Resolving Code Ownership Disputes Effectively
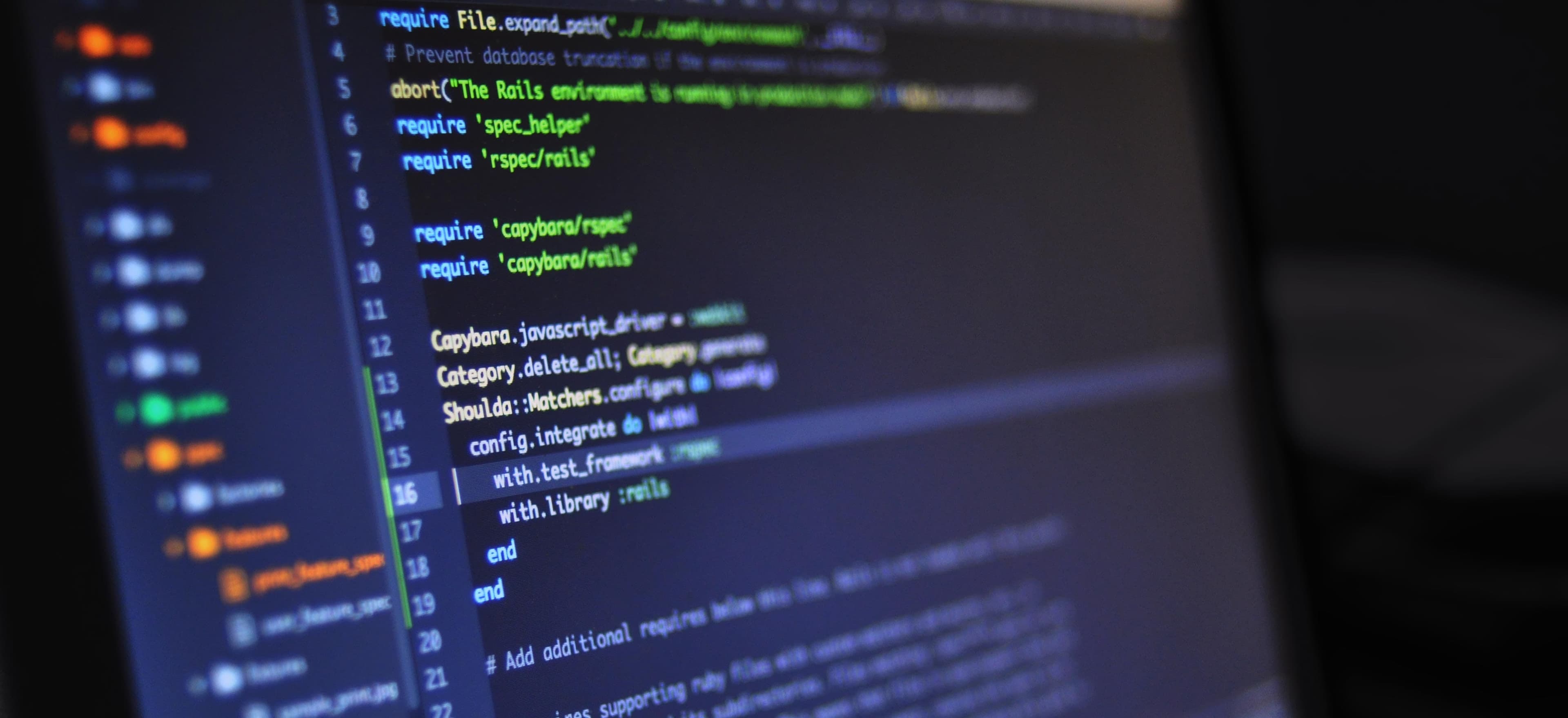
- Published on
Resolving Code Ownership Disputes Effectively
In any collaborative coding environment, conflicts over code ownership can arise. These disputes can hinder productivity, create tension among team members, and ultimately lead to a decline in the quality of the codebase. As such, it is crucial to establish clear guidelines and protocols for managing code ownership disputes within a development team. In this article, we will explore various strategies for resolving code ownership disputes effectively, with a primary focus on Java development teams.
Understanding Code Ownership
Code ownership refers to the concept of taking responsibility for a specific portion of the codebase. It involves understanding, maintaining, and updating the code, and often extends to making decisions about its architecture and design. In many cases, code ownership is implicitly established based on the individuals who have historically worked on a particular section of the code. However, as teams grow and projects evolve, conflicts may arise regarding who should own a specific piece of code.
Establishing Clear Ownership Policies
One of the most effective ways to prevent code ownership disputes is to establish clear ownership policies within the team. These policies should outline the criteria for code ownership, as well as the responsibilities and expectations associated with it. When it comes to Java development, it's essential to define ownership not just at the class or method level, but also at the package and module level.
Example of Ownership Policy in Java
/**
* This class represents a concrete example of how code ownership can be established in Java.
* Each developer is responsible for the classes within a specific package, and they communicate any changes
* to other team members through code reviews and discussions.
*/
package com.example.package1;
public class ExampleClass {
// Class implementation here
}
In the above example, the ownership policy is clearly defined at the package level. Each package is assigned to a specific developer, who is responsible for maintaining the classes within that package.
Encouraging Collaboration
While clear ownership policies are important, it's equally essential to foster a culture of collaboration within the team. Encouraging developers to work together, share knowledge, and provide constructive feedback can help mitigate ownership disputes. In Java development, this often involves establishing code review processes and holding regular knowledge-sharing sessions.
Example of Collaboration through Code Reviews in Java
/**
* This method represents an example of a code review process in Java, where team members provide constructive feedback
* to each other, ultimately improving the quality of the codebase and fostering a collaborative environment.
*/
public void performCodeReview() {
// Code review process implementation here
}
By incorporating regular code reviews into the development workflow, team members can collectively take ownership of the codebase, leading to a more cohesive and collaborative team dynamic.
Mediation and Conflict Resolution
In the event of a code ownership dispute, it's essential to have a mediation and conflict resolution process in place. This process should provide a platform for discussing the dispute and reaching a resolution that is fair and acceptable to all parties involved. In Java development, this could involve leveraging version control systems and issue tracking tools to facilitate discussions and document decisions.
Example of Conflict Resolution using Version Control in Java
/**
* This code snippet represents a scenario where conflicting changes are identified within a version control system like Git,
* and the team utilizes branching and merging strategies to resolve the conflict and reach a consensus on code ownership.
*/
public void resolveCodeConflict() {
// Version control conflict resolution implementation here
}
By utilizing version control systems effectively, teams can better manage and resolve code ownership disputes, ensuring that all decisions and changes are documented and transparent.
Seeking Leadership Intervention
In some cases, unresolved code ownership disputes may require intervention from team leadership or project management. Leaders should facilitate discussions, consider input from all parties, and make decisions that align with the overall goals and objectives of the project. Additionally, leaders can help redefine ownership boundaries and redistribute responsibilities to prevent future conflicts.
In Conclusion, Here is What Matters
Resolving code ownership disputes effectively is essential for maintaining a healthy and productive development environment. By establishing clear ownership policies, fostering collaboration, implementing mediation and conflict resolution processes, and seeking leadership intervention when necessary, Java development teams can navigate ownership disputes with confidence and maintain a strong, cohesive codebase.
In conclusion, proactive communication and adherence to established ownership policies are fundamental to preventing and resolving code ownership disputes effectively within Java development teams. By promoting collaboration and transparency, teams can mitigate conflicts and focus on delivering high-quality code that drives project success.
For further reading on best practices in Java development and fostering a collaborative coding environment, check out the following resources:
- Effective Java: Third Edition
- The Pragmatic Programmer: Your Journey To Mastery
- Collaborative Development in GitHub
Remember, effective conflict resolution and clear ownership guidelines are key to maintaining a harmonious and efficient development environment.