Understanding and Implementing Clojure Macro Basics
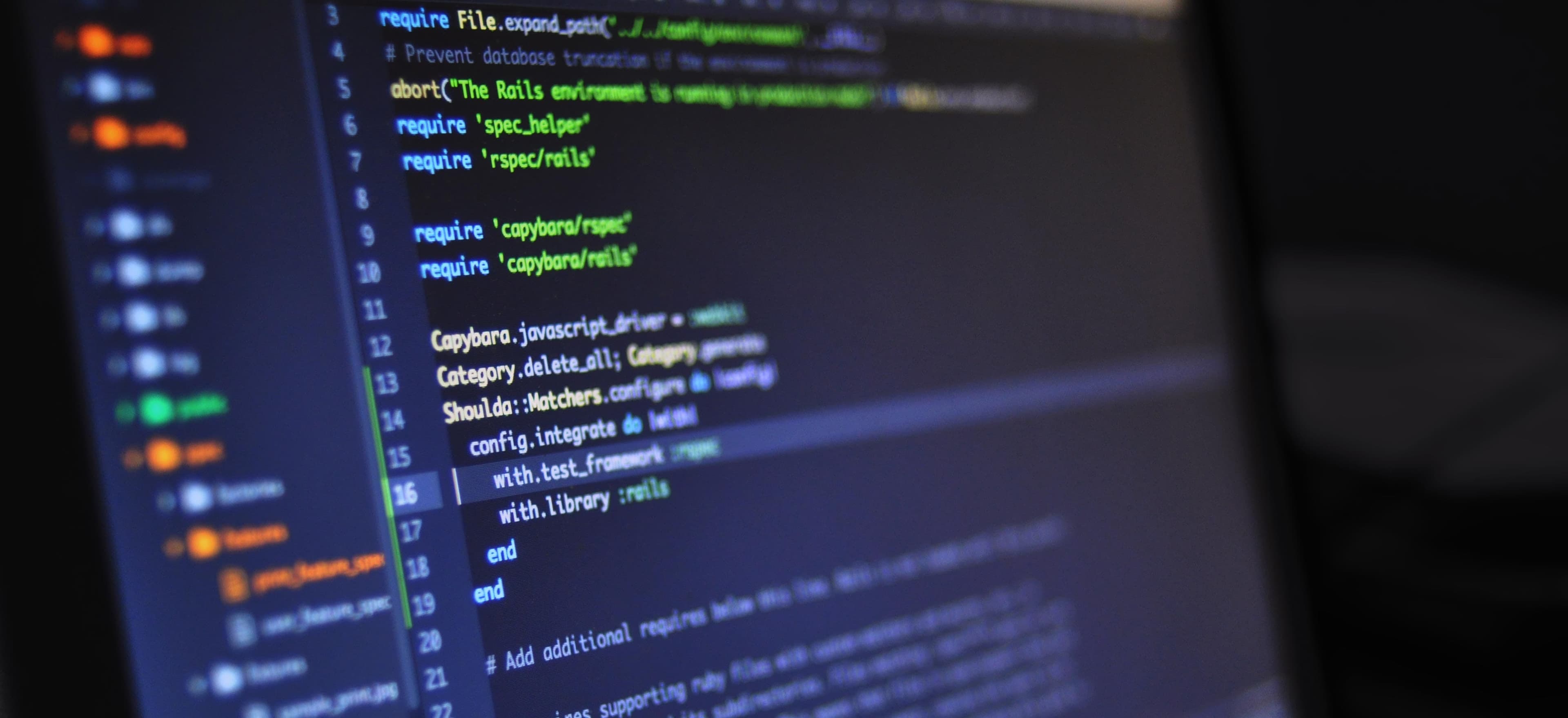
- Published on
A Brief Introduction to Clojure Macros
Clojure, a dynamic, general-purpose programming language, is known for its powerful macro system. In this post, we'll delve into the basics of Clojure macros, understand their significance, and walk through practical examples to implement them effectively.
What are Clojure Macros?
In Clojure, macros are a mechanism for transforming code at compile time. They allow developers to define new syntax, abstract patterns, and automate repetitive tasks. Macros are an essential tool for creating DSLs (Domain Specific Languages) and improving code expressiveness.
Why Use Clojure Macros?
Macros enable developers to extend the language by creating custom abstractions. This empowers them to write more concise, readable, and maintainable code. By using macros, repetitive code patterns can be encapsulated, ensuring consistency and reducing cognitive load.
Anatomy of a Clojure Macro
Let's explore the fundamental structure of a Clojure macro before we delve into its implementation.
(defmacro custom-if
[condition then-expr else-expr]
`(if ~condition ~then-expr ~else-expr))
defmacro
is the macro definition form in Clojure.custom-if
is the name of the macro we are defining.[condition then-expr else-expr]
are the arguments the macro takes.- The backtick symbol ``` is used for syntax-quoting.
- The tilde symbol
~
unquotes the contained expression, allowing the evaluation of the variables.
Implementing a Custom Macro
Let's create a simple macro named not-empty
that checks if a collection is not empty. This macro will mimic the behavior of Clojure's not-empty
function but operate at compile time.
(defmacro not-empty
[coll]
`(if (seq ~coll) ~coll nil))
In this example, the not-empty
macro checks if the collection coll
is not empty. If it's not empty, it returns the collection; otherwise, it returns nil
. This macro simplifies the code by eliminating the need to use the seq
function explicitly.
Now, let's see the not-empty
macro in action:
(defn process-data [data]
(let [valid-data (not-empty data)]
(if valid-data
(process valid-data)
(log "Invalid data"))))
By using the not-empty
macro, the code becomes more expressive and readable. The conditional check and the seq
function invocation are abstracted, leading to cleaner code.
Practical Example: Threading Macro
Clojure's threading macro is a widely-used macro that allows for a more readable and expressive way of chaining functions. Let's examine a simple use case of the threading macro.
(->> (range 10)
(map inc)
(filter even?)
(reduce +))
In this example, the threading macro ->>
threads the result of each expression as the last argument of the next expression. This results in a concise and clear representation of a sequence of operations.
Best Practices and Considerations
- Use Macros Sparingly: While macros are powerful, they should be used judiciously. Overuse of macros can lead to code that is difficult to understand and maintain.
- Ensure Hygiene: When writing macros, ensure that the introduced symbols do not inadvertently capture or interfere with existing symbols in the code.
- Test Thoroughly: Just like regular code, macros should be thoroughly tested to ensure they behave as expected and handle edge cases correctly.
A Final Look
Clojure macros are a powerful feature that enables developers to extend the language, create abstractions, and enhance code expressiveness. By understanding the basics of Clojure macros and employing best practices, developers can leverage macros to write more concise and maintainable code.
Further exploration of Clojure macros can lead to the creation of expressive DSLs and innovative abstractions that facilitate elegant and efficient solutions to complex problems.
In conclusion, mastering Clojure macros is an indispensable skill for any Clojure developer looking to elevate their code to the next level.
To deepen your understanding of Clojure macros, you can explore the official Clojure macro documentation and the book "Clojure Programming" by Chas Emerick, Brian Carper, and Christophe Grand.
Start incorporating Clojure macros into your code today and unleash the full potential of this remarkable language feature.
Checkout our other articles