Efficient Real-Time Messaging with STOMP Over WebSockets
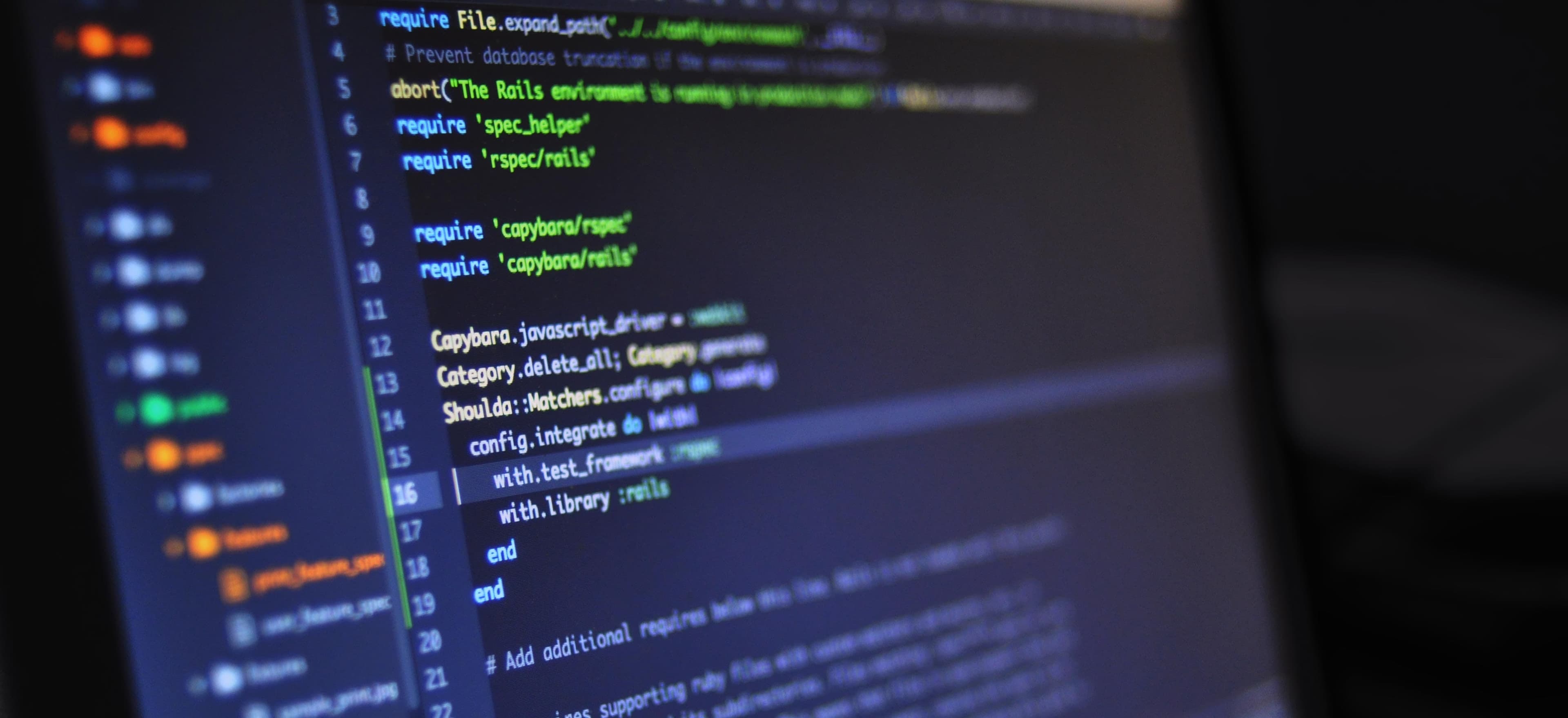
- Published on
Efficient Real-Time Messaging with STOMP Over WebSockets
In today's fast-paced world, real-time messaging has become a crucial aspect of many applications. Whether it's a chat application, live updates in a web application, or real-time analytics, the need for efficient real-time messaging is undeniable. To achieve this, the use of messaging protocols such as STOMP (Simple Text Oriented Messaging Protocol) over WebSockets has gained significant traction.
Understanding STOMP and WebSockets
What is STOMP?
STOMP is a simple, interoperable wire format that allows clients to communicate with message brokers. It provides a lightweight and easy-to-implement messaging protocol suitable for a wide range of applications.
What are WebSockets?
WebSockets is a communication protocol that provides full-duplex communication channels over a single TCP connection. It enables interaction between a web browser and a web server with lower overhead, resulting in real-time data exchange.
Integrating STOMP Over WebSockets in Java
Using Spring Framework
Dependency Configuration
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-messaging</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-websocket</artifactId>
<version>5.3.9</version>
</dependency>
Incorporating the Spring framework allows for seamless integration of STOMP over WebSockets in Java applications. It provides an elegant solution for real-time messaging needs.
Initializing WebSocket Configuration
WebSocket Configuration Class
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig extends AbstractWebSocketMessageBrokerConfigurer {
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/ws").withSockJS();
}
public void configureMessageBroker(MessageBrokerRegistry registry) {
registry.enableSimpleBroker("/topic");
registry.setApplicationDestinationPrefixes("/app");
}
}
Here, we define the WebSocket configuration where incoming messages are routed to the message broker and outgoing messages are routed to the WebSocket.
Creating a STOMP Controller
STOMP Controller Class
@Controller
public class StompController {
@MessageMapping("/sendMessage")
@SendTo("/topic/messages")
public Message sendMessage(Message message) {
return message;
}
}
The STOMP controller handles incoming messages and sends them to the specified destination ("/topic/messages" in this case).
Connecting to the WebSocket from the Client Side
Client-Side Connection
var stompClient = Stomp.over(new SockJS('/ws'));
stompClient.connect({}, function (frame) {
stompClient.subscribe('/topic/messages', function (response) {
// Process the received message
});
});
On the client side, the connection to the WebSocket is established using STOMP to subscribe to a specific destination ("/topic/messages") and handle the received messages.
Advantages of Using STOMP Over WebSockets
-
Efficiency: STOMP over WebSockets reduces latency and provides real-time communication capabilities, making it ideal for applications requiring instant updates.
-
Interoperability: STOMP's simple and text-oriented nature allows it to be easily integrated into a wide range of technologies and platforms.
-
Reliability: The use of WebSockets ensures a robust and continuous connection, minimizing the risk of message loss.
-
Scalability: With the ability to handle a large number of concurrent connections, STOMP over WebSockets is scalable for high-demand applications.
To Wrap Things Up
Integrating STOMP over WebSockets in Java applications opens up a world of possibilities for efficient real-time messaging. The combination of STOMP's simplicity and interoperability with WebSockets' real-time capabilities provides a powerful solution for modern applications.
As the demand for real-time communication continues to grow, implementing STOMP over WebSockets is not only a best practice but also a necessity for delivering seamless and responsive user experiences.
By leveraging the capabilities of STOMP over WebSockets, Java developers can empower their applications with efficient real-time messaging, setting the stage for highly interactive and dynamic user interactions.
To delve deeper into the world of real-time messaging and its implementation in Java, explore the official documentation for Spring WebSocket and STOMP.