Mastering Test-Driven Development for Efficient Code
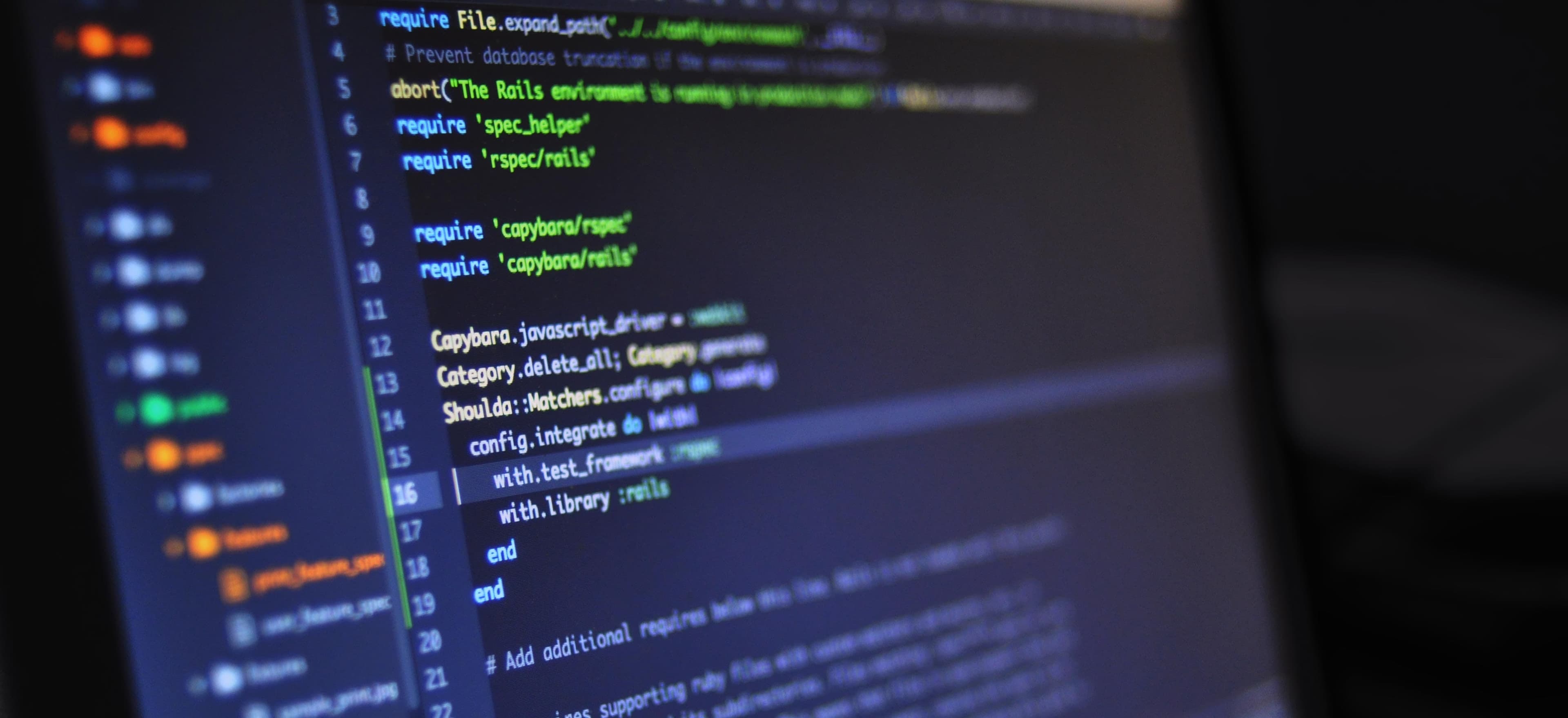
- Published on
Mastering Test-Driven Development for Efficient Code
Test-Driven Development (TDD) is a software development process that focuses on short development cycles. It relies on the repetition of a very short development cycle: first, the developer writes an (initially failing) automated test case that defines a desired improvement or new function, then produces the minimum amount of code to pass that test, and finally refactors the new code to acceptable standards. TDD can lead to efficient and robust code when utilized correctly.
In this article, we will explore the concept of Test-Driven Development in Java and how it can lead to more efficient code.
The Basics of Test-Driven Development
At its core, Test-Driven Development revolves around the mantra of "Red-Green-Refactor." This means writing a failing test first (Red), making the test pass by writing the minimum amount of code (Green), and then refactoring the code to improve its structure while ensuring the test still passes (Refactor).
The Advantages of Test-Driven Development
-
Improved Code Quality: TDD leads to well-tested and reliable code since tests are written before the code itself. This ensures that the codebase has a comprehensive test suite, making it easier to catch and fix bugs.
-
Better Design and Architecture: TDD encourages developers to focus on writing clean, modular, and maintainable code. The need to write testable code often leads to the creation of loosely coupled modules and clear interfaces.
-
Easier Maintenance: With a solid suite of tests, making changes to the codebase becomes less risky. The tests act as a safety net, allowing developers to make modifications confidently while ensuring previous functionality is not compromised.
Getting Started with Test-Driven Development in Java
In Java, JUnit is a popular choice for writing and running tests. It provides annotations to identify test methods and supports assertions for verifying expected results. Let's take a look at a basic example of TDD in Java using JUnit.
Setting Up the Project
First, make sure to include the JUnit dependency in your pom.xml
if you are using Maven:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
For Gradle, include the following in your build.gradle
:
testImplementation 'junit:junit:4.12'
Writing a Simple Test
Suppose we want to implement a basic Calculator
class with an add
method. We will start by writing a test for this method:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
Implementing the Minimum Code to Pass the Test
Now, we'll create the Calculator
class to make the test pass:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Refactoring
At this stage, our test is passing, and the code satisfies the minimal requirement. We can now refactor the add
method with confidence, knowing that as long as the test continues to pass, we have not introduced any functional changes.
Best Practices for Test-Driven Development
To truly master Test-Driven Development, it's essential to follow certain best practices that can enhance the effectiveness of the process.
Write Focused and Understandable Tests
Tests should be clear, concise, and focused on a specific behavior. Well-named test methods can serve as living documentation and improve the overall understandability of the codebase.
Embrace the Red-Green-Refactor Cycle
Following the strict sequence of writing a failing test, making it pass, and then refactoring is crucial. This cycle ensures that the code remains clean and efficient as it evolves.
Avoid Writing Unnecessary Code
TDD encourages the creation of just enough code to fulfill the requirements. Avoid writing speculative code that may not be immediately necessary, as it can lead to unnecessary complexity.
Continuously Refactor
Refactoring is an ongoing process that should be constantly integrated into the development cycle. This helps keep the codebase clean, maintainable, and adaptable to change.
Utilize Mocking and Stubbing
In complex systems, utilizing mocking frameworks like Mockito can isolate the code under test and provide controlled environments for more reliable and focused testing.
My Closing Thoughts on the Matter
Test-Driven Development is a powerful approach for writing efficient and reliable code. By following the principles of TDD, developers can create well-tested, maintainable codebases that are less prone to bugs and easier to extend. Although mastering TDD may require time and practice, the benefits it provides make it a valuable skill for any Java developer.
In summary, embracing TDD in Java can lead to not just efficient code, but also a more structured, maintainable, and robust codebase. By obeying the core principles and best practices of TDD, developers can elevate their coding standards and deliver higher quality software products.
Start your TDD journey today and witness the significant impact it can have on your code's efficiency and reliability. Happy coding!