Creating and Managing Kubernetes Resources with fabric8 Kubernetes and OpenShift Java DSL
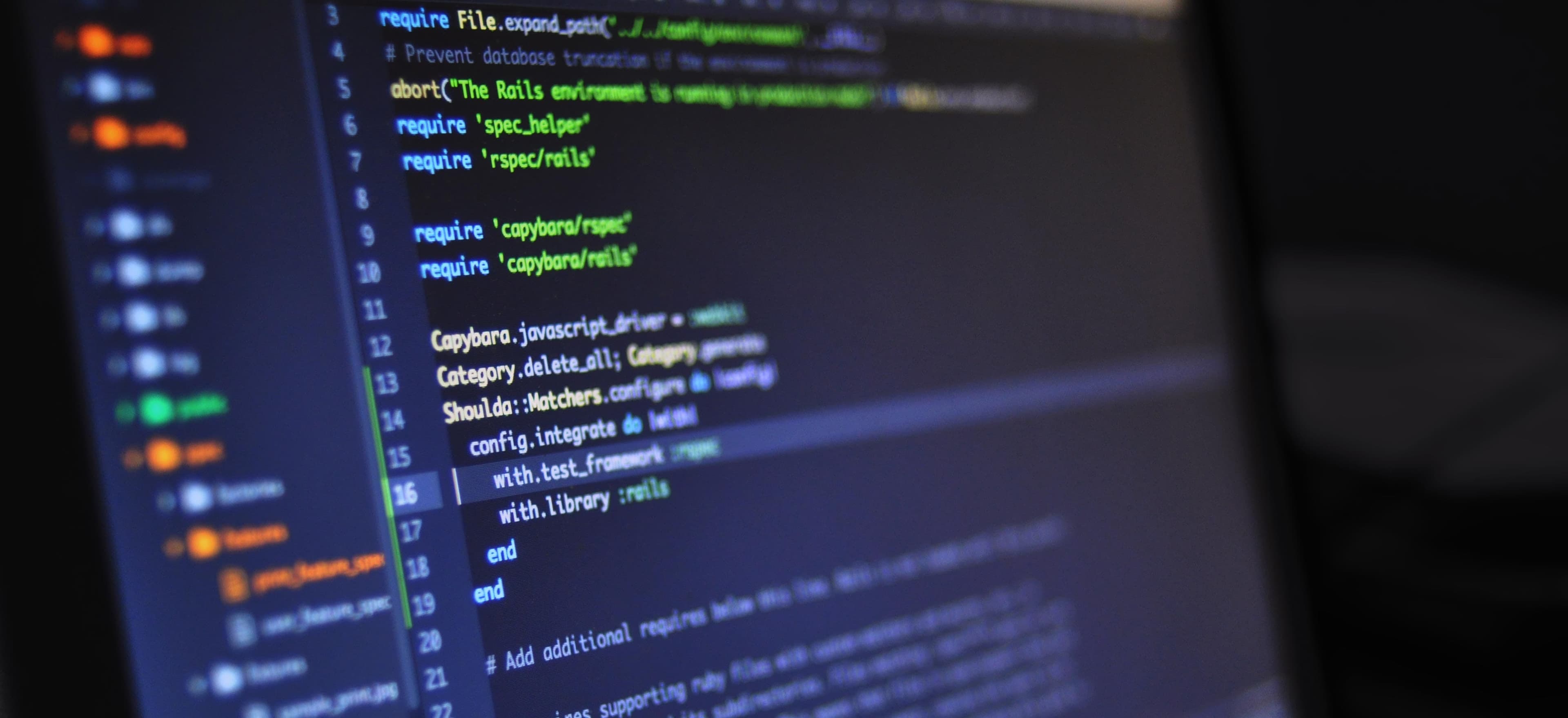
- Published on
Before We Begin
In the world of cloud-native development, managing Kubernetes resources efficiently is crucial. Java developers can leverage the fabric8 Kubernetes and OpenShift Java DSL to easily create, manage, and interact with Kubernetes resources using their familiar Java language.
In this blog post, we will explore how to use the fabric8 Kubernetes and OpenShift Java DSL to create, manage, and work with Kubernetes resources, such as pods, deployments, services, and more. We will provide code examples and explanations to guide you through the process.
What is fabric8 Kubernetes and OpenShift Java DSL?
The fabric8 Kubernetes and OpenShift Java DSL is a Java library that provides a set of fluent APIs for interacting with Kubernetes and OpenShift resources. It allows Java developers to work with Kubernetes resources in a more intuitive and expressive manner, leveraging the power of the Java language.
Prerequisites
Before we dive into using the fabric8 Kubernetes and OpenShift Java DSL, make sure you have the following prerequisites:
- Basic understanding of Kubernetes concepts.
- Java JDK installed on your system.
- Maven or Gradle for dependency management, as per your preference.
- Access to a Kubernetes cluster or Minikube for local development.
Getting Started
To start using the fabric8 Kubernetes and OpenShift Java DSL, you need to include the necessary dependencies in your Java project. For Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>io.fabric8</groupId>
<artifactId>kubernetes-client</artifactId>
<version>5.0.0</version> <!-- replace with the latest version -->
</dependency>
For Gradle, include the following dependency in your build.gradle
:
implementation 'io.fabric8:kubernetes-client:5.0.0' // replace with the latest version
Once you have added the dependency, you can start using the fabric8 Kubernetes and OpenShift Java DSL in your project.
Creating Kubernetes Resources
Let's begin by creating a simple Kubernetes resource, such as a Pod, using the fabric8 Kubernetes Java DSL. Below is an example of how to create a Pod using the DSL:
import io.fabric8.kubernetes.api.model.Pod;
import io.fabric8.kubernetes.api.model.PodBuilder;
import io.fabric8.kubernetes.client.DefaultKubernetesClient;
public class KubernetesResourceCreator {
public static void main(String[] args) {
try (DefaultKubernetesClient client = new DefaultKubernetesClient()) {
Pod pod = new PodBuilder()
.withNewMetadata().withName("example-pod").endMetadata()
.withNewSpec().addNewContainer().withName("nginx").withImage("nginx").endContainer().endSpec()
.build();
client.pods().create(pod);
}
}
}
In the above example, we are creating a new Pod named "example-pod" running an Nginx container. We use the fluent API provided by the fabric8 Kubernetes Java DSL to construct the Pod object and then create it using the Kubernetes client.
Understanding the Code
- We import the necessary classes from the
io.fabric8.kubernetes
package to work with Kubernetes resources. - The
PodBuilder
class allows us to construct a Pod object using a fluent API, making the code more readable and expressive. - We use the
DefaultKubernetesClient
to connect to the Kubernetes cluster and create the Pod using thecreate
method.
Managing Kubernetes Resources
Once we have created Kubernetes resources, it's essential to know how to manage and interact with them. Let's look at an example of how to list all Pods in a Kubernetes cluster and then delete a Pod using the fabric8 Kubernetes Java DSL:
Listing Pods
import io.fabric8.kubernetes.api.model.Pod;
import io.fabric8.kubernetes.client.DefaultKubernetesClient;
import io.fabric8.kubernetes.client.KubernetesClient;
public class KubernetesResourceManager {
public static void main(String[] args) {
try (KubernetesClient client = new DefaultKubernetesClient()) {
System.out.println("List of Pods in the cluster:");
client.pods().list().getItems().forEach(pod -> System.out.println(pod.getMetadata().getName()));
}
}
}
The above code demonstrates how to fetch and list all the Pods present in the Kubernetes cluster using the fabric8 Kubernetes Java DSL. We utilize the list
method to retrieve the list of Pods and then iterate through them to display their names.
Deleting a Pod
import io.fabric8.kubernetes.client.DefaultKubernetesClient;
import io.fabric8.kubernetes.client.KubernetesClient;
public class KubernetesResourceManager {
public static void main(String[] args) {
try (KubernetesClient client = new DefaultKubernetesClient()) {
boolean isDeleted = client.pods().withName("example-pod").delete();
if (isDeleted) {
System.out.println("Pod deleted successfully");
}
}
}
}
In the above code snippet, we demonstrate how to delete a Pod named "example-pod" using the fabric8 Kubernetes Java DSL. We utilize the withName
method to specify the name of the Pod and then call the delete
method to delete it.
Conclusion
In this blog post, we have explored how to use the fabric8 Kubernetes and OpenShift Java DSL to create, manage, and interact with Kubernetes resources using Java. We have covered creating a Pod and managing it by listing and deleting it from the Kubernetes cluster.
The fabric8 Kubernetes and OpenShift Java DSL provides a powerful and expressive way to work with Kubernetes resources in Java, making it an ideal choice for Java developers building cloud-native applications.
By leveraging the fabric8 Kubernetes and OpenShift Java DSL, Java developers can streamline their Kubernetes resource management tasks and focus on building robust and scalable applications within the Kubernetes ecosystem.
Start integrating the fabric8 Kubernetes and OpenShift Java DSL into your Java projects to take full advantage of managing Kubernetes resources efficiently with Java.
For more in-depth information and advanced usage, refer to the fabric8 Kubernetes and OpenShift Java DSL documentation.
Happy Kubernetes resource management with Java!