Optimizing API Development for DeFi Ecosystem
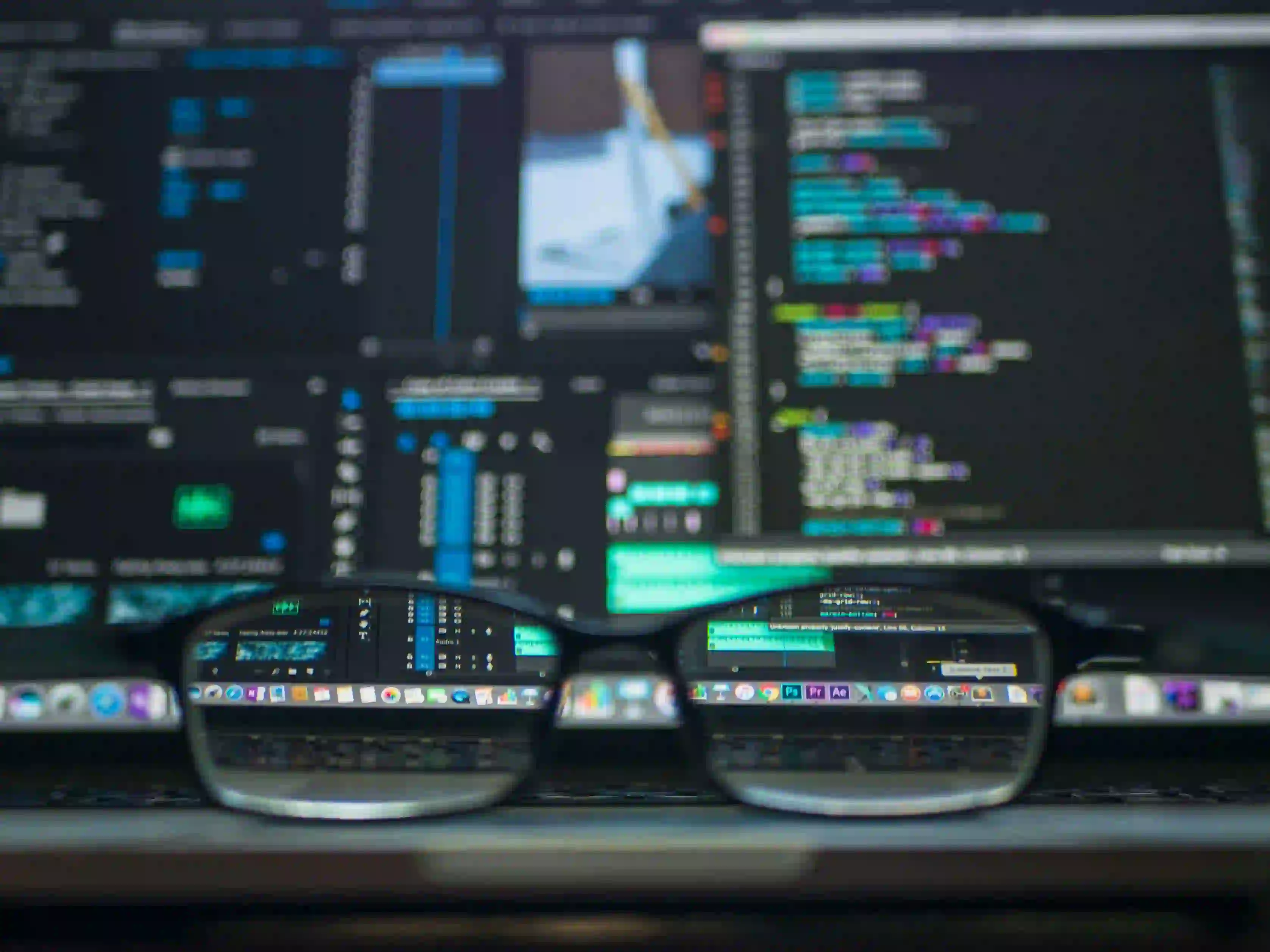
Optimizing API Development for DeFi Ecosystem
In the rapidly growing and evolving world of decentralized finance (DeFi), the demand for efficient and secure API development has never been higher. DeFi applications rely heavily on various APIs to access, process, and integrate with different protocols, smart contracts, and blockchain networks. This post will explore the importance of optimizing API development for the DeFi ecosystem and discuss best practices using Java, a powerful and widely-used programming language.
The Growing Importance of DeFi APIs
As the DeFi ecosystem expands, the need for reliable, performant, and secure APIs becomes increasingly critical. DeFi applications depend on seamless interactions with blockchain networks, decentralized exchanges, lending platforms, and oracles. Well-designed APIs not only facilitate these interactions but also play a crucial role in ensuring the security and integrity of the entire DeFi infrastructure.
The Role of Java in DeFi API Development
Java, with its strong emphasis on performance, scalability, and security, is an ideal choice for developing DeFi APIs. Its robust ecosystem, mature libraries, and extensive community support make it well-suited for building and maintaining mission-critical financial applications. Additionally, the inherent portability of Java allows DeFi APIs to run on different platforms with minimal modifications.
Best Practices for Optimizing DeFi APIs with Java
1. Use Asynchronous Programming for Enhanced Performance
In DeFi applications, where real-time data and responsiveness are paramount, leveraging asynchronous programming can significantly boost API performance. Java offers excellent support for asynchronous programming through libraries like CompletableFuture and Reactive Streams. By utilizing non-blocking I/O and asynchronous operations, DeFi APIs can efficiently handle concurrent requests and minimize response times.
// Example of CompletableFuture in Java
CompletableFuture.supplyAsync(() -> {
// Asynchronous operation
return fetchDataFromBlockchain();
}).thenApply(data -> {
// Process the data
return processAndTransformData(data);
}).thenAccept(result -> {
// Handle the result
returnResultToClient(result);
});
2. Implement Robust Error Handling and Fault Tolerance
In the context of DeFi, where the stakes are high, resilient error handling is non-negotiable. Java's exception handling mechanism, coupled with frameworks like Hystrix or resilience4j, empowers developers to build fault-tolerant APIs. By gracefully handling errors, timeouts, and retries, DeFi APIs can maintain stability and availability even in the face of unexpected failures.
// Example of fault tolerance with resilience4j
@CircuitBreaker(name = "defiApi", fallbackMethod = "fallbackMethod")
public ApiResponse<> fetchData() {
// API call to DeFi protocol
}
3. Leverage Caching for Improved Latency and Scalability
Efficient data retrieval is crucial for DeFi APIs, and caching frequently accessed information can significantly reduce latency and alleviate pressure on backend systems. Java provides robust caching solutions such as caffeine or Ehcache, allowing developers to store and retrieve data with minimal overhead. By strategically caching blockchain responses, DeFi APIs can deliver faster and more responsive user experiences.
// Example of caching with Caffeine
LoadingCache<String, String> cache = Caffeine.newBuilder().expireAfterWrite(5, TimeUnit.MINUTES).build(key -> fetchDataFromBlockchain(key));
String cachedData = cache.get("key");
4. Prioritize Security and Compliance
Security is paramount in the DeFi landscape, and Java's emphasis on strong typing, memory management, and secure coding practices aligns well with the stringent security requirements of DeFi APIs. Additionally, integrating well-established security libraries like Apache Shiro or Spring Security can fortify DeFi APIs against vulnerabilities and unauthorized access, ensuring compliance with regulatory standards.
// Example of securing API with Spring Security
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/**").authenticated()
.anyRequest().permitAll()
.and()
.httpBasic();
}
}
5. Embrace OpenAPI Standards for Interoperability
Adopting standardized API specifications like OpenAPI (formerly Swagger) fosters interoperability and seamless integration within the DeFi ecosystem. Java offers robust support for defining and documenting APIs using libraries like SpringFox or OpenAPI-generator. By adhering to OpenAPI standards, DeFi APIs can enhance discoverability, facilitate collaboration, and streamline the integration process for developers and third-party applications.
// Example of using OpenAPI with SpringFox
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.defi.api"))
.paths(PathSelectors.any())
.build();
}
}
In Conclusion, Here is What Matters
Optimizing API development for the DeFi ecosystem is a multifaceted endeavor that demands meticulous attention to performance, security, and interoperability. By leveraging the strengths of Java and adhering to best practices, developers can build resilient, high-performance DeFi APIs that form the backbone of innovative decentralized financial applications.
In conclusion, the convergence of Java's robust features and the dynamic requirements of DeFi presents a compelling opportunity for developers to shape the future of decentralized finance through optimized API development.
By implementing these best practices and leveraging the power of Java, the potential for creating scalable, secure, and interoperable DeFi APIs is within reach. The evolution of DeFi relies on a solid foundation of well-optimized APIs, and Java stands as a formidable ally in this transformative journey.