Maximizing MapReduce Efficiency for Beginners
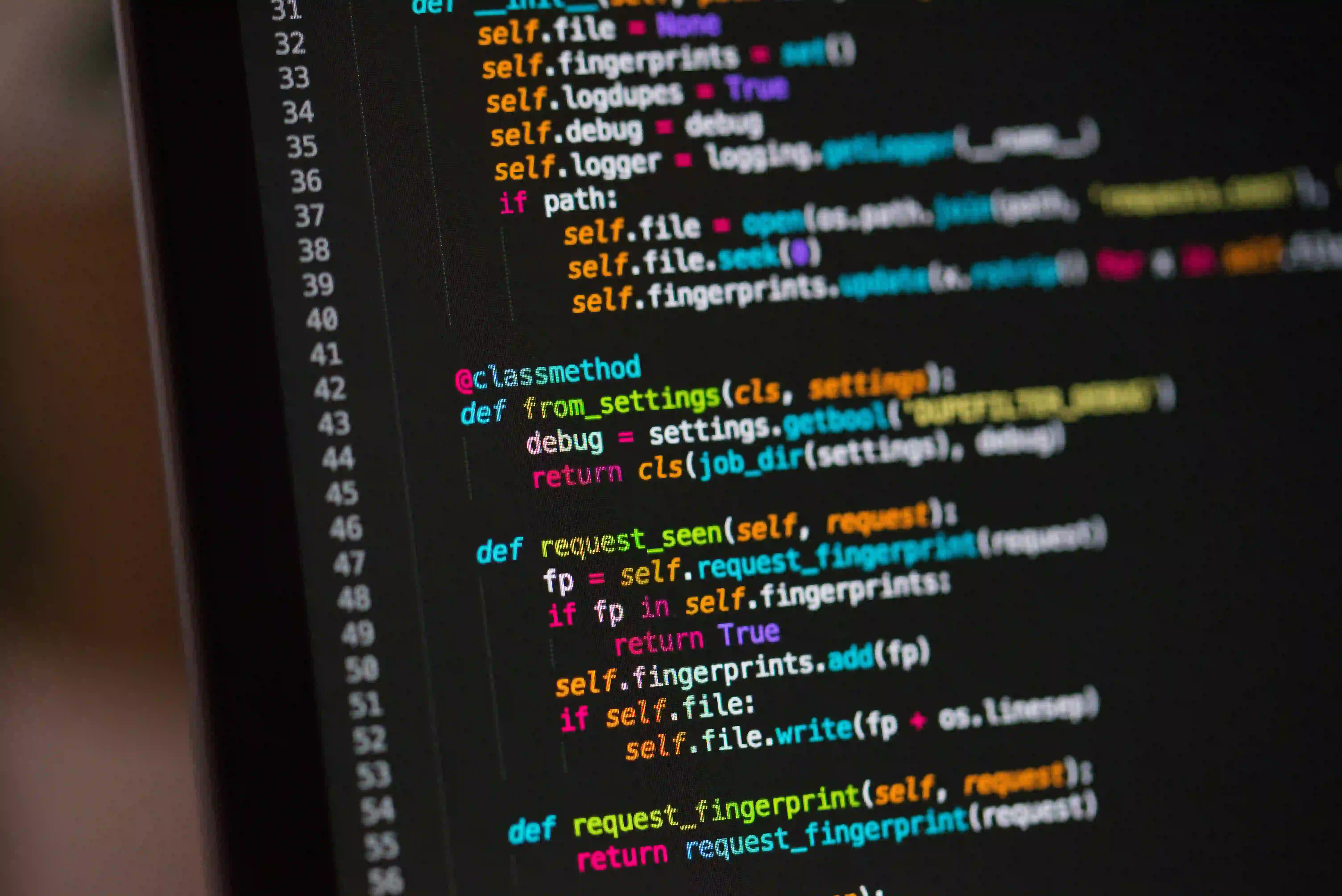
Maximizing MapReduce Efficiency for Beginners
When it comes to processing large-scale data sets, MapReduce has proven to be a powerful and popular tool. However, optimizing the efficiency of MapReduce jobs can be a complex task, especially for those new to the field. In this blog post, we'll delve into some essential tips and best practices to help beginners maximize the efficiency of their MapReduce jobs.
Understanding MapReduce Efficiency
MapReduce is a programming model and processing technique used for processing and generating large data sets. It divides the processing into two phases: the Map phase, where input data is divided into smaller chunks and processed in parallel, and the Reduce phase, where the results from the Map phase are aggregated and processed to produce the final output.
Efficiency in the context of MapReduce refers to the optimal utilization of computing resources, reduction of job execution time, and minimizing resource wastage. Achieving MapReduce efficiency involves factors such as data locality, task parallelism, and resource allocation.
Tip 1: Utilize Combiners
Combiners are mini reducers that perform a local reduction of the intermediate map output. Integrating combiners into your MapReduce job can significantly reduce the amount of data shuffled across the network during the Reduce phase. This optimization not only saves network bandwidth but also minimizes the load on the reducers, leading to faster job completion.
Code Example:
// Adding a combiner in your MapReduce job
job.setCombinerClass(YourCombinerClass.class);
Why: By using combiners, you can aggregate the intermediate outputs on each mapper node before transferring the data to the reducer. This reduces the volume of data shuffled, leading to improved efficiency.
Tip 2: Partitioning for Data Locality
Data locality plays a crucial role in the efficiency of MapReduce jobs. When input data is processed where it resides, it minimizes data transfer across the network, reducing latency and enhancing efficiency. Properly partitioning your data can optimize data locality, ensuring that related data is processed together on the same node where it resides.
Why: Partitioning your data optimally ensures that the Map tasks operate on data residing on the same node, thus minimizing data transfer and improving overall job efficiency.
Tip 3: Configuring Input Formats and Compression
Choosing the appropriate input format and compression codec can have a substantial impact on the efficiency of your MapReduce job. Selecting the right input format (e.g., TextInputFormat, KeyValueInputFormat) and compression codec (e.g., Snappy, Gzip) based on the nature of your input data can result in faster data processing and reduced storage requirements.
Why: Using suitable input formats and compression codecs can significantly improve the speed of data processing while reducing storage overhead.
Tip 4: Speculative Execution
Speculative Execution is a feature in Hadoop that allows tasks to be executed on multiple nodes simultaneously, with the first to complete being accepted and the others killed. Enabling speculative execution can guard against potential stragglers, where a slow node delays the overall job completion.
Code Example:
<!-- Enabling speculative execution in Hadoop configuration -->
<property>
<name>mapreduce.map.tasks.speculative.execution</name>
<value>true</value>
</property>
<property>
<name>mapreduce.reduce.tasks.speculative.execution</name>
<value>true</value>
</property>
Why: Speculative Execution can prevent delays caused by slow-running tasks, improving the overall completion time of MapReduce jobs.
Tip 5: Proper Configuration and Tuning
Hadoop and MapReduce offer a plethora of configuration parameters that can be tuned to optimize job performance. Parameters related to heap size, buffer size, number of mappers and reducers, and speculative execution thresholds can be adjusted to suit the specific characteristics of your job and cluster.
Why: Proper configuration and tuning of Hadoop and MapReduce parameters are essential in maximizing job efficiency by aligning the cluster resources with the workload demands.
Final Thoughts
Maximizing the efficiency of MapReduce jobs requires a deep understanding of various optimization techniques and best practices. By incorporating the aforementioned tips, newcomers to MapReduce can start their journey towards building and executing efficient data processing jobs.
Remember, achieving optimal MapReduce efficiency is an iterative process that involves experimentation, monitoring, and refining the job execution based on the characteristics of the data and the cluster. With these foundational concepts and practices at hand, diving deeper into the world of MapReduce optimization becomes more accessible, empowering beginners to harness the full potential of this powerful data processing paradigm.
Stay tuned for more in-depth discussions and practical examples on mastering MapReduce efficiency!
In this blog post, you learned essential tips for optimizing MapReduce efficiency, including utilizing combiners, partitioning for data locality, configuring input formats and compression, enabling speculative execution, and proper configuration and tuning. Optimizing MapReduce efficiency involves fine-tuning various aspects of the job to maximize resource utilization and reduce job execution time. With a solid understanding of these optimization techniques, beginners can unleash the full potential of MapReduce in processing large-scale data sets. If you're interested in diving deeper into the world of MapReduce, be sure to check out this comprehensive guide for further insights and practical examples.