Impact of Servlet 3.0 on Java Web Development
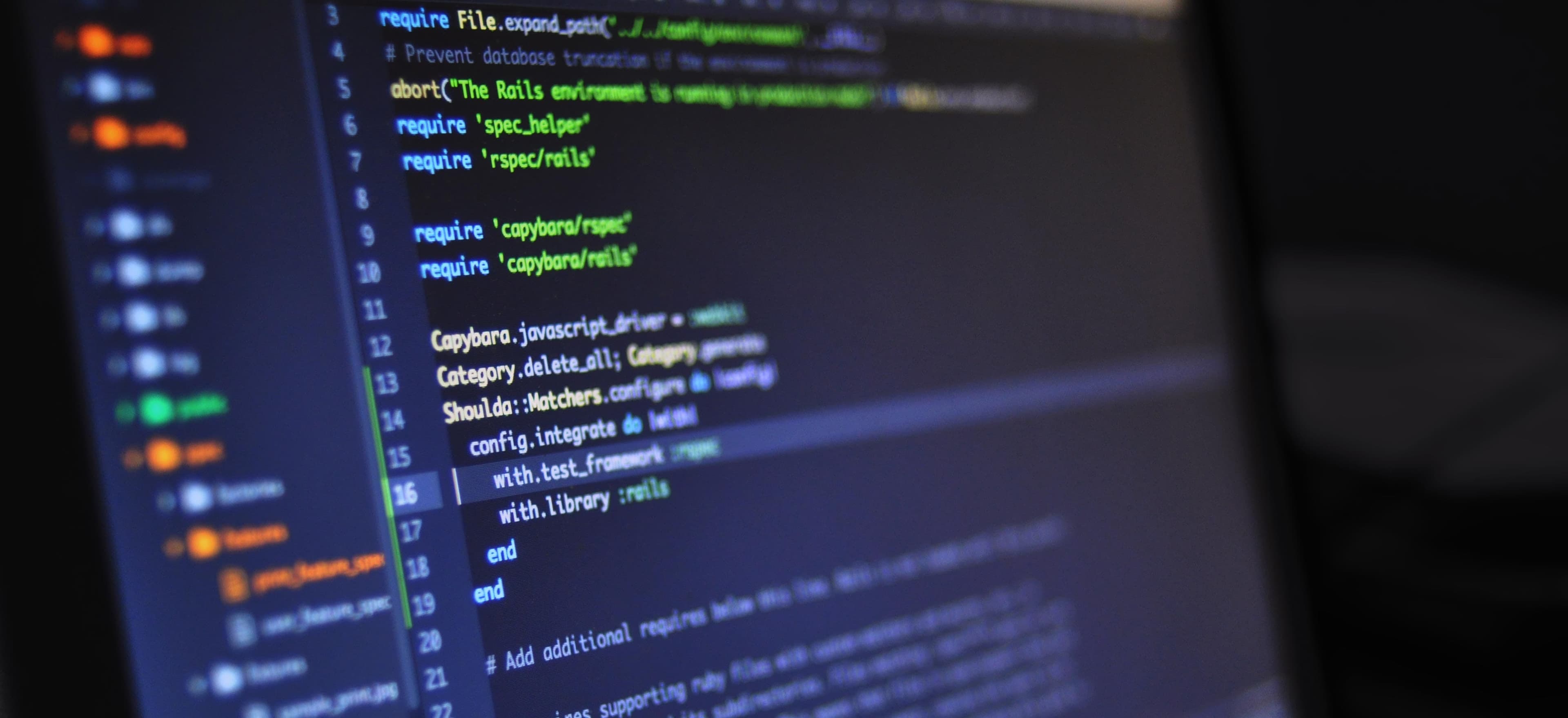
- Published on
Impact of Servlet 3.0 on Java Web Development
Servlet 3.0, introduced as part of Java EE 6, brought several significant enhancements to the Java web development landscape. These enhancements streamlined the development process, improved performance, and provided better support for modern web development practices. In this article, we will delve into the impact of Servlet 3.0 on Java web development and explore how it has shaped the way Java web applications are built.
Asynchronous Processing
One of the key features introduced in Servlet 3.0 is support for asynchronous processing. Traditionally, servlets were designed to handle requests synchronously, which could lead to performance bottlenecks, especially in applications with high concurrency. With Servlet 3.0, developers can now handle requests asynchronously, allowing the server to process other requests while waiting for potentially time-consuming operations to complete. This improves the overall responsiveness and scalability of Java web applications.
@WebServlet("/asyncServlet", asyncSupported = true)
public class AsyncServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
AsyncContext asyncContext = request.startAsync();
// Perform asynchronous processing
}
}
The @WebServlet
annotation with the asyncSupported
attribute set to true
indicates that the servlet supports asynchronous processing. Inside the doGet
method, the startAsync
method is called to initiate asynchronous processing.
Dynamic Servlet Registration
Prior to Servlet 3.0, servlets had to be declared in the deployment descriptor (web.xml) or programmatically registered using the Servlet API. Servlet 3.0 introduced a more flexible approach through annotations, allowing servlets to be dynamically registered without the need for explicit configuration in the deployment descriptor.
@WebServlet("/dynamicServlet")
public class DynamicServlet extends HttpServlet {
// Servlet implementation
}
The @WebServlet
annotation eliminates the need to configure servlet mappings in the web.xml file, making the servlet registration process more streamlined and enhancing the overall developer experience.
Web-Fragment Support
Servlet 3.0 introduced the concept of web fragments, which allows libraries and frameworks to define their own deployment descriptors without conflicting with the main web.xml file. This modular approach facilitates easier integration of third-party libraries and frameworks into Java web applications, promoting greater reusability and maintainability.
File Upload Enhancements
Handling file uploads in earlier versions of Servlet required manual parsing of the request body. Servlet 3.0 simplified this process by providing built-in support for handling multipart requests, making it easier for developers to process file uploads without resorting to third-party libraries or custom parsing logic.
@MultipartConfig
@WebServlet("/fileUploadServlet")
public class FileUploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) {
Part filePart = request.getPart("file");
// Process the uploaded file
}
}
The @MultipartConfig
annotation configures the servlet to handle multipart requests, while the request.getPart
method retrieves the uploaded file for processing.
Summary
In conclusion, Servlet 3.0 has significantly influenced Java web development by introducing asynchronous processing, dynamic servlet registration, web-fragment support, and file upload enhancements. These features have modernized the way Java web applications are built, promoting better performance, scalability, and developer productivity. Embracing Servlet 3.0 and leveraging its capabilities is essential for staying competitive in the ever-evolving landscape of web development.
To delve deeper into the topic, check out the official documentation of Java EE for detailed insights.
Stay tuned for more insightful content on Java development!