Overcoming Fear of Failure
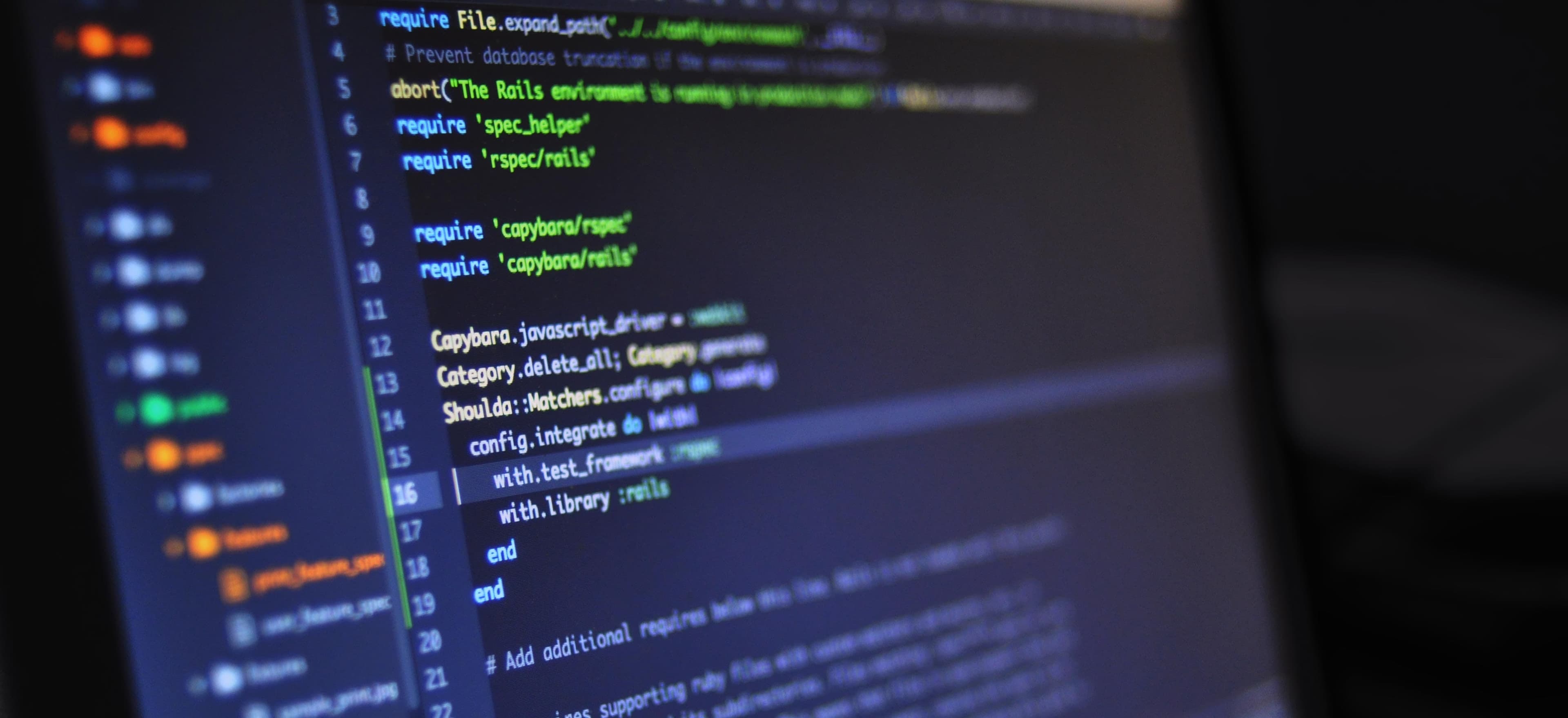
- Published on
Embracing Failure in Java Development
In the world of Java development, the fear of failure is a common hurdle that many developers face. However, it's essential to understand that failure is an integral part of the learning process. In this blog post, we'll explore the concept of failure in Java development and discuss how embracing failure can lead to growth and improvement.
Understanding Failure in Java Development
Failure in Java development can manifest in various forms, such as encountering runtime errors, exceptions, or logical flaws in the code. It's crucial to recognize that failure is not indicative of incompetence, but rather an opportunity to refine one's skills and knowledge.
The Role of Test-Driven Development (TDD)
Test-Driven Development (TDD) is a key practice in Java development that emphasizes writing tests before implementing the code. By deliberately writing tests that are expected to fail initially, developers can confront failure proactively, thereby gaining a deeper understanding of the requirements and potential edge cases.
@Test
public void whenDividingByZero_thenArithmeticExceptionIsThrown() {
Calculator calculator = new Calculator();
assertThrows(ArithmeticException.class, () -> {
calculator.divide(10, 0);
});
}
In this example, the test is intentionally written to fail when dividing by zero, allowing the developer to then implement the handling of this expected failure in the Calculator
class.
Embracing Failure for Personal Growth
Failure should be viewed as a valuable experience that fosters personal and professional growth. In the context of Java development, encountering and addressing failures leads to a deeper understanding of the language, its nuances, and best practices.
Learning from Stack Traces
When encountering runtime errors or exceptions in Java, it's common to analyze the accompanying stack traces to pinpoint the source of the failure. By interpreting stack traces, developers can gain insights into the flow of the program and identify potential areas for improvement.
Exception in thread "main" java.lang.NullPointerException
at com.example.MyClass.myMethod(MyClass.java:10)
at com.example.MyClass.main(MyClass.java:5)
In this stack trace, the NullPointerException
at line 10 of MyClass
highlights a potential issue with null references. By addressing this failure, developers can enhance their code's robustness.
Leveraging Failure in Collaborative Environments
In collaborative Java development environments, failures should be openly discussed and shared among team members. By creating a culture that normalizes failure, developers are more inclined to seek assistance and engage in constructive dialogue, ultimately fostering a collective learning experience.
Conducting Code Reviews
Code reviews are a valuable practice for identifying and addressing failures in Java code. By having peers review the code, potential flaws and improvements can be highlighted, contributing to overall code quality.
/*
Reviewer's Comment:
Potential NullPointerException when accessing 'result' without null check.
Consider adding a null check or handling this scenario appropriately.
*/
public void processResult(Result result) {
if (result != null) {
// Process the result
}
}
In this code review comment, the reviewer identifies a potential NullPointerException
scenario, prompting the developer to revisit the code and address the failure.
Key Takeaways
While the fear of failure is prevalent in Java development, embracing failure as a natural part of the learning process is crucial for personal and professional growth. By incorporating practices such as TDD, learning from stack traces, and fostering a collaborative environment, developers can leverage failures to refine their skills, enhance code quality, and ultimately thrive in the dynamic landscape of Java development.
In summary, in the world of Java development, failure should be embraced, not feared, as it is a catalyst for advancement and improvement.
And remember, as Winston Churchill once said:
"Success is not final, failure is not fatal: It is the courage to continue that counts."
So, let's embrace failure in our Java journey and keep coding fearlessly!