Handling Retryable Operations in Distributed Systems
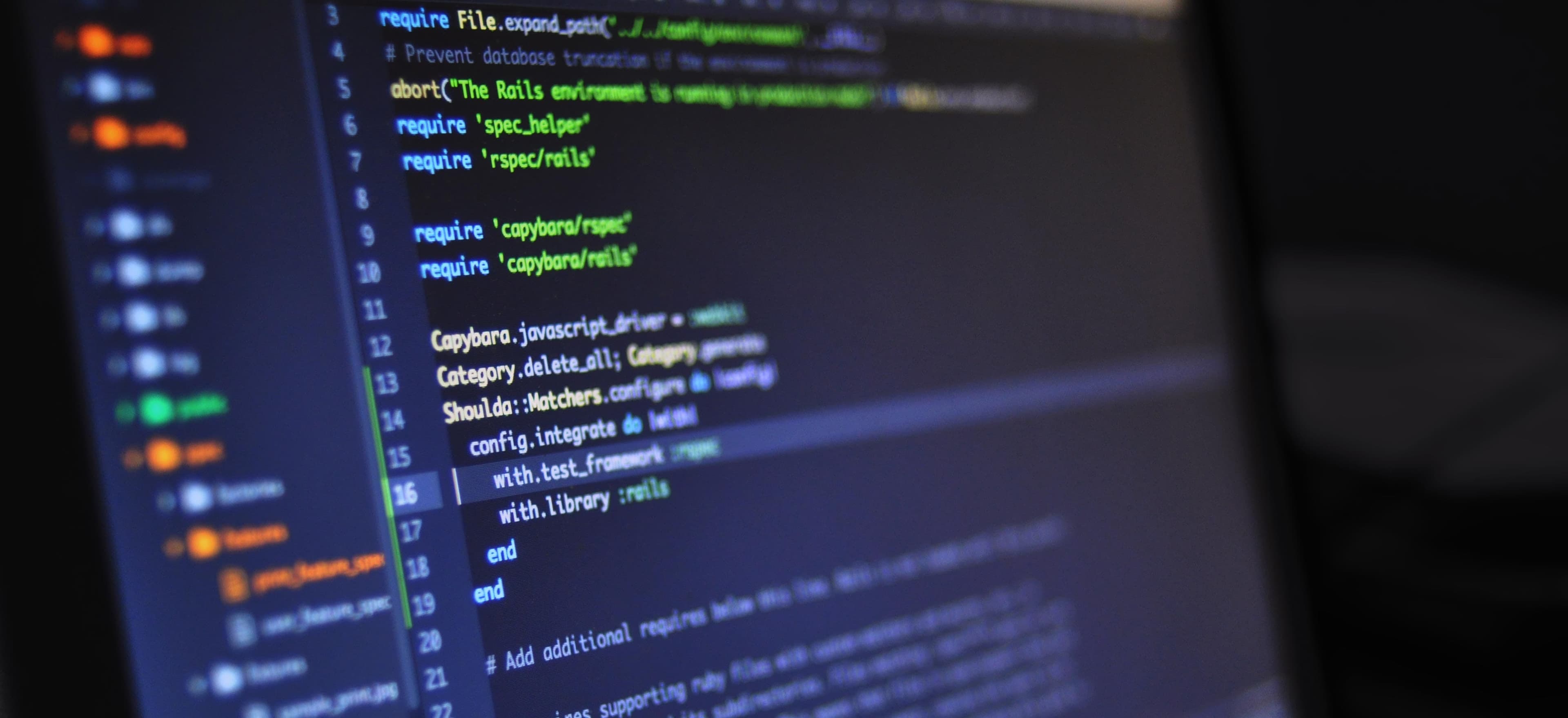
- Published on
Handling Retryable Operations in Distributed Systems
In modern distributed systems, failures can occur at any level - network issues, service unavailability, or even temporary high load. As a result, handling retryable operations has become an essential aspect of building resilient and reliable systems. In this article, we will explore various strategies for handling retryable operations in Java, focusing on best practices and common pitfalls to avoid.
Understanding Retryable Operations
Retryable operations are those that may fail initially but have a higher probability of success upon subsequent attempts. Examples include making network requests, accessing remote services, or interacting with external APIs. When these operations fail, retrying them after a certain delay can often lead to success.
However, blindly retrying operations without a well-thought-out strategy can lead to cascading failures and unnecessary load on the system. It's crucial to implement retry logic in a controlled manner, taking into account factors such as backoff strategies, maximum retry attempts, and idempotency of operations.
Retry Strategies in Java
1. Exponential Backoff
Exponential backoff is a popular retry strategy that introduces an increasing delay between consecutive retry attempts. This approach helps in reducing the load on the system and allows the system to recover from transient failures. Let's take a look at a simple example of implementing exponential backoff in Java:
public class ExponentialBackoff {
public static void retryOperationWithExponentialBackoff(int maxAttempts) {
int retries = 0;
while (retries < maxAttempts) {
try {
// Perform the operation
// If successful, exit the loop
break;
} catch (Exception ex) {
// Handle the exception
long delay = (long) (Math.pow(2, retries) * 1000); // Exponential backoff formula
Thread.sleep(delay);
retries++;
}
}
}
}
In this example, we use an exponentially increasing delay between retries, starting with a base delay of 1 second and doubling it with each attempt. This gradual increase in delay helps in mitigating the impact of a potential surge of concurrent retries.
2. Fixed Interval Retry
In some cases, a fixed interval retry strategy may be more suitable, especially for operations with known stability and response times. This approach involves retrying the operation at fixed intervals, regardless of the previous outcomes. Let's consider a scenario where we want to retry an operation every 5 seconds for a maximum of 3 attempts:
public class FixedIntervalRetry {
public static void retryOperationWithFixedInterval(int maxAttempts, int intervalInSeconds) {
int retries = 0;
while (retries < maxAttempts) {
try {
// Perform the operation
// If successful, exit the loop
break;
} catch (Exception ex) {
// Handle the exception
Thread.sleep(intervalInSeconds * 1000);
retries++;
}
}
}
}
The fixed interval retry strategy provides a more predictable retry pattern, which can be beneficial for certain types of operations.
3. Circuit Breaker Pattern
In addition to retry strategies, the circuit breaker pattern is often employed to prevent the continuous retrying of operations that are unlikely to succeed in the near future. The circuit breaker monitors the state of the operation and "trips" or opens when the failure rate exceeds a certain threshold. This allows the system to fail fast and prevent cascading failures.
There are various Java libraries, such as Resilience4j and Hystrix, that provide implementations of the circuit breaker pattern and integrate seamlessly with retry logic.
Best Practices for Handling Retryable Operations
-
Limit the Number of Retries: Setting a reasonable limit on the number of retry attempts prevents endless looping in case of persistent failures. It also helps in preventing excessive load on the system.
-
Idempotent Operations: Where possible, make the retryable operations idempotent, meaning that they produce the same result regardless of the number of retries. This property is crucial for ensuring correctness when retrying operations.
-
Logging and Monitoring: Implement thorough logging and monitoring for retryable operations to track the occurrence of retries, identify underlying issues, and analyze the effectiveness of the chosen retry strategy.
-
Handle Specific Exceptions: When implementing retry logic, be specific about the types of exceptions that warrant retry attempts. Blindly retrying all exceptions can lead to unintended consequences and hide underlying issues.
Common Pitfalls to Avoid
-
Infinite Retries: Failing to define an upper limit on retry attempts can lead to infinite loops and put unnecessary strain on the system. Always set a maximum retry count and consider implementing a circuit breaker for scenarios of prolonged failure.
-
Lack of Exponential Backoff: Using a fixed retry interval without any backoff strategy can exacerbate the impact of transient failures, leading to increased contention and potential overload on the system.
-
Ignoring Failure Causes: Relying solely on retry logic without investigating the root causes of failures can compound issues and mask systemic problems. It's important to address underlying issues proactively rather than solely relying on retries.
-
Unmonitored Retries: Failing to monitor and analyze the effectiveness of retry attempts can result in a lack of visibility into the overall system health and performance.
Key Takeaways
Handling retryable operations in distributed systems is a critical aspect of building robust and reliable applications. By incorporating well-designed retry strategies, such as exponential backoff and circuit breakers, and adhering to best practices, Java developers can minimize the impact of transient failures and improve the resilience of their systems.
Incorporating these best practices and avoiding common pitfalls will contribute to the overall stability and performance of distributed systems, ensuring that retryable operations are executed in a controlled and efficient manner.
In conclusion, adopting a thoughtful approach to retryable operations not only enhances the reliability of distributed systems but also contributes to a positive user experience by minimizing the impact of transient failures and providing a more resilient application architecture.
By carefully considering and implementing the discussed strategies and best practices, Java developers can effectively handle retryable operations in distributed systems, ultimately contributing to the robustness and reliability of their applications.
Additional Resources:
Checkout our other articles