Optimizing Performance in WSO2 Application Development
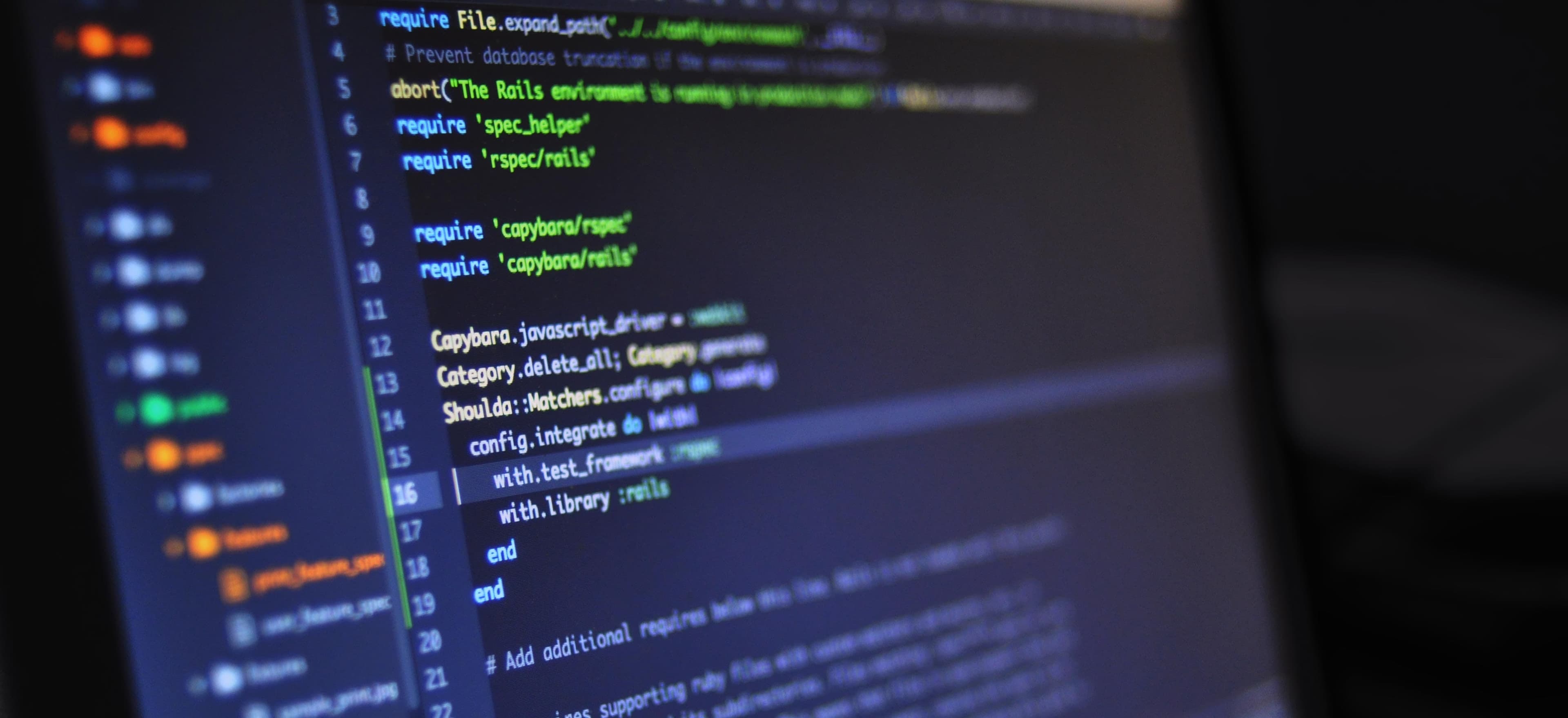
- Published on
Optimizing Performance in WSO2 Application Development
When it comes to developing high-performance applications using WSO2, it's essential to adopt best practices and optimization techniques. In this post, we'll explore various strategies for optimizing the performance of your Java applications developed on the WSO2 platform. We'll delve into topics such as code optimization, caching, database optimization, and tuning WSO2 configurations.
Leveraging Java Best Practices
Writing efficient Java code is crucial for the performance of any application. Utilizing best practices such as using efficient data structures, minimizing object creation, and optimizing loops and conditionals can significantly enhance the performance of your WSO2 applications. Let's illustrate this with a simple example:
// Inefficient code
String result = "";
for (int i = 0; i < 1000; i++) {
result += "value" + i;
}
// Optimized code using StringBuilder
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("value").append(i);
}
String result = sb.toString();
In the above example, using StringBuilder
instead of string concatenation within a loop can greatly improve performance due to reduced memory overhead and better efficiency.
Enhancing Performance through Caching
Caching is a powerful technique for improving the performance of WSO2 applications. By caching frequently accessed data, you can reduce the need for repetitive processing and database queries. WSO2 provides various caching mechanisms such as in-memory caching and distributed caching using technologies like Hazelcast.
// Example of in-memory caching using WSO2 Caching API
CacheManager cacheManager = Caching.getCacheManagerFactory().getCacheManager("sampleCacheManager");
Cache<String, String> cache = cacheManager.getCache("sampleCache");
cache.put("key1", "value1");
String cachedValue = cache.get("key1");
By utilizing caching effectively, you can minimize the response time of your applications and handle higher loads more efficiently.
Database Optimization Strategies
Efficient database interactions play a vital role in the overall performance of WSO2 applications. Optimizing database queries, utilizing appropriate indexing, and reducing unnecessary data retrieval can significantly enhance the application's responsiveness.
// Example of optimized database query using PreparedStatement
String sql = "SELECT * FROM users WHERE username = ?";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement stmt = conn.prepareStatement(sql)) {
stmt.setString(1, "johnDoe");
ResultSet rs = stmt.executeQuery();
// Process the result set
} catch (SQLException e) {
// Handle exceptions
}
In the above example, using PreparedStatement
instead of regular Statement
helps prevent SQL injection and improves query execution performance.
Tuning WSO2 Configurations
WSO2 provides a range of configuration options that allow you to fine-tune various aspects of the platform to optimize performance. Tweaking parameters related to thread pools, connection timeouts, and buffer sizes can have a significant impact on the application's overall throughput and responsiveness.
<!-- Example of tuning HTTP transport receiver in WSO2 -->
<transportReceiver name="http" class="org.wso2.carbon.transport.http.netty.NettyTransportListener">
<parameter name="bossThreadPoolSize">1000</parameter>
<parameter name="workerThreadPoolSize">2000</parameter>
</transportReceiver>
By carefully adjusting these configurations based on the specific requirements of your application, you can extract the maximum performance from the WSO2 platform.
Final Thoughts
In conclusion, optimizing performance in WSO2 application development involves a multi-faceted approach that encompasses efficient Java coding practices, leveraging caching mechanisms, optimizing database interactions, and fine-tuning WSO2 configurations. By incorporating these strategies into your development process, you can ensure that your WSO2 applications deliver exceptional performance and scalability.
By constantly evaluating and refining the performance of your WSO2 applications, you can meet the growing demands of modern enterprise systems while providing an outstanding user experience.
For further information on WSO2 optimization, you can refer to the official WSO2 documentation.
Happy optimizing!