Managing File Changes with Akka Actors
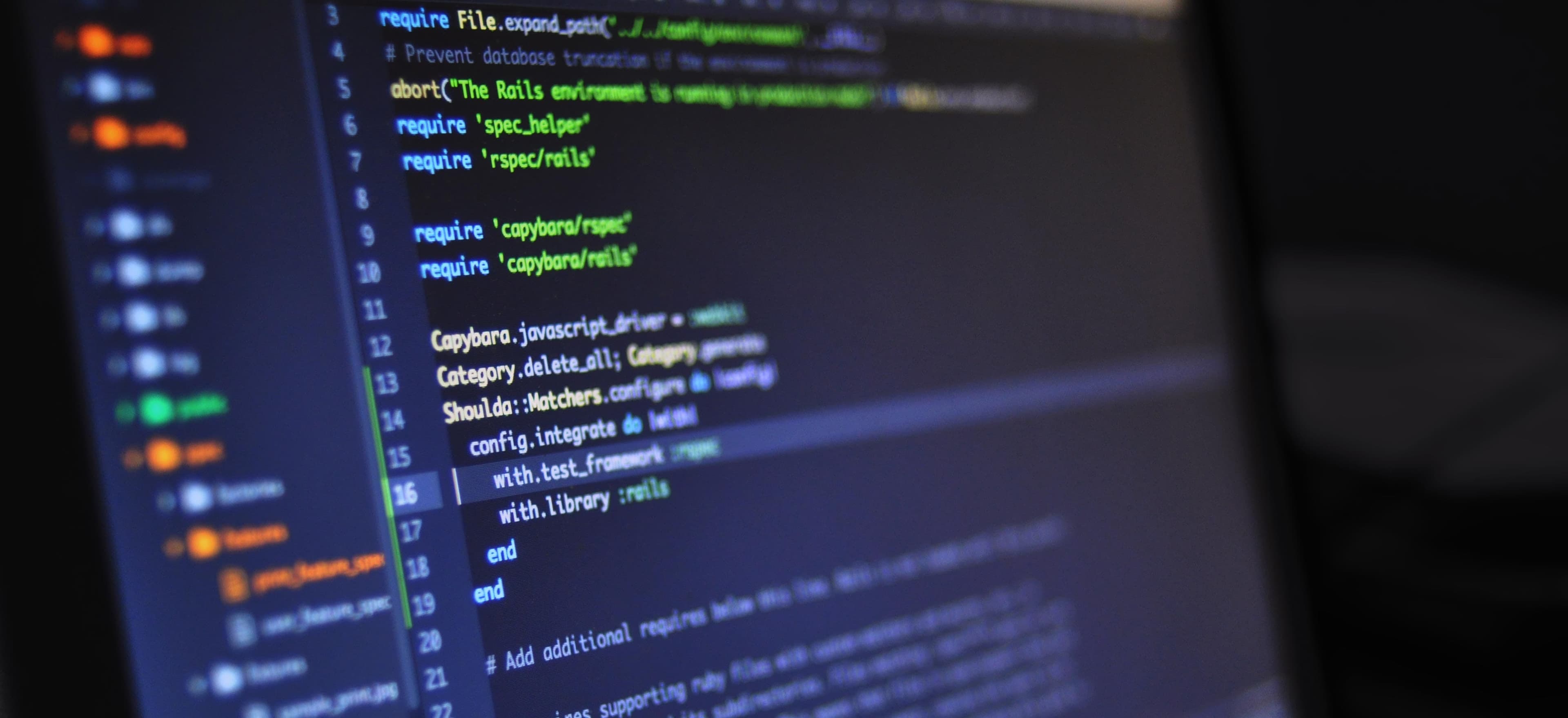
- Published on
Managing File Changes with Akka Actors
In this blog post, we will explore how to use Akka Actors in Java to manage file changes. Akka is a powerful toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications. By leveraging Akka Actors, we can create a responsive and fault-tolerant system for handling file changes.
Why Akka Actors?
Akka Actors provide a powerful model for building concurrent and distributed applications. The actor model encapsulates state and behavior, allowing for effective parallelism and isolation of mutable state. By leveraging actors, we can build scalable and fault-tolerant systems that are well-suited for managing file changes.
Setting Up the Akka Environment
Before diving into the implementation, let’s ensure Akka is set up in our Java environment. You can add the Akka dependency to your Maven project by including the following in your pom.xml
:
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-actor_2.12</artifactId>
<version>2.6.16</version>
</dependency>
For non-Maven projects, you can download the Akka library from the official website.
With Akka added to the project, we are ready to start implementing the file change management system using actors.
Creating the FileChangeActor
The first step is to create an Akka Actor responsible for monitoring file changes. We'll name it FileChangeActor
. This actor will watch a specific file and notify other actors about any changes.
public class FileChangeActor extends AbstractActor {
private String filePath;
private File file;
public FileChangeActor(String filePath) {
this.filePath = filePath;
this.file = new File(filePath);
if (!file.exists()) {
throw new IllegalArgumentException("File does not exist: " + filePath);
}
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(CheckFile.class, this::checkFile)
.build();
}
private void checkFile(CheckFile checkFile) {
if (file.lastModified() > checkFile.getLastModifiedTime()) {
getSender().tell(new FileChanged(filePath), getSelf());
}
}
// Messages
public static class CheckFile {
private long lastModifiedTime;
public CheckFile(long lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
public long getLastModifiedTime() {
return lastModifiedTime;
}
}
public static class FileChanged {
private String filePath;
public FileChanged(String filePath) {
this.filePath = filePath;
}
public String getFilePath() {
return filePath;
}
}
}
In the FileChangeActor
, we define two message classes: CheckFile
and FileChanged
. The CheckFile
message is used to request a file check, providing the last modified time for comparison. The FileChanged
message is sent to notify other actors about a file change.
Using the FileChangeActor
Once the FileChangeActor
is created, we can use it within our system to monitor file changes. The following code snippet demonstrates how to create an instance of FileChangeActor
and send a CheckFile
message to monitor the file for changes.
import akka.actor.ActorRef;
import akka.actor.ActorSystem;
import akka.actor.Props;
import java.time.Duration;
public class FileChangeApp {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("fileChangeSystem");
ActorRef fileChangeActor = system.actorOf(Props.create(FileChangeActor.class, "path/to/file.txt"), "fileChangeActor");
// Periodically check for file changes
system.scheduler().schedule(
Duration.ofSeconds(1),
Duration.ofSeconds(5),
fileChangeActor,
new FileChangeActor.CheckFile(System.currentTimeMillis()),
system.dispatcher(),
ActorRef.noSender()
);
}
}
In this example, we create an instance of FileChangeActor
and schedule periodic file checks using system.scheduler().schedule
. This demonstrates how Akka Actors can be used to asynchronously monitor file changes in the background.
Handling File Change Notifications
Now that we have a mechanism to monitor file changes, we need to handle the file change notifications. We can create another actor, FileChangeHandlerActor
, to process the file change notifications and take appropriate actions.
public class FileChangeHandlerActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(FileChangeActor.FileChanged.class, this::handleFileChanged)
.build();
}
private void handleFileChanged(FileChangeActor.FileChanged fileChanged) {
// Perform actions upon file change
System.out.println("File changed: " + fileChanged.getFilePath());
}
}
In this FileChangeHandlerActor
, we define the behavior to handle FileChanged
messages. Upon receiving a file change notification, the actor can perform specific actions as needed.
Wiring Actors Together
To complete the system, we need to wire the FileChangeActor
and FileChangeHandlerActor
together. We can do this by using an ActorRef
to send file change notifications from FileChangeActor
to FileChangeHandlerActor
.
public class FileChangeApp {
public static void main(String[] args) {
// Create ActorSystem and actors
ActorRef fileChangeHandlerActor = system.actorOf(Props.create(FileChangeHandlerActor.class), "fileChangeHandlerActor");
ActorRef fileChangeActor = system.actorOf(Props.create(FileChangeActor.class, "path/to/file.txt"), "fileChangeActor");
// Subscribe FileChangeHandlerActor to receive notifications from FileChangeActor
fileChangeActor.tell(new FileChangeActor.Subscribe(fileChangeHandlerActor), ActorRef.noSender());
// Schedule file checks as before
}
}
Here, we create an instance of FileChangeHandlerActor
and subscribe it to receive notifications from FileChangeActor
. This enables seamless communication between the actors, allowing the system to appropriately handle file change events.
The Closing Argument
In this blog post, we explored how to use Akka Actors in Java to manage file changes. By leveraging the actor model, we created a robust system capable of monitoring file changes and responding accordingly. Akka Actors provide an elegant solution for building concurrent, responsive, and fault-tolerant file change management systems.
By implementing this approach, you can create sophisticated file monitoring systems that are well-suited for a variety of use cases including log file tracking, data ingestion, and real-time processing of file changes.
With the power of Akka Actors at your disposal, managing file changes in Java becomes a seamless and efficient process, opening up a world of possibilities for building responsive and resilient file-based applications.
Checkout our other articles