Using Spark Next Rest Framework in Java
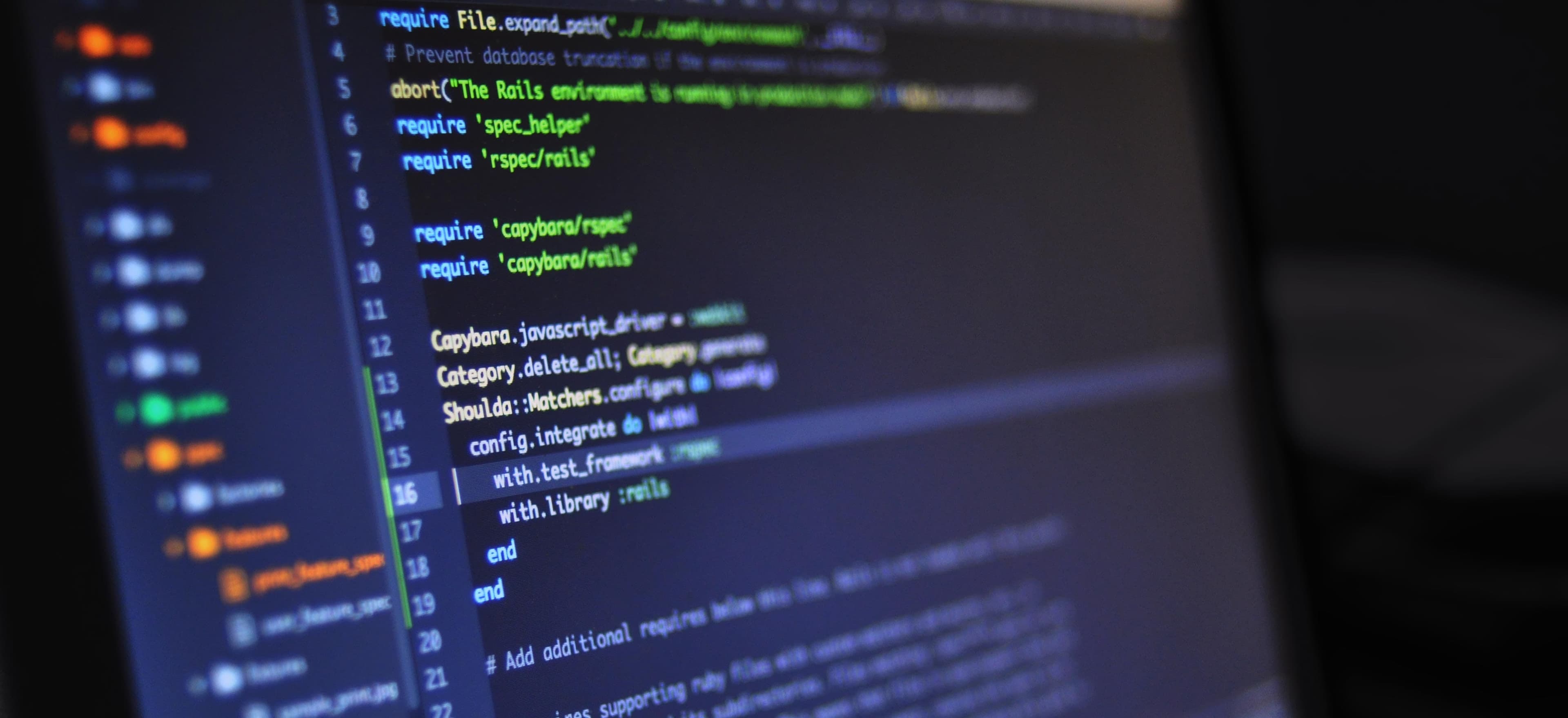
- Published on
Building Restful APIs with Spark Java Framework
In the world of web development, creating a robust and efficient backend for your application is crucial. When it comes to building Restful APIs in Java, developers often seek out lightweight and fast frameworks that allow them to quickly set up endpoints and handle HTTP requests. One such framework that has gained popularity in recent years is Spark Java.
What is Spark Java?
Spark is a micro framework for creating web applications in Java 8 with minimal effort. It is known for its simplicity and ease of use, making it an ideal choice for building Restful APIs. Spark is not to be confused with Apache Spark, which is a big data processing framework. Instead, Spark Java is a lightweight web framework that provides a simple and expressive syntax.
Setting up a Spark Project
To get started with Spark, you need to set up a Java project using your preferred build tool, such as Maven or Gradle. Once your project is set up, you can add the Spark dependency to your pom.xml
file if you're using Maven, or to your build.gradle
file if you're using Gradle.
Maven Dependency
<dependencies>
<dependency>
<groupId>com.sparkjava</groupId>
<artifactId>spark-core</artifactId>
<version>2.9.3</version>
</dependency>
</dependencies>
Gradle Dependency
dependencies {
implementation 'com.sparkjava:spark-core:2.9.3'
}
After adding the dependency, you can start writing your Restful APIs using Spark's expressive syntax.
Creating a Simple Restful API
Let's create a simple Restful API using Spark to understand how easy it is to set up endpoints and handle HTTP requests.
Hello World Example
import static spark.Spark.*;
public class HelloWorld {
public static void main(String[] args) {
get("/hello", (req, res) -> "Hello World");
}
}
In this example, we've created a simple endpoint that responds with "Hello World" when a GET request is made to /hello
.
Why it's awesome: Spark's expressive syntax allows you to define routes and handle requests with minimal code. The get("/hello", (req, res) -> "Hello World")
line demonstrates the simplicity and elegance of defining a GET endpoint that returns a static string.
Handling Request Parameters
Restful APIs often need to handle request parameters, such as query parameters or path parameters. Let's look at how Spark simplifies this process.
Path Parameter Example
import static spark.Spark.*;
public class PathParamExample {
public static void main(String[] args) {
get("/hello/:name", (req, res) -> "Hello " + req.params(":name"));
}
}
In this example, we've defined a GET endpoint with a path parameter :name
. When a request is made to /hello/Alice
, the API responds with "Hello Alice".
Why it's awesome: Spark handles path parameters effortlessly with its req.params(":name")
syntax, allowing you to access the values directly without cumbersome boilerplate code.
Error Handling with Spark
Error handling is an essential aspect of building robust Restful APIs. Spark provides simple yet powerful ways to handle errors and define custom error responses.
Handling 404 Not Found
import static spark.Spark.*;
public class NotFoundExample {
public static void main(String[] args) {
notFound((req, res) -> {
res.type("application/json");
return "{\"message\":\"Custom 404 Not Found\"}";
});
}
}
In this example, we've defined a custom 404 Not Found handler that responds with a JSON object containing a custom message whenever a request is made to an unhandled endpoint.
Why it's awesome: Spark allows you to define custom error handlers with ease, enabling you to provide meaningful responses for different error scenarios.
Integrating Middleware for Cross-cutting Concerns
Middleware functionality is crucial for adding cross-cutting concerns, such as logging, authentication, and request/response modification, to your Restful APIs. Spark simplifies the integration of middleware through filters.
Logging Requests and Responses
import static spark.Spark.*;
public class LoggingMiddleware {
public static void main(String[] args) {
before((req, res) -> {
System.out.println("Received request: " + req.requestMethod() + " " + req.pathInfo());
});
after((req, res) -> {
System.out.println("Sent response: " + res.status());
});
}
}
In this example, we've used before
and after
filters to log incoming requests and outgoing responses, respectively.
Why it's awesome: Spark's middleware filters enable you to seamlessly integrate cross-cutting concerns into your API without cluttering your route definitions.
Closing Remarks
In conclusion, Spark Java Framework provides a clean and concise way to build Restful APIs in Java. Its simplicity, expressiveness, and lightweight nature make it a preferred choice for developers who want to focus on writing clean and efficient code without sacrificing functionality.
If you're interested in diving deeper into Spark or exploring more advanced features and integrations, you can refer to the official Spark documentation and GitHub repository for further learning.
Start exploring Spark today and experience the joy of building Restful APIs with ease and elegance in Java!