Common Mistakes in Using Parsing Objects
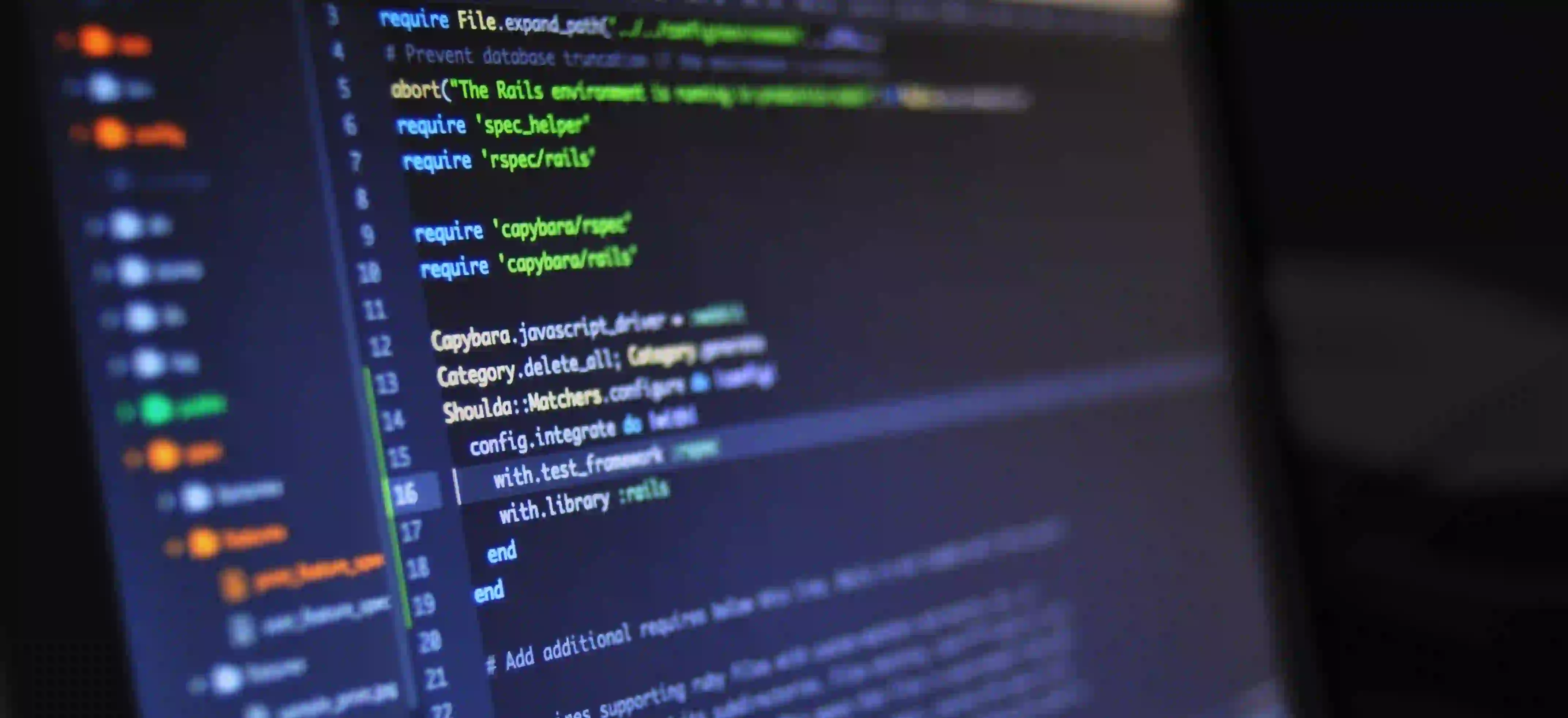
Common Mistakes in Using Parsing Objects
When working with parsing objects in Java, it's easy to make mistakes that can lead to unexpected behavior or errors in your code. Whether you're working with JSON, XML, or any other type of data, understanding how to use parsing objects effectively is crucial for building robust and reliable applications.
In this article, we'll explore some common mistakes that developers make when using parsing objects in Java, and we'll discuss how to avoid them.
Mistake 1: Not Handling Exceptions
One of the most common mistakes when working with parsing objects is not properly handling exceptions. When parsing data, there are many things that can go wrong, such as malformed input, missing fields, or invalid data types. Failing to catch and handle these exceptions can result in runtime errors that crash your application.
try {
// Parsing code
} catch (Exception e) {
// Handle the exception
}
Always surround your parsing code with a try-catch
block and handle any potential exceptions appropriately. This will make your code more robust and prevent unexpected crashes.
Mistake 2: Not Closing Resources
Another common mistake is not properly closing parsing object resources after they are no longer needed. This can lead to resource leaks and potentially slow down or crash your application, especially if you are parsing a large amount of data.
try {
// Parsing code
} finally {
// Close the parsing object resources
}
Use a finally
block to ensure that parsing object resources are always closed, even if an exception is thrown during the parsing process. This will help prevent resource leaks and keep your application running smoothly.
Mistake 3: Not Using the Correct Parsing Object
It's crucial to use the correct parsing object for the type of data you are working with. For example, if you're parsing JSON data, you should use a JSON parsing library such as Gson or Jackson. Using the wrong parsing object can lead to parsing errors and unexpected behavior in your application.
// Using Gson for JSON parsing
Gson gson = new Gson();
MyObject object = gson.fromJson(jsonString, MyObject.class);
Be sure to research and choose the appropriate parsing library for the type of data you need to parse, and familiarize yourself with its documentation and best practices.
Mistake 4: Not Validating Input Data
Failing to validate input data before parsing can also lead to errors in your code. Always ensure that the data you are parsing meets the expected format and requirements before attempting to parse it. This can help prevent parsing errors and ensure that your code behaves as intended.
if (isValidJson(jsonString)) {
// Parse the JSON data
} else {
// Handle invalid input
}
Create validation checks for the input data before initiating the parsing process to avoid unexpected exceptions or incorrect parsing results.
Mistake 5: Not Handling Null or Missing Values
It's essential to handle null or missing values when parsing data, especially when working with optional fields or dynamic data sources. Failing to do so can result in NullPointerExceptions or incorrect data in your application.
String value = jsonObject.has("key") ? jsonObject.getString("key") : "default value";
Always check for the existence of a field before attempting to retrieve its value, and provide a default value or handle the absence of the field accordingly.
Final Thoughts
Avoiding these common mistakes when using parsing objects in Java can significantly improve the reliability and performance of your code. By properly handling exceptions, closing resources, using the correct parsing object, validating input data, and handling null or missing values, you can ensure that your parsing code is robust and error-free.
Remember to always refer to the documentation and best practices of the parsing library you are using, and continuously test your parsing code with various types of input data to identify and address any potential issues.
Keep these best practices in mind, and your parsing code will be more resilient and less prone to errors, resulting in a smoother and more reliable application.
For further learning about parsing objects and their best practices, check out the following resources:
- Oracle's Java Documentation
- Gson User Guide
- Jackson Documentation
Start implementing these best practices in your parsing code today, and elevate the quality of your Java applications!