Finding the Middle Point of Two LatLong Coordinates
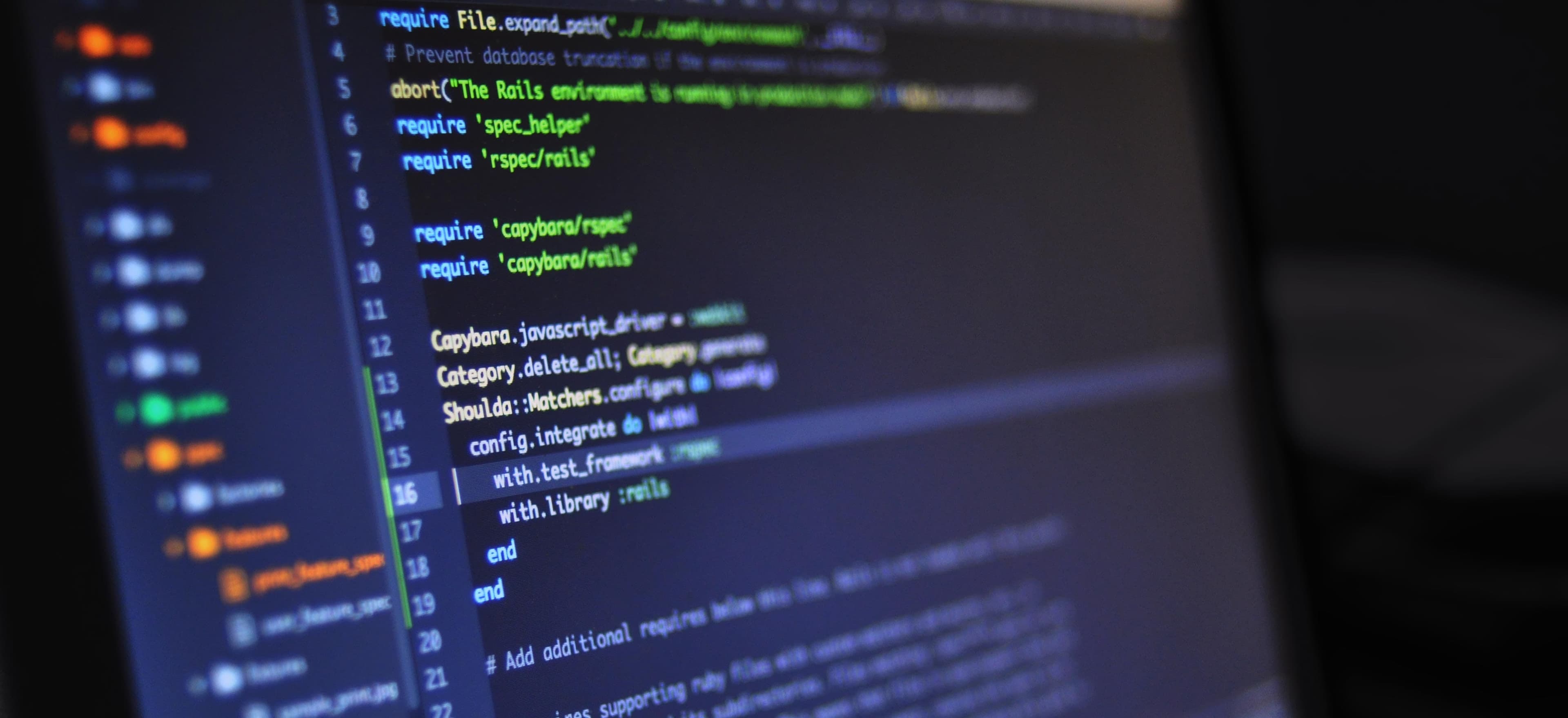
- Published on
Calculating the Middle Point of Two LatLong Coordinates in Java
When working with geographical coordinates, there are often times when you need to find the middle point between two sets of coordinates. This can be useful in various applications such as mapping, navigation, and geospatial analysis. In this blog post, we will delve into how to calculate the middle point of two LatLong coordinates using Java.
Understanding LatLong Coordinates
LatLong (Latitude and Longitude) coordinates are used to specify a point on the Earth's surface. Latitude measures how far north or south a point is from the Equator, while Longitude measures how far east or west a point is from the Prime Meridian. In Java, we can represent coordinates using the double
data type.
The Haversine Formula
To calculate the middle point between two LatLong coordinates, we can use the Haversine formula. The Haversine formula is a well-known equation used for calculating distances between two points on the Earth, given their latitude and longitude. While traditionally used for distance calculation, the formula can also be employed to find the intermediate point between two coordinates.
Implementing the Haversine Formula
Let's start by creating a Java method that takes two sets of LatLong coordinates as input and calculates the middle point using the Haversine formula. Here's a sample implementation of the method:
public class LatLongUtils {
public static LatLong calculateMiddlePoint(LatLong coord1, LatLong coord2) {
double lat1 = Math.toRadians(coord1.getLatitude());
double lon1 = Math.toRadians(coord1.getLongitude());
double lat2 = Math.toRadians(coord2.getLatitude());
double lon2 = Math.toRadians(coord2.getLongitude());
double Bx = Math.cos(lat2) * Math.cos(lon2 - lon1);
double By = Math.cos(lat2) * Math.sin(lon2 - lon1);
double lat3 = Math.atan2(Math.sin(lat1) + Math.sin(lat2),
Math.sqrt((Math.cos(lat1) + Bx) * (Math.cos(lat1) + Bx) + By * By));
double lon3 = lon1 + Math.atan2(By, Math.cos(lat1) + Bx);
return new LatLong(Math.toDegrees(lat3), Math.toDegrees(lon3));
}
}
In this LatLongUtils
class, we have a method calculateMiddlePoint
that takes two LatLong
objects as input and returns a new LatLong
representing the middle point. We first convert the input coordinates from degrees to radians using Math.toRadians
as the Haversine formula uses trigonometric functions that operate on radians.
Next, we calculate intermediate values Bx
and By
using trigonometric functions. Finally, we apply the inverse operations to obtain the latitude and longitude of the middle point in degrees, which we then return as a new LatLong
object.
Testing the Method
Let's test our calculateMiddlePoint
method with some sample LatLong coordinates:
public class Main {
public static void main(String[] args) {
LatLong coord1 = new LatLong(40.7128, -74.0060); // New York City
LatLong coord2 = new LatLong(34.0522, -118.2437); // Los Angeles
LatLong middlePoint = LatLongUtils.calculateMiddlePoint(coord1, coord2);
System.out.println("The middle point is: " + middlePoint.getLatitude() + ", " + middlePoint.getLongitude());
}
}
When running the above code, we should see the calculated middle point printed to the console. In this example, we used the coordinates for New York City and Los Angeles as input, and the output should represent the middle point between these two locations.
Wrapping Up
In this blog post, we explored how to calculate the middle point of two LatLong coordinates using the Haversine formula in Java. Understanding geographical calculations and coordinate systems is essential for various applications, and having the ability to derive the middle point between coordinates can be valuable in many scenarios.
By implementing the Haversine formula in Java and testing it with sample coordinates, we have demonstrated a practical approach to solving this problem. As you continue to work on geospatial projects in Java, this knowledge will serve as a fundamental building block for handling geographic data and calculations.