Overcoming Technical Debt: Key Strategies
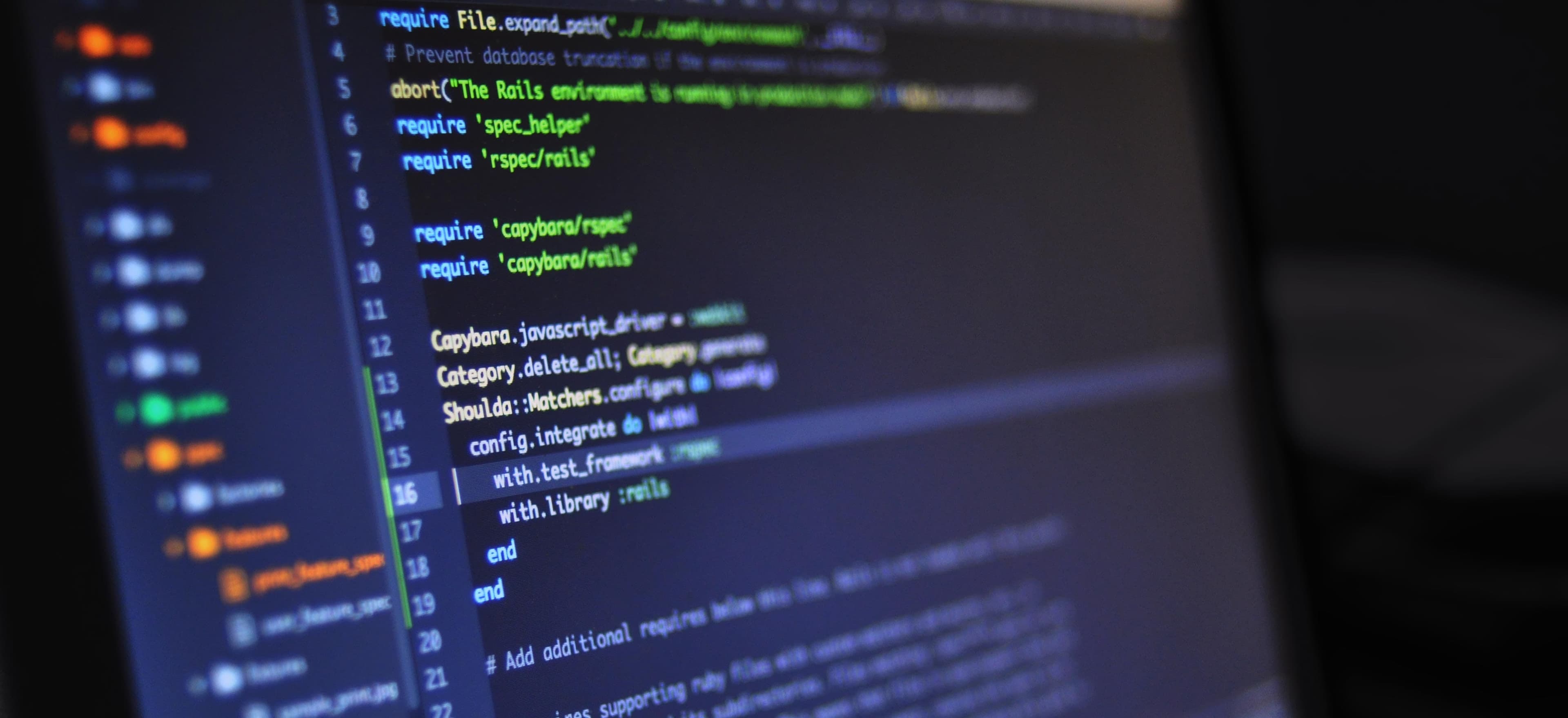
- Published on
Overcoming Technical Debt: Key Strategies
Technical debt is a common challenge in software development that can hinder productivity and innovation. As a Java developer, it's essential to understand how to identify and address technical debt effectively. In this article, we'll explore key strategies for overcoming technical debt in Java projects, including refactoring, automated testing, and prioritization.
Refactoring for Debt Reduction
Refactoring is the process of restructuring existing code without changing its external behavior to improve readability, maintainability, and extensibility. In the context of technical debt, refactoring plays a crucial role in reducing complexity and eliminating code smells.
Example:
// Before refactoring
public void calculateTotalPrice(List<CartItem> items) {
double total = 0;
for (int i = 0; i < items.size(); i++) {
total += items.get(i).getPrice() * items.get(i).getQuantity();
}
logger.log("Total price calculated: " + total);
}
// After refactoring
public double calculateTotalPrice(List<CartItem> items) {
return items.stream()
.mapToDouble(item -> item.getPrice() * item.getQuantity())
.sum();
}
In the example above, refactoring the calculateTotalPrice
method using Java 8 streams simplifies the code and improves its readability.
Automated Testing for Confidence
Automated testing is a fundamental practice for ensuring code quality and preventing regression. It provides confidence in making changes to the codebase while maintaining its correctness.
Example:
@Test
public void calculateTotalPrice_ShouldReturnCorrectTotal() {
List<CartItem> items = Arrays.asList(new CartItem("A", 10, 2), new CartItem("B", 5, 4));
double expectedTotal = 10*2 + 5*4;
double actualTotal = cartCalculator.calculateTotalPrice(items);
assertEquals(expectedTotal, actualTotal, 0.001);
}
The example showcases a unit test for the calculateTotalPrice
method, ensuring that it returns the expected total for a given list of items.
Prioritization for Effective Debt Management
Not all technical debt is created equal. It's essential to prioritize addressing the most critical areas of debt that impact the system's stability, security, and performance.
Example:
// High-priority technical debt
// Inefficient algorithm leading to performance bottlenecks
// Medium-priority technical debt
// Code duplication across multiple modules
// Low-priority technical debt
// Minor code style inconsistencies
By categorizing technical debt based on its impact, developers can focus on resolving high-priority issues first, ensuring that the most critical areas are addressed promptly.
Leveraging Tools for Debt Analysis
Several tools can assist in identifying and analyzing technical debt within a codebase. For Java projects, tools like SonarQube and FindBugs provide valuable insights into areas of improvement.
By integrating these tools into the continuous integration (CI) process, developers can receive feedback on technical debt metrics, code smells, and potential bugs, enabling proactive debt management.
Closing the Chapter
Overcoming technical debt requires a proactive and systematic approach. By leveraging refactoring, automated testing, prioritization, and tools for debt analysis, Java developers can effectively manage and reduce technical debt in their projects, fostering a healthier and more sustainable codebase. Embracing these strategies will lead to improved developer productivity, faster time-to-market, and better overall software quality.
Addressing technical debt is not just a responsibility; it is an opportunity to optimize the codebase and lay a strong foundation for future development. With these strategies in place, Java developers can navigate the complexities of technical debt with confidence and efficiency.
Checkout our other articles