Handling Object Composition in Java
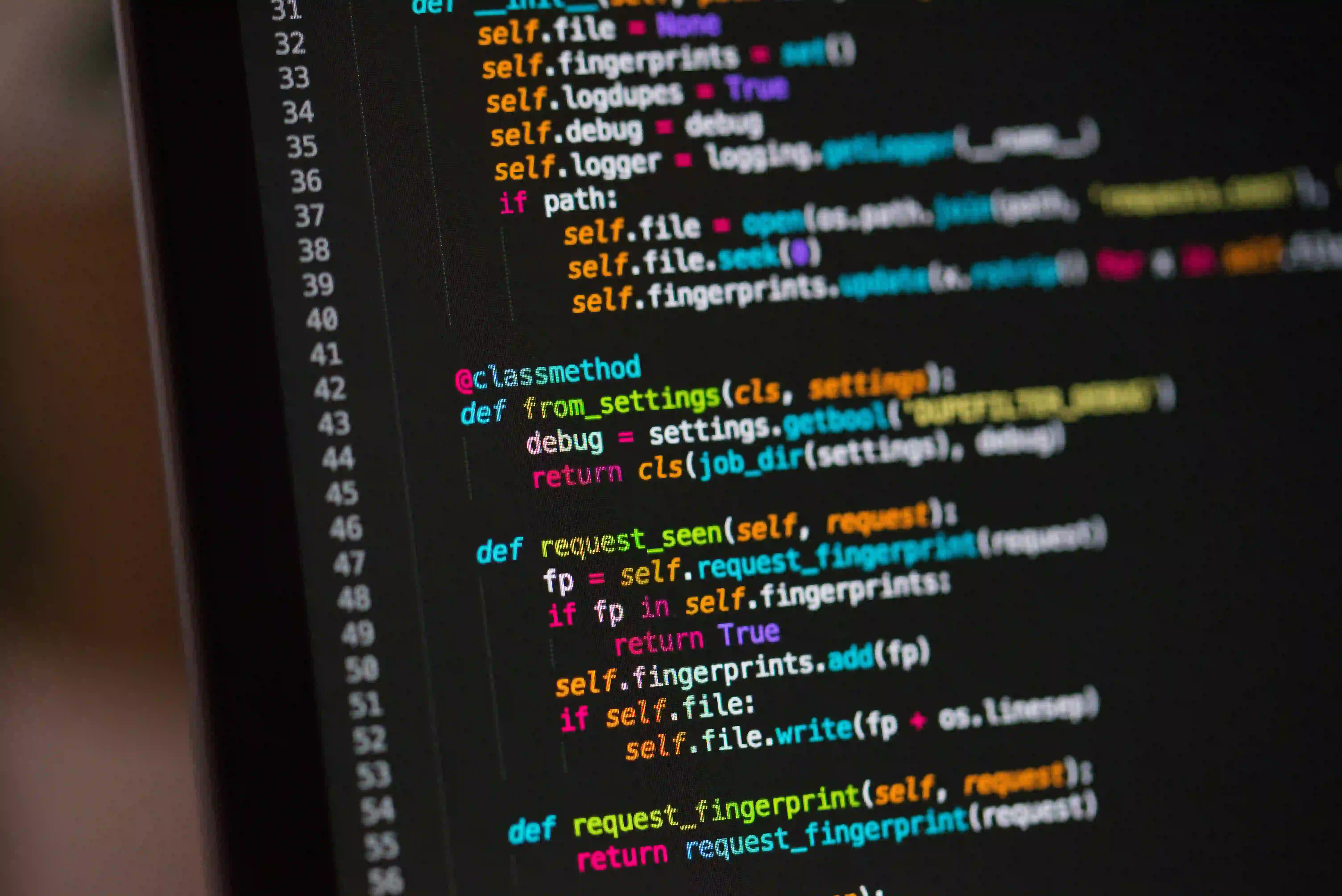
Object composition is a fundamental concept in object-oriented programming. It involves creating complex objects by combining simpler objects, allowing for a more modular and reusable design. In Java, object composition is achieved through the use of classes and interfaces to create relationships between objects. This article will explore the principles of object composition in Java, covering the use of interfaces, inheritance, and composition to create flexible and maintainable code.
What is Object Composition?
Object composition refers to the practice of building complex object structures by combining simpler objects. This approach emphasizes the creation of objects that are composed of other objects, rather than relying solely on inheritance. By combining objects, we can create flexible, modular, and loosely coupled designs that are easier to maintain and extend.
Interfaces for Composable Behavior
In Java, interfaces play a crucial role in defining composable behavior. An interface defines a contract for a set of behaviors without specifying the implementation. This allows multiple classes to implement the same interface, providing different implementations for the defined behaviors.
Let's consider an example where we have a Shape
interface that defines the behavior for calculating the area of a shape:
public interface Shape {
double calculateArea();
}
By defining the Shape
interface, we can have different classes such as Circle
and Rectangle
implement this interface and provide their own implementations for calculating the area. This allows us to compose different shapes without being concerned about their specific implementations.
Why use interfaces for object composition?
- Interfaces allow for flexibility: By programming to an interface, rather than a concrete implementation, we can easily switch the behavior at runtime by providing different implementations of the interface.
- Interfaces promote code reusability: Classes can implement multiple interfaces, enabling them to exhibit different behaviors in different contexts.
Inheritance vs. Composition
In the context of object composition, it's important to understand the distinction between inheritance and composition. Inheritance is the mechanism by which a new class is created, inheriting the properties and behaviors of an existing class. Composition, on the other hand, involves constructing a class using references to other classes to make it modular and easily maintainable.
Inheritance Example in Java
public class Animal {
public void eat() {
System.out.println("Animal is eating");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("Dog is barking");
}
}
In the above example, the Dog
class inherits the eat()
method from the Animal
class. While inheritance provides code reuse, it can lead to rigid class hierarchies and tight coupling between classes.
Composition Example in Java
public class Engine {
public void start() {
System.out.println("Engine started");
}
}
public class Car {
private Engine engine;
public Car() {
this.engine = new Engine();
}
public void startCar() {
engine.start();
}
}
In this example, the Car
class is composed of an Engine
instance. This allows for greater flexibility as the Car
class can change its engine at runtime without altering its structure.
Why prefer composition over inheritance for object composition?
- Flexibility: Composition allows for greater flexibility by providing the ability to change behavior at runtime.
- Loose coupling: Using composition results in less tightly coupled code, making it easier to modify and maintain.
Delegation and Forwarding
Delegation is a powerful technique used in object composition to achieve code reuse. It involves one object passing on a method call to another object to perform the actual work. In Java, delegation is often implemented using interfaces and composition.
Let's consider a scenario where we have a Printer
interface for printing documents, and we want to delegate the actual printing functionality to different printer implementations.
public interface Printer {
void print(String document);
}
public class LaserPrinter implements Printer {
public void print(String document) {
// Logic to print using a laser printer
}
}
public class InkjetPrinter implements Printer {
public void print(String document) {
// Logic to print using an inkjet printer
}
}
public class PrintController {
private Printer printer;
public void setPrinter(Printer printer) {
this.printer = printer;
}
public void printDocument(String document) {
printer.print(document);
}
}
In the above example, the PrintController
class delegates the printing functionality to different printer implementations through the Printer
interface. This allows the PrintController
to remain agnostic to the specific type of printer being used.
Why use delegation for object composition?
- Modularity: Delegation promotes modularity by allowing each component to focus on a specific task.
- Code Reuse: Delegating common functionality to shared components promotes code reuse and maintainability.
Key Takeaways
Object composition plays a crucial role in creating flexible, modular, and maintainable code in Java. By using interfaces, composition, delegation, and forwarding, we can design software systems that are easier to extend, modify, and test. Embracing object composition empowers developers to build robust and adaptable systems that can evolve with changing requirements.
In conclusion, object composition is not just a technique but a fundamental principle of building software systems that are resilient to change and conducive to growth.
In this post, we've explored the principles of object composition in Java and provided examples to illustrate its application. By leveraging object composition, developers can create code that is better organized, more flexible, and easier to maintain.
By understanding the benefits of object composition and applying these principles to your Java projects, you can build software systems that are scalable, adaptable, and robust.
The references provided below offer further insights into object composition and its application in Java.
References:
- Oracle Java Tutorials: Interfaces
- Baeldung Blog: Inheritance in Java
- Refactoring Guru: Delegation Pattern
- Effective Java, 3rd Edition by Joshua Bloch, Publisher: Addison-Wesley Professional.
Remember, mastering object composition is a key step towards becoming a proficient Java developer. Happy coding!