Optimizing Battery Life: Addressing Android Broadcast Receiver Impact
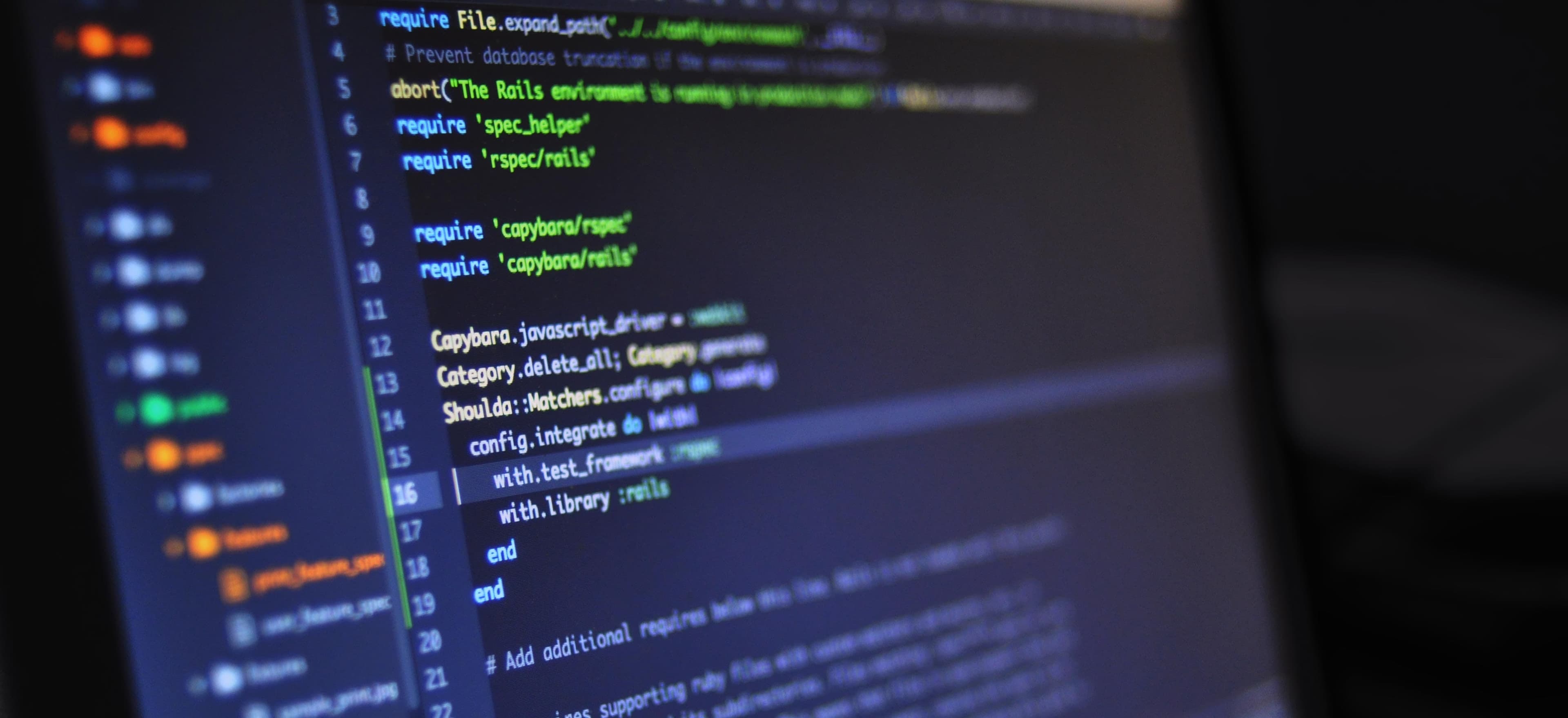
- Published on
Analyzing Battery Drain in Android: Understanding the Impact of Broadcast Receivers
Being a crucial aspect of the Android operating system, broadcast receivers serve as the primary component for enabling various applications to respond to system-wide broadcast announcements, including the arrival of a text message, screen turning off or on, device rebooting, and more. Despite their significance, broadcast receivers can have a substantial impact on the device's battery life if not managed efficiently.
In this blog post, we will delve into the impact of broadcast receivers on battery life in Android devices and explore best practices to optimize their usage. We will discuss key strategies for mitigating battery drain caused by broadcast receivers and examine efficient ways to handle broadcasts to ensure minimal impact on battery consumption.
Understanding the Impact
Broadcast receivers play a pivotal role in event-driven communication within the Android system. However, if not handled carefully, they can lead to battery drain due to excessive resource consumption, such as CPU cycles, network activity, and wake locks.
The impact stems from the fact that when a broadcast is sent, all registered broadcast receivers are awakened, leading to potential performance bottlenecks and increased power usage, particularly when multiple apps are frequently receiving broadcasts.
Best Practices for Optimizing Battery Life
To mitigate the impact of broadcast receivers on battery life, it is crucial to follow best practices and implement efficient strategies. Let’s delve into some effective approaches to address and optimize battery consumption caused by broadcast receivers in Android applications.
1. Prioritize Intent Filters
When registering a broadcast receiver, specifying the appropriate intent filters is essential. By precisely defining the types of broadcasts that the receiver should handle, unnecessary wake-ups can be minimized, thereby reducing the battery impact. Utilize explicit intent filters instead of implicit ones whenever possible, as this allows for targeted handling of specific broadcasts.
// Example of registering a broadcast receiver with explicit intent filter
IntentFilter filter = new IntentFilter("com.example.CUSTOM_ACTION");
registerReceiver(receiver, filter);
2. Unregister Receivers When Inactive
It is critical to unregister broadcast receivers when they are inactive or no longer required to receive broadcasts. Failing to do so can lead to unnecessary resource consumption and prolonged execution, adversely affecting the device's battery life. Prioritize unregistering receivers within the appropriate lifecycle methods of the component, such as onPause()
or onStop()
, to ensure timely cleanup.
// Example of unregistering a broadcast receiver
@Override
protected void onStop() {
super.onStop();
unregisterReceiver(receiver);
}
3. Use Local Broadcast Manager
In scenarios where broadcasts are intended for intra-application communication, leveraging the Local Broadcast Manager provided by the Android Support Library is advantageous. Unlike global broadcasts, local broadcasts are not exposed to other applications, resulting in reduced system-wide overhead and improved battery efficiency.
// Example of sending a local broadcast using LocalBroadcastManager
LocalBroadcastManager.getInstance(context).sendBroadcast(intent);
4. Minimize Work in onReceive()
The onReceive()
method of a broadcast receiver should execute minimal work to swiftly process the broadcast and release resources promptly. Long-running tasks or operations with significant computational overhead should be delegated to background threads or services to avoid blocking the main thread and causing excessive battery drain.
// Example of delegating long-running task to a background thread
@Override
public void onReceive(Context context, Intent intent) {
executor.execute(() -> processIntent(intent));
}
5. Opt for Manifest-Registered Receivers When Appropriate
When a broadcast receiver needs to respond to system-wide events, manifest registration as opposed to dynamic registration can be more suitable. Manifest-registered receivers allow for the system to manage the lifecycle of the receiver, ensuring that it is active only when needed and not lingering in the background, consuming resources unnecessarily.
<!-- Example of manifest registration of a broadcast receiver -->
<receiver android:name=".MyReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED"/>
</intent-filter>
</receiver>
To Wrap Things Up
In conclusion, the impact of broadcast receivers on battery life in Android devices cannot be overlooked, and optimizing their usage is imperative for enhancing the overall user experience. By prioritizing intent filters, efficiently managing receiver registration and unregistration, and judiciously handling broadcasts, developers can significantly minimize the battery drain associated with broadcast receivers.
Implementing these best practices not only contributes to improved battery efficiency but also fosters a smoother, more responsive user experience, ultimately leading to greater user satisfaction with the application.
Optimizing battery life through effective broadcast receiver management is an integral aspect of Android app development, and adhering to these best practices will undoubtedly result in more resource-efficient applications, benefiting both users and developers alike.
For further insights into Android broadcast receivers and battery optimization, refer to the Android Developer Guide and Optimizing Battery Life documentation.