Microservices: Ensuring Seamless Service Discovery
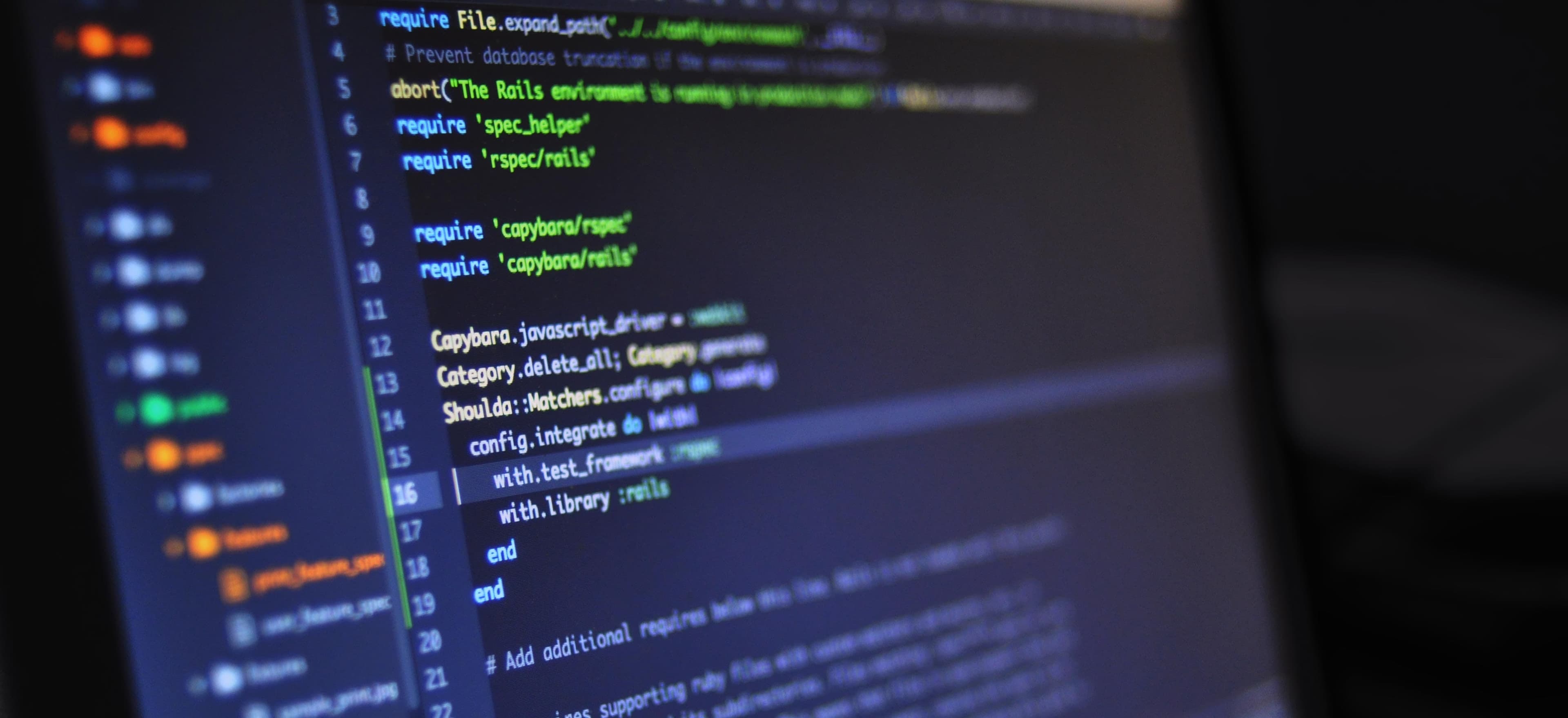
- Published on
Microservices: Ensuring Seamless Service Discovery
In the world of microservices architecture, one of the key challenges is ensuring seamless service discovery. As the number of services grows, it becomes crucial to have a reliable and efficient mechanism for services to discover and communicate with each other. This is where service discovery comes into play.
What is Service Discovery?
Service discovery is the process by which services locate and communicate with each other. In a microservices architecture, where services are distributed and dynamic, traditional methods of service discovery, such as static configuration files, are no longer effective. Instead, dynamic service discovery mechanisms are required to accommodate the ever-changing nature of microservices.
The Role of Service Discovery in Microservices
In a microservices architecture, services are constantly being added, removed, or scaled up and down based on demand. As a result, the locations and IP addresses of services are not fixed, making it challenging for one service to find and communicate with another. Here's where service discovery comes in - it provides a dynamic way for services to find each other, regardless of their locations or underlying infrastructure.
The Importance of Seamless Service Discovery
Seamless service discovery is crucial for the overall health and performance of a microservices-based system. Without it, services would not be able to locate each other, leading to communication failures and ultimately, system instability. Additionally, seamless service discovery enables the implementation of advanced microservices patterns such as dynamic routing, load balancing, and failover.
Implementing Service Discovery in Java
In the Java ecosystem, several tools and libraries facilitate seamless service discovery. One of the most popular and effective approaches is to use a service registry in conjunction with a client-side service discovery mechanism.
Service Registry
A service registry, such as Netflix Eureka or Consul, acts as a central database of service locations and metadata. When a service instance starts up, it registers itself with the registry, providing essential details such as its hostname, IP address, and port. Other services can then query the registry to discover and communicate with the registered services.
Client-Side Service Discovery
In a client-side service discovery approach, the responsibility of service discovery lies with the client making the outbound request. When a service needs to communicate with another service, it queries the service registry to obtain the location of the target service. With this information, the client can then directly communicate with the discovered service.
Code Implementation
Let's delve into a simple implementation of client-side service discovery using Spring Cloud Netflix Eureka. In this example, we'll create a basic RESTful microservice that registers itself with the Eureka server and communicates with another service discovered through Eureka.
@SpringBootApplication
@EnableEurekaClient
@RestController
public class DemoServiceApplication {
@GetMapping("/hello")
public String hello() {
RestTemplate restTemplate = new RestTemplate();
ResponseEntity<String> response = restTemplate.getForEntity("http://discovered-service/hello", String.class);
return "Response from discovered service: " + response.getBody();
}
public static void main(String[] args) {
SpringApplication.run(DemoServiceApplication.class, args);
}
}
In the above code, we have a simple Spring Boot application that registers itself as a Eureka client using @EnableEurekaClient
. The /hello
endpoint makes a REST call to another service named "discovered-service"
. By utilizing the service name instead of hardcoding the service's location, we enable dynamic service discovery through Eureka.
This code exemplifies the elegance and simplicity of integrating service discovery within a Java microservices application. Instead of dealing with static URLs or IP addresses, the service leverages the dynamic nature of service discovery to locate and communicate with other services seamlessly.
Closing the Chapter
Seamless service discovery is imperative in a microservices architecture, ensuring that services can dynamically locate and communicate with each other. In the Java ecosystem, tools like Netflix Eureka and techniques such as client-side service discovery simplify the implementation of service discovery within microservices.
By embracing service discovery, Java microservices can achieve a high degree of resilience, scalability, and fault tolerance, thereby enabling the efficient communication and coordination of services within a distributed system.
In conclusion, prioritizing seamless service discovery is fundamental to the success of microservices, and the Java ecosystem provides the necessary tools and practices to achieve this essential architectural requirement.
To delve deeper into service discovery and its significance in microservices, check out this insightful article on Microservices Service Discovery and Registry. Additionally, for a comprehensive understanding of service discovery patterns and best practices, refer to this informative guide on Service Discovery in a Microservices Architecture.
With the emergence of microservices, ensuring seamless service discovery is more crucial than ever, and Java developers have the means to implement robust and efficient service discovery mechanisms within their microservices applications.