Optimizing Java Virtual Machine Execution Engine
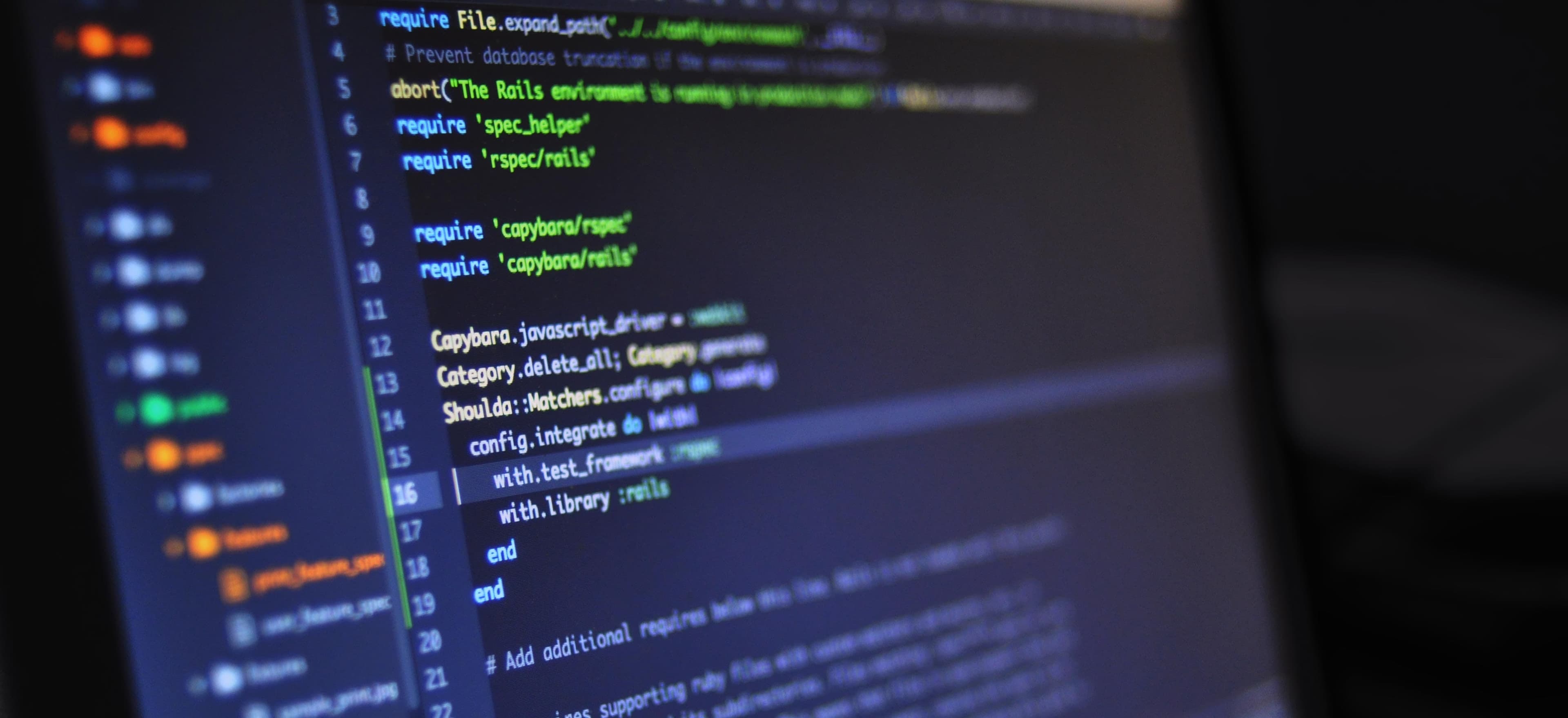
- Published on
Optimizing Java Virtual Machine (JVM) Execution Engine
When it comes to optimizing the performance of Java applications, understanding the Java Virtual Machine (JVM) and its execution engine is crucial. The JVM's execution engine is responsible for executing Java bytecode, and by optimizing its performance, you can significantly improve the overall performance of your Java applications.
In this article, we will explore various techniques for optimizing the JVM's execution engine, including Just-In-Time (JIT) compilation, bytecode optimizations, and runtime profiling.
Just-In-Time (JIT) Compilation
One of the most powerful features of the JVM's execution engine is its ability to dynamically compile bytecode to native machine code at runtime using a technique called JIT compilation. This allows the JVM to optimize the performance of the application by identifying and compiling hotspots - frequently executed code paths - into highly efficient native machine code.
By default, the JVM employs multiple levels of JIT compilation: first, it interprets the bytecode, then it performs JIT compilation to optimize frequently accessed code, and finally, it may perform advanced optimizations based on runtime profiling.
public class JITCompilationExample {
public static void main(String[] args) {
int a = 10;
int b = 20;
int c = a + b;
System.out.println("Sum: " + c);
}
}
In the above example, the JVM may use JIT compilation to optimize the a + b
operation by compiling it to native machine code, resulting in improved performance.
Bytecode Optimizations
In addition to JIT compilation, bytecode optimizations play a crucial role in improving the performance of the JVM's execution engine. The JVM performs various bytecode optimizations to simplify and streamline the execution of the code.
For instance, the JVM may inline methods, eliminate dead code, reorder instructions for better pipelining, and perform constant folding to reduce the number of instructions executed.
public class BytecodeOptimizationsExample {
public int calculate(int x, int y) {
return (x * 2) + (y * 2);
}
}
In the above example, the JVM may apply constant folding to pre-calculate the result of (x * 2)
and (y * 2)
at compile time, resulting in optimized bytecode and improved runtime performance.
Runtime Profiling
Another crucial aspect of optimizing the JVM's execution engine is runtime profiling. Profiling allows the JVM to gather runtime performance data, such as method execution times, memory usage, and hotspots, which can be used to optimize the application's performance.
Tools like Java Flight Recorder and Java Mission Control provide comprehensive profiling capabilities, allowing developers to identify performance bottlenecks and make informed optimization decisions based on real runtime data.
public class RuntimeProfilingExample {
public void performOperation(int iterations) {
for (int i = 0; i < iterations; i++) {
// Perform some operation
}
}
}
By using runtime profiling tools, developers can identify the hotspots in the performOperation
method and apply targeted optimizations to improve its performance.
Closing Remarks
Optimizing the JVM's execution engine is key to achieving high-performance Java applications. By leveraging JIT compilation, bytecode optimizations, and runtime profiling, developers can unlock the full potential of the JVM and deliver efficient and responsive Java applications.
Understanding the inner workings of the JVM's execution engine and applying the optimization techniques discussed in this article will empower you to write high-performance Java code.
In conclusion, optimizing the JVM's execution engine is a multidimensional task that involves understanding JIT compilation, bytecode optimizations, and runtime profiling. By mastering these techniques, you can unleash the full potential of the JVM and deliver high-performance Java applications.
For further reading on optimizing Java applications, consider exploring the official Java Performance Tuning Guide and the Java Platform, Standard Edition Tools and Utilities documentation.
Now, go forth and optimize your Java applications for maximum performance!