Debugging Arquillian Tests in NetBeans with WebLogic 12c
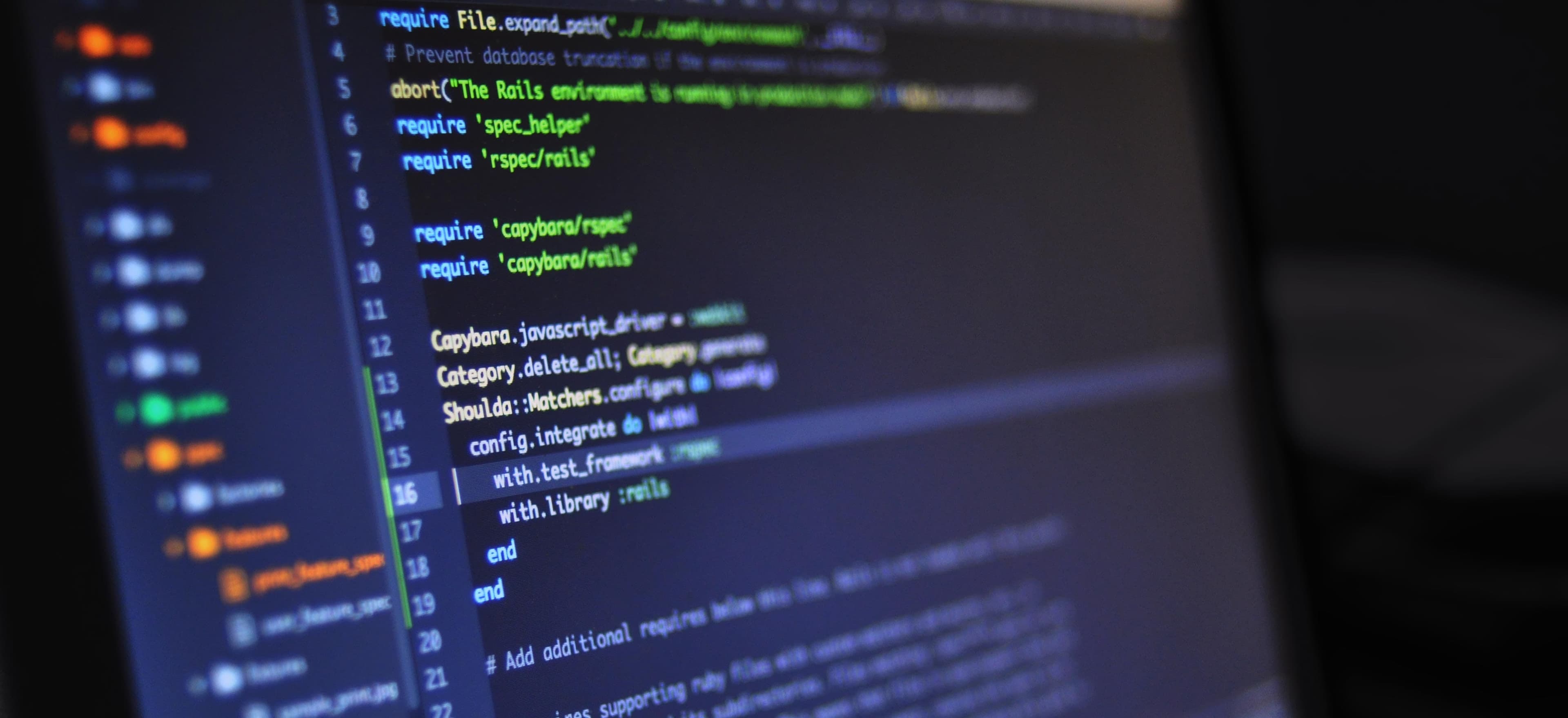
- Published on
Debugging Arquillian Tests in NetBeans with WebLogic 12c
When it comes to testing Java EE applications, Arquillian is a powerful and flexible testing platform. However, debugging Arquillian tests in an integrated development environment (IDE) like NetBeans with a server like WebLogic 12c can be quite a challenge. This guide will walk you through the process of setting up and debugging Arquillian tests in NetBeans with WebLogic 12c, helping you streamline your testing and debugging workflow.
Setting Up Arquillian in NetBeans
Before we dive into debugging, let's ensure we have Arquillian set up in NetBeans. To do this, we need to create a new project or open an existing one in NetBeans.
Adding Arquillian Libraries
To add Arquillian support to your project, follow these steps:
- Right-click on your project in NetBeans and select "Properties".
- Click on "Libraries" and then select "Add Library".
- Choose "Arquillian" from the list and click "Add Library".
This will add the necessary Arquillian libraries to your project, allowing you to write and run Arquillian tests within NetBeans.
Configuring Arquillian Container
Once the Arquillian libraries are added, you will need to configure the Arquillian container to use WebLogic 12c. This can be done by creating an arquillian.xml
file in the src/test/resources
directory of your project. Here's an example configuration for WebLogic 12c:
<arquillian xmlns="http://jboss.org/schema/arquillian"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://jboss.org/schema/arquillian
http://jboss.org/schema/arquillian/arquillian_1_0.xsd">
<container qualifier="weblogic" default="true">
<configuration>
<property name="managementAddress">localhost</property>
<property name="managementPort">7001</property>
<property name="adminUserName">weblogic</property>
<property name="adminPassword">welcome1</property>
</configuration>
</container>
</arquillian>
In this configuration, we specify the management address, port, admin username, and password for our WebLogic 12c server. Replace these values with your specific server details.
Writing Arquillian Tests
With the setup out of the way, let's write a simple Arquillian test that we can use for debugging. For this example, let's assume we have an EJB named Calculator
with a method add
that adds two numbers.
@Stateless
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Now, let's write an Arquillian test for this EJB:
@RunWith(Arquillian.class)
public class CalculatorTest {
@EJB
private Calculator calculator;
@Deployment
public static Archive<?> createDeployment() {
return ShrinkWrap.create(JavaArchive.class, "test.jar")
.addClasses(Calculator.class);
}
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3));
}
}
In this test, we use @RunWith(Arquillian.class)
to enable Arquillian, inject the Calculator
EJB using @EJB
, and create a deployment archive using ShrinkWrap. The testAdd
method then tests the add
method of the Calculator
EJB.
Debugging Arquillian Tests in NetBeans
Now that we have written our Arquillian test, let's see how we can debug it in NetBeans using WebLogic 12c as the server.
Setting Breakpoints
To debug the Arquillian test, we need to set breakpoints in our test and the code being tested. In NetBeans, simply click on the left margin of the line where you want to set a breakpoint to toggle it.
For instance, we can set a breakpoint inside the testAdd
method to inspect the behavior of the add
method in the Calculator
EJB.
Running in Debug Mode
To run the Arquillian test in debug mode, right-click on the test file in NetBeans and select "Debug File" or use the shortcut Shift + F5
. This will start the Arquillian test in debug mode, allowing you to hit the breakpoints and inspect the test execution.
Inspecting Variables and Call Stack
While the test is paused at a breakpoint, you can inspect the values of variables and the call stack to understand the state of the application at that point. NetBeans provides a user-friendly interface for inspecting variables and exploring the call stack, helping you troubleshoot issues with your Arquillian tests.
Recap
In this blog post, we've explored the process of debugging Arquillian tests in NetBeans with WebLogic 12c. We started by setting up Arquillian in NetBeans, followed by writing a simple Arquillian test, and finally, we delved into the debugging process using NetBeans' debugging capabilities.
By leveraging the power of Arquillian and the debugging features of NetBeans, you can efficiently write, run, and debug your Java EE applications, ensuring they are robust and reliable.
Now that you have a solid understanding of debugging Arquillian tests in NetBeans with WebLogic 12c, you're equipped to enhance your testing workflow and achieve greater confidence in the quality of your Java EE applications.
Happy debugging!