The Importance of Task Execution Order
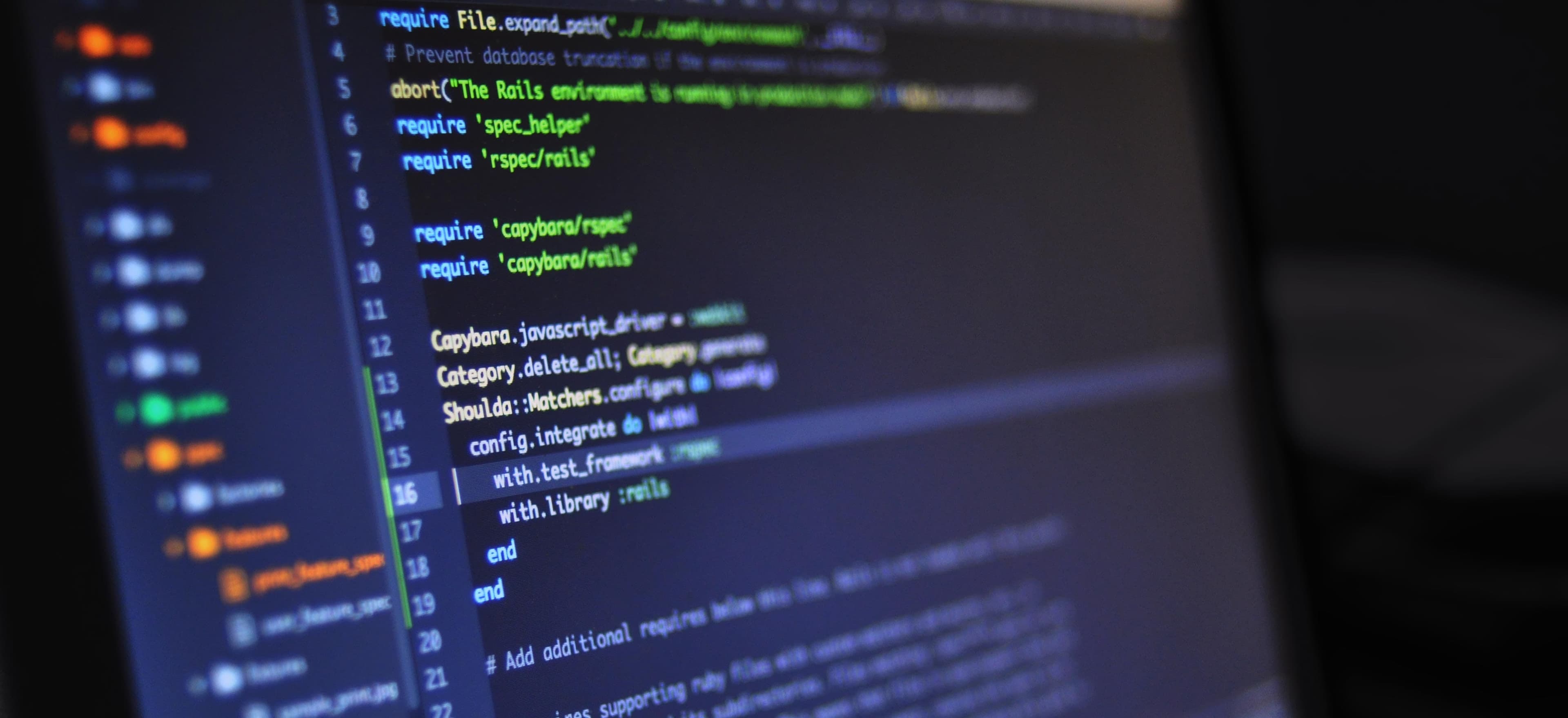
- Published on
The Importance of Task Execution Order in Java
In the world of programming, the order in which tasks are executed can have a significant impact on the outcome of a program. This is especially true in Java, where the execution order of tasks can determine whether a program runs smoothly or encounters issues. Understanding task execution order is crucial for Java developers to write efficient, predictable, and reliable code.
Why Task Execution Order Matters
In Java, tasks can be executed in a variety of ways, including sequential execution, concurrent execution, and parallel execution. The order in which tasks are executed can be influenced by factors such as thread scheduling, synchronization, and dependencies between tasks.
Consider a scenario where a program needs to fetch data from a remote server, process the data, and then update the user interface. If these tasks are not executed in the correct order, the program may experience unexpected behavior, such as displaying incomplete or outdated information to the user.
Understanding Sequential Execution
Sequential execution refers to the default behavior of Java programs, where tasks are executed one after the other in a predefined order. This is often suitable for simple, linear workflows where each task depends on the completion of the previous one.
public class SequentialExecutionExample {
public static void main(String[] args) {
fetchDataFromServer();
processData();
updateUI();
}
private static void fetchDataFromServer() {
// Implementation to fetch data from server
}
private static void processData() {
// Implementation to process the data
}
private static void updateUI() {
// Implementation to update the user interface
}
}
In this example, fetchDataFromServer()
must complete before processData()
can begin, and processData()
must finish before updateUI()
is called.
Introducing Concurrent Execution
Concurrent execution involves running multiple tasks simultaneously, which can improve program performance by utilizing available resources more efficiently. However, it also introduces challenges such as thread safety and synchronization.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ConcurrentExecutionExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.submit(ConcurrentExecutionExample::fetchDataFromServer);
executor.submit(ConcurrentExecutionExample::processData);
executor.submit(ConcurrentExecutionExample::updateUI);
executor.shutdown();
}
private static void fetchDataFromServer() {
// Implementation to fetch data from server
}
private static void processData() {
// Implementation to process the data
}
private static void updateUI() {
// Implementation to update the user interface
}
}
In this example, the ExecutorService
allows tasks to be submitted for concurrent execution. The order in which the tasks are executed is not guaranteed, and they may run simultaneously depending on the available threads in the thread pool.
Dealing with Dependencies and Order
When dealing with tasks that have dependencies or require a specific execution order, Java provides mechanisms for managing these scenarios. One such mechanism is the use of CompletableFuture
to orchestrate the execution order of asynchronous tasks.
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<Void> fetchData = CompletableFuture.runAsync(CompletableFutureExample::fetchDataFromServer);
CompletableFuture<Void> processData = fetchData.thenRun(CompletableFutureExample::processData);
CompletableFuture<Void> updateUI = processData.thenRun(CompletableFutureExample::updateUI);
updateUI.join(); // Wait for all tasks to complete
}
private static void fetchDataFromServer() {
// Implementation to fetch data from server
}
private static void processData() {
// Implementation to process the data
}
private static void updateUI() {
// Implementation to update the user interface
}
}
In this example, CompletableFuture
allows us to define dependencies between tasks using methods such as thenRun
. This ensures that processData()
will not execute until fetchDataFromServer()
has completed, and updateUI()
will wait for processData()
to finish.
Bringing It All Together
In Java programming, understanding and managing task execution order is crucial for writing efficient, predictable, and reliable code. Whether dealing with sequential, concurrent, or asynchronous tasks, developers must consider the dependencies and order of execution to ensure the correct behavior of their programs.
By utilizing features such as ExecutorService
for concurrent execution and CompletableFuture
for orchestrating asynchronous tasks, Java developers can control the order in which tasks are executed, leading to more robust and responsive applications.
In conclusion, mastering task execution order empowers Java developers to create performant and dependable software, where the correct sequencing of tasks ensures a smooth user experience and efficient resource utilization.
For further reading on task execution order in Java, check out the official Java documentation.