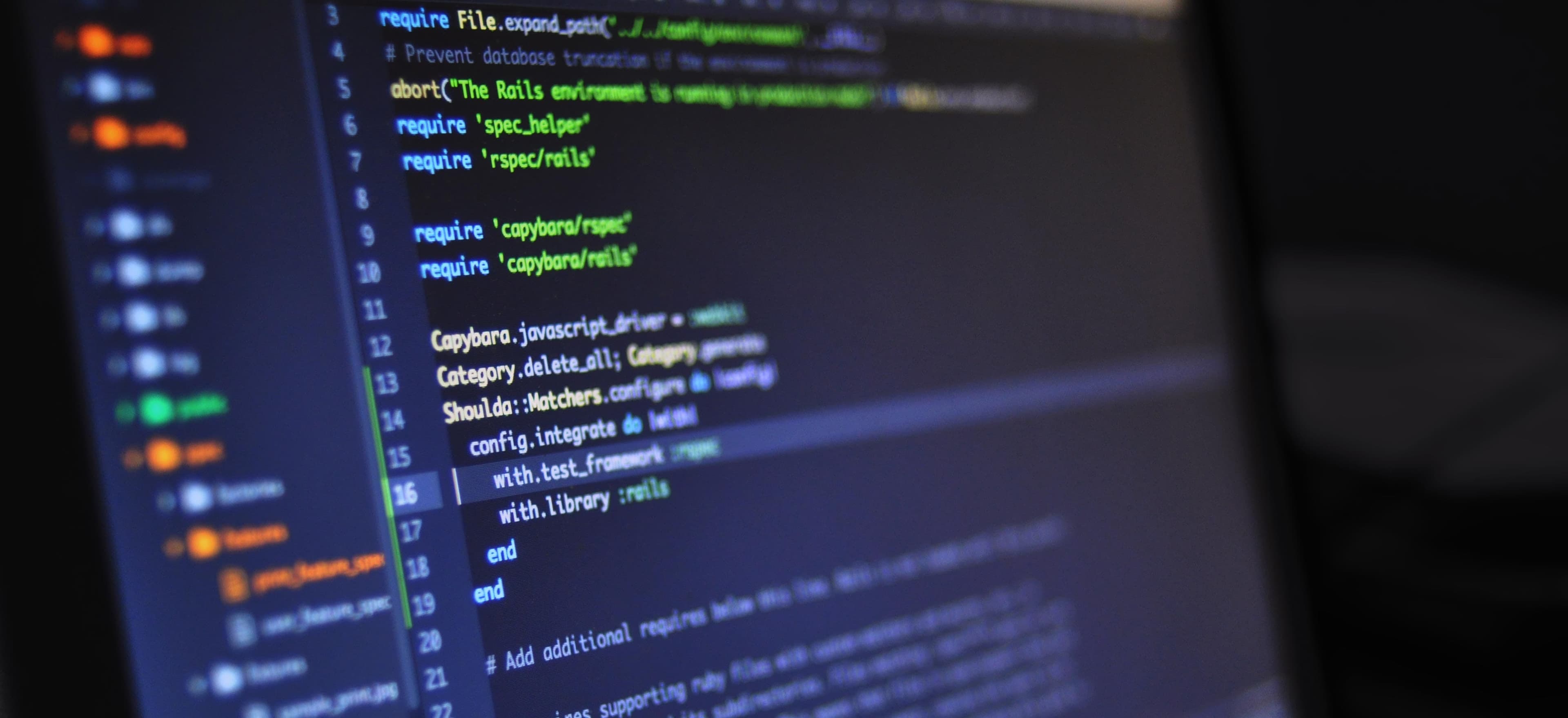
- Published on
Migrating Legacy OAuth 2 in Spring Boot 2
When maintaining a Spring Boot application, there often comes a time when you need to update its dependencies to keep it secure and up-to-date. If your application relies on OAuth 2 for authentication and authorization, moving from a legacy version to the latest one is crucial for security and compatibility reasons. In this guide, we will walk through the process of migrating legacy OAuth 2 in a Spring Boot 2 application.
Understanding OAuth 2
Before delving into the migration process, it's essential to have a solid understanding of OAuth 2. OAuth 2 is an industry-standard protocol for authorization, enabling third-party applications to obtain limited access to a user's HTTP service. It allows users to grant external applications access to their resources without exposing their credentials.
In a Spring Boot application, OAuth 2 is often implemented using Spring Security, which provides comprehensive security features for Java applications.
Assessing the Current Implementation
The first step in migrating from legacy OAuth 2 is to understand the current implementation within the Spring Boot application. Review the existing OAuth 2 configuration and identify any specific dependencies on legacy versions of Spring Security and OAuth 2 library.
It's crucial to analyze how the current implementation interacts with your application's authentication and authorization flows. This assessment will provide a clear picture of the potential impact of the migration.
Updating Dependencies
Once the current implementation is well understood, the next step involves updating the relevant dependencies and libraries to the latest versions. In your pom.xml
(Maven) or build.gradle
(Gradle), identify the versions of Spring Security and OAuth 2 libraries and update them to the latest stable releases.
For example, in a Maven pom.xml
, you can update the dependencies as follows:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
<version>2.5.4</version>
</dependency>
Similarly, update any other Spring Security and OAuth 2 dependencies to their latest versions.
Refactoring Configuration
With the updated dependencies in place, the next step is to refactor the OAuth 2 configuration to align with the latest practices and conventions.
In Spring Boot 2, the @EnableOAuth2Sso
annotation has been replaced by @EnableOAuth2Client
and @EnableOAuth2Resource
. Before making this change, review the existing OAuth 2 client configuration and modify it accordingly. For example:
@Configuration
@EnableOAuth2Client
public class OAuth2ClientConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/login**").permitAll()
.anyRequest().authenticated();
}
}
By updating the configuration to use @EnableOAuth2Client
, the application adapts to the latest OAuth 2 features and extensions provided by Spring Security.
Testing and Validation
After refactoring the OAuth 2 configuration, it's imperative to thoroughly test the application to ensure that the migration has been successful. Verify that the authentication and authorization flows function as expected and that any customizations or extensions to the OAuth 2 implementation remain intact.
It's advisable to perform both unit tests and integration tests to cover all aspects of the OAuth 2 functionality within the application.
Handling Breaking Changes
During the migration process, it's common to encounter breaking changes between the legacy and updated versions of OAuth 2. One such change could be the introduction of new configuration properties or deprecation of existing ones.
Carefully review the release notes and documentation for the updated versions of OAuth 2 and Spring Security to identify any breaking changes. Make the necessary adjustments in the application's codebase to accommodate these changes.
Communicating with External Services
In many cases, Spring Boot applications that utilize OAuth 2 interact with external services, such as identity providers or API gateways. When migrating from legacy OAuth 2, it's crucial to communicate with the owners or maintainers of these external services to ensure a seamless transition.
Notify them about the upcoming migration and inquire about any changes or updates required on their end to support the latest OAuth 2 enhancements.
Final Considerations
Migrating from legacy OAuth 2 in a Spring Boot 2 application is a critical task that ensures the application remains secure and compatible with the latest industry standards. By understanding the current implementation, updating dependencies, refactoring configuration, testing thoroughly, handling breaking changes, and communicating with external services, you can successfully complete this migration process.
Remember, keeping your application's dependencies up-to-date not only enhances security but also provides access to the latest features and improvements offered by OAuth 2 and Spring Security.
Ensure to document the migration process and keep a comprehensive record of the changes made to the OAuth 2 configuration and dependencies for future reference.
For further reading on OAuth 2 and Spring Security, refer to the OAuth 2.0 RFC and the Spring Security documentation.
Happy coding!