Mastering Implicits in Scala: Common Pitfalls Explained
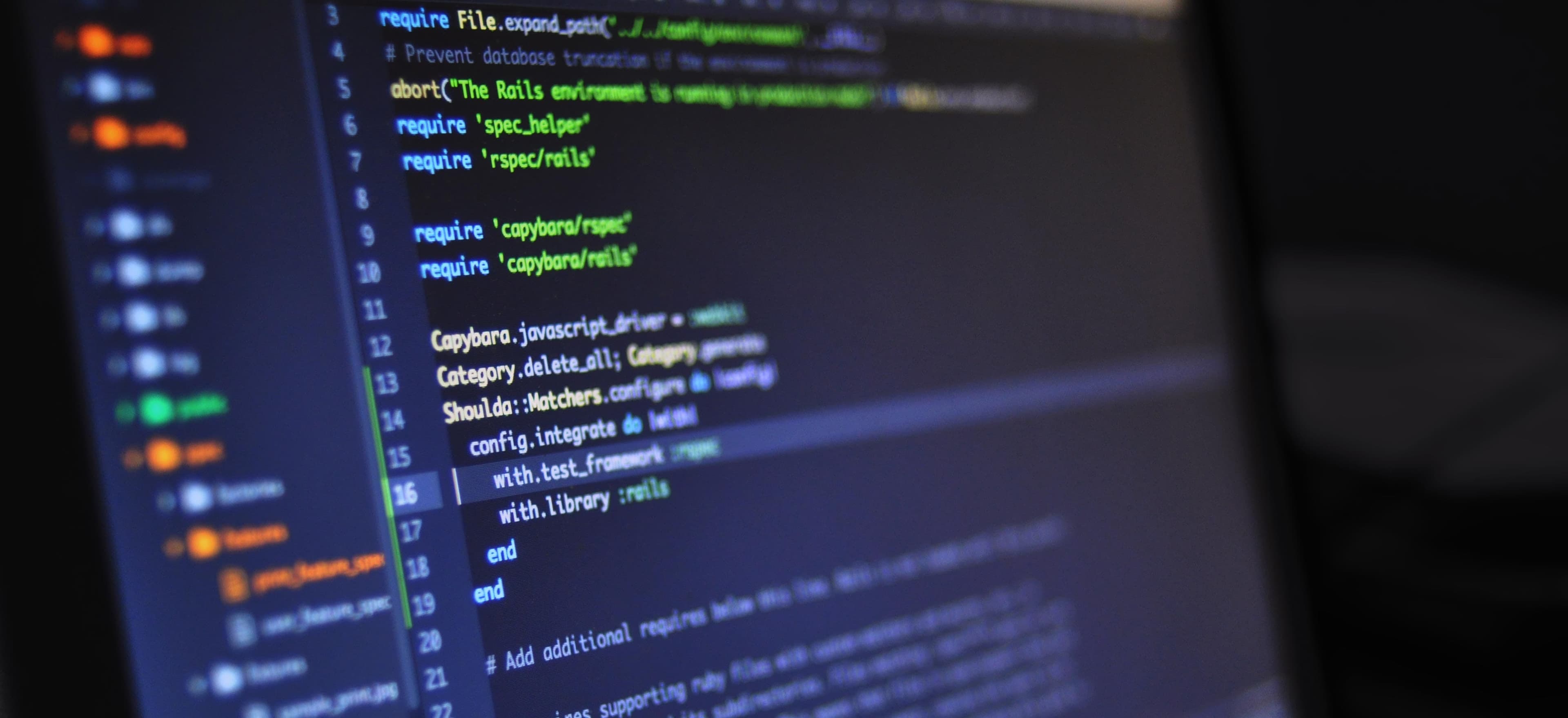
- Published on
Mastering Implicits in Scala: Common Pitfalls Explained
Scala's implicit
feature is one of the language's most powerful paradigms, allowing developers to write cleaner and more concise code. However, it also introduces complexity that can lead to misunderstandings or errors if not properly managed. This blog post aims to demystify implicits in Scala, highlighting common pitfalls and offering clear solutions.
What Are Implicits?
Implicits in Scala can be categorized into two main types: implicit parameters and implicit conversions.
- Implicit Parameters allow you to pass parameters to functions or methods without explicitly specifying them at every call site.
- Implicit Conversions provide a way to automatically convert one type to another when needed.
Here’s a quick example to illustrate implicit parameters:
case class User(name: String)
def greet(implicit user: User): String = {
s"Hello, ${user.name}!"
}
// Using an implicit value
implicit val defaultUser: User = User("Alice")
println(greet) // Output: Hello, Alice!
Why Use Implicits?
Using implicits can simplify your code significantly. It can reduce boilerplate and improve readability. However, it is crucial to wield this power carefully. Below, we discuss common pitfalls and how to avoid them.
Common Pitfall 1: Overusing Implicits
Using implicits can lead to implicit behavior that is hard to track. When a method relies on implicit parameters, it can become challenging to determine which values are being passed. This can obscure cause-and-effect relationships in code.
Example of Overuse
Consider a scenario where you have too many implicits:
implicit val user1: User = User("Bob")
implicit val user2: User = User("Charlie")
def greet(implicit user: User): String = {
s"Hello, ${user.name}!"
}
// Calling greet with multiple implicits in scope leads to ambiguity
println(greet) // Which user is being used?
Best Practice
Limit the use of implicits. Always favor clarity over brevity. If you ever find yourself maintaining a collection of many implicits, consider refactoring them into more explicit parameters. This enhances maintainability.
Common Pitfall 2: Implicit Not Found
A frequent issue developers face is the "implicit not found" error. This occurs when Scala cannot find a suitable implicit value for a parameter.
Example of Implicit Not Found
def greet(implicit user: User): String = {
s"Hello, ${user.name}!"
}
// No implicit value in scope
println(greet) // Error: could not find implicit value for parameter user
Best Practice
Ensure that you have the required implicits in scope. If you are designing a method that relies on implicits, document the expected implicit parameters clearly, or use context bounds to indicate that certain implicits are required.
Common Pitfall 3: Implicit Conversions
Implicit conversions can also be a double-edged sword. They can introduce hidden behavior that may not be immediately obvious.
Example of Implicit Conversion
implicit def intToString(x: Int): String = x.toString
val str: String = 42 // This converts 42 to "42"
Solution
Use implicit conversions sparingly. Prefer extensions or case classes to wrap types instead. This prevents unexpected behavior and improves type safety.
case class Wrapper(value: Int)
implicit class WrapperOps(wrapper: Wrapper) {
def toStringValue: String = wrapper.value.toString
}
val wrapped = Wrapper(42)
println(wrapped.toStringValue) // Output: "42"
Common Pitfall 4: Implicit Ambiguity
When there are multiple implicits in scope that could satisfy a parameter’s type, Scala will throw an ambiguity error.
Example of Implicit Ambiguity
case class User(name: String)
implicit val user1: User = User("Alice")
implicit val user2: User = User("Bob")
// Which user should be used?
def greet(implicit user: User): String = {
s"Hello, ${user.name}!"
}
// This will cause an ambiguity error
println(greet)
Best Practice
To resolve ambiguity:
- Limit the scope of implicits. Ensure that only one implicit value of a given type is in scope.
- Use
import
statements judiciously. Avoid bringing implicits from too many sources.
You can also use explicit values when calling a method to resolve ambiguity.
println(greet(user1)) // Output: Hello, Alice!
println(greet(user2)) // Output: Hello, Bob!
A Final Look: Use Implicits Wisely
Scala implicits are a powerful tool that can enhance your code when used correctly. However, their overuse or misuse can lead to ambiguous, hard-to-maintain code. Remember the following best practices:
- Use implicits judiciously to avoid complicating your code.
- Make sure you have the needed implicits in scope before using them.
- Prefer explicitness over implicitness when unsure.
For more on Scala's implicits, consider checking out the official Scala documentation and the Scala Style Guide, which emphasize best practices around language features like implicits.
By mastering implicits, you can harness their power while steering clear of potential pitfalls, leading to cleaner, more maintainable Scala applications. Happy coding!