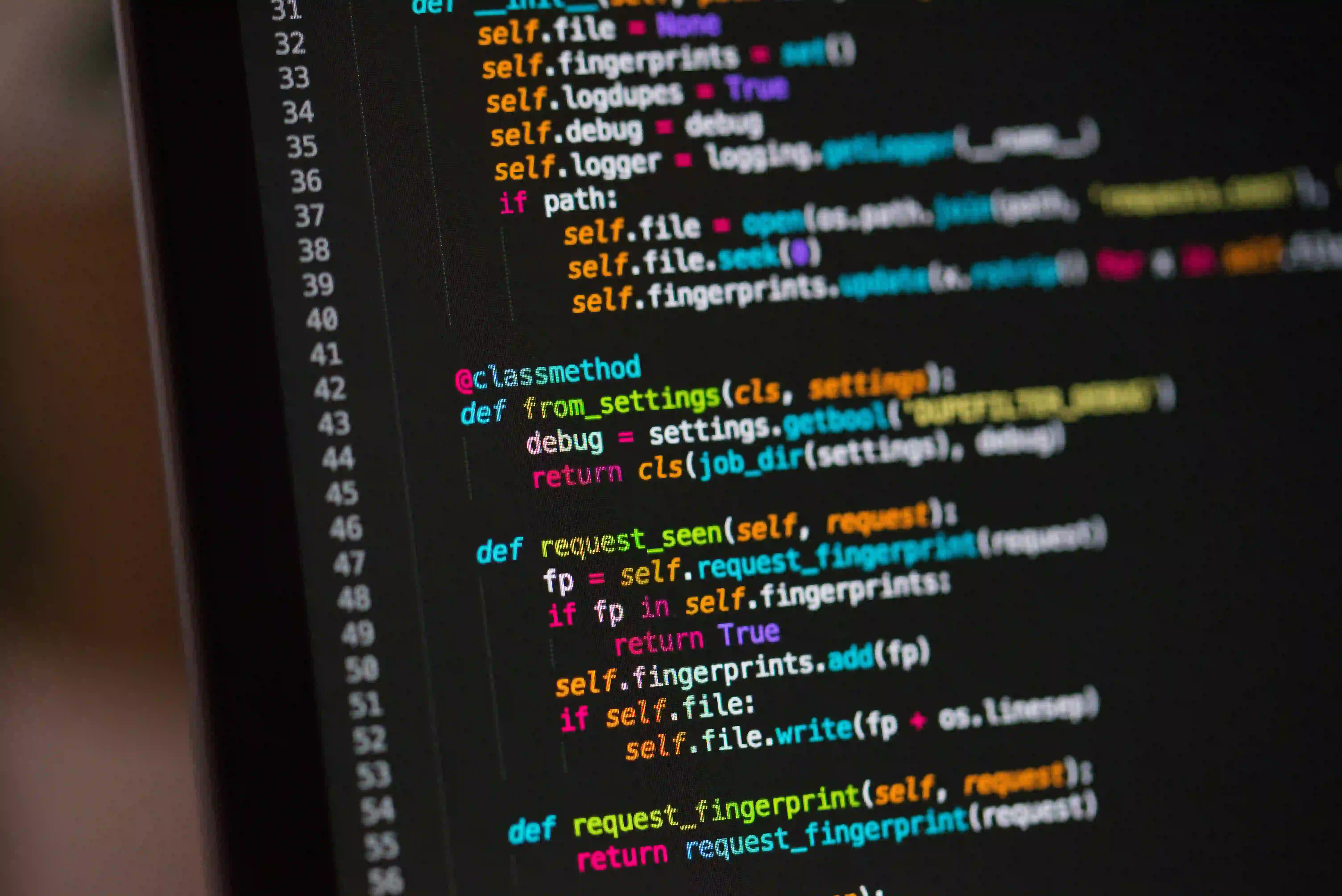
Calculating Unique Digits in Java Numbers
When working with numbers in Java, it's common to encounter tasks that involve extracting and analyzing the digits within them. One such task is determining the number of unique digits present in a given number. In this blog post, we'll explore how to approach this problem in Java, covering both the theory behind the solution and the practical implementation using code examples.
Understanding the Problem
Before diving into the implementation, let's take a moment to understand the problem at hand. When we talk about unique digits in a number, we are referring to the distinct individual digits that make up the number. For example, the number 12345 has five unique digits (1, 2, 3, 4, and 5).
The key to solving this problem lies in iterating through the digits of the number and keeping track of which digits have been encountered. We can use a data structure to store the encountered digits and then count the total number of unique digits at the end of the iteration.
Strategy for Solution
To solve this problem, we can use a Set
to store the unique digits we encounter while iterating through the number. A Set
is a collection that does not allow duplicate elements, making it an ideal choice for this task. We'll iterate through the number by extracting individual digits and adding them to the Set
, and finally, we'll return the size of the Set
as the count of unique digits.
Implementation in Java
Let's dive into the implementation of the solution in Java. We'll create a method that takes an integer as input and returns the count of unique digits in the number.
import java.util.HashSet;
import java.util.Set;
public class UniqueDigitsCalculator {
public static int countUniqueDigits(int number) {
Set<Integer> uniqueDigits = new HashSet<>();
while (number > 0) {
int digit = number % 10;
uniqueDigits.add(digit);
number /= 10;
}
return uniqueDigits.size();
}
}
In the countUniqueDigits
method, we initialize a HashSet
called uniqueDigits
to store the unique digits. We then iterate through the number by repeatedly taking the remainder when divided by 10, which gives us the rightmost digit. We add this digit to the uniqueDigits
set and divide the number by 10 to move to the next digit. Finally, we return the size of the uniqueDigits
set, which gives us the count of unique digits in the number.
Testing the Implementation
Now that we have the implementation ready, let's test it with some sample inputs to verify its correctness.
public class Main {
public static void main(String[] args) {
int number1 = 12345;
int number2 = 987654321;
System.out.println("Number of unique digits in " + number1 + ": " + UniqueDigitsCalculator.countUniqueDigits(number1));
System.out.println("Number of unique digits in " + number2 + ": " + UniqueDigitsCalculator.countUniqueDigits(number2));
}
}
When we run the Main
class, we should see the following output:
Number of unique digits in 12345: 5
Number of unique digits in 987654321: 9
The output confirms that our countUniqueDigits
method is working as expected, correctly counting the unique digits in the given numbers.
Lessons Learned
In this blog post, we explored the problem of calculating unique digits in Java numbers and implemented a solution using a Set
to store the encountered digits. By breaking down the problem and understanding the underlying concepts, we were able to devise an efficient and elegant solution. This approach not only provides the count of unique digits but also demonstrates the power of using appropriate data structures to solve such challenges in Java programming.
To further enhance your understanding, you can delve into more advanced topics such as digit manipulation and number theory in Java. Understanding these concepts will not only enrich your programming skills but also empower you to tackle a wider range of numerical challenges in Java.
We hope this blog post has been informative and valuable in expanding your knowledge of working with numbers in Java. Happy coding!