The Power of Java Records in Modern Development
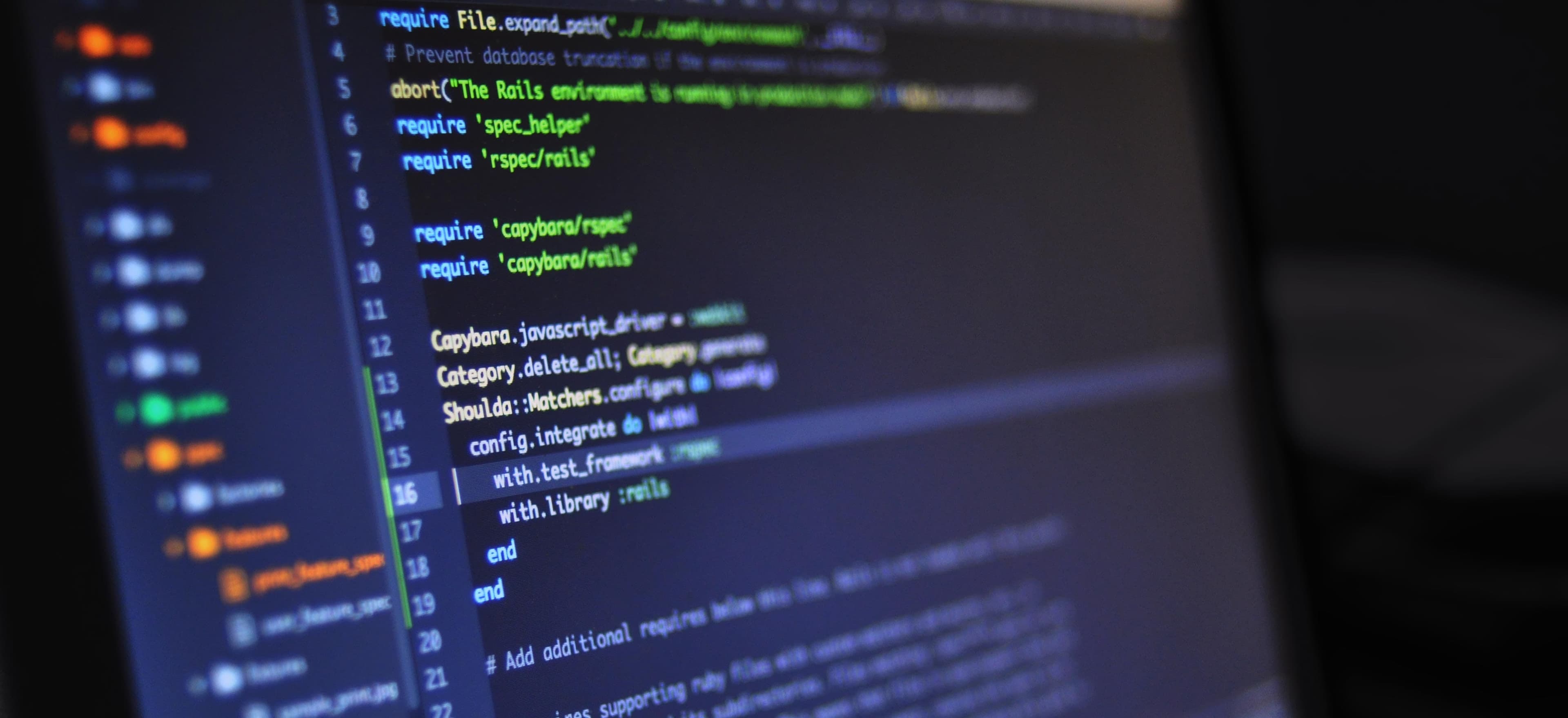
- Published on
In today's fast-paced world of software development, Java remains a stalwart and versatile language. With the release of Java 14, the introduction of records has opened up new possibilities for writing concise and expressive code. In this post, we will delve into the power of Java records and how they can revolutionize modern development practices.
What are Java Records?
Java records are a new feature introduced in Java 14 as a preview feature and finally made permanent in Java 16. They provide a concise way to declare classes that are transparent holders for immutable data. Records are a form of nominal tuples, which means they group related data together.
Declaring a Java Record
To declare a record in Java, you simply use the 'record' keyword, followed by the name of the record and its components. Each component represents a field of the record.
public record Point(int x, int y) {}
In this example, we've declared a record named 'Point' with two components, 'x' and 'y', both of type 'int'. This single line of code replaces the entire class definition, constructor, accessors, and equals/hashCode/toString methods that are typically required.
Why Use Java Records?
- Conciseness: Java records reduce boilerplate code and make the codebase more concise and readable.
- Immutability: Records are immutable by default, making it easier to reason about state and avoid accidental mutations.
- Built-in Methods: Records provide built-in methods like equals(), hashCode(), and toString(), promoting consistency and reducing errors in implementing these methods manually.
- Interoperability: Records seamlessly work with existing libraries and frameworks, enabling smooth integration into modern development ecosystems.
- Pattern Matching: Records enable pattern matching, which is a powerful feature for handling data in more sophisticated ways.
Accessing Components of a Record
To access the components of a record, you can use the accessor methods that are automatically generated by the compiler. For example, with the 'Point' record we declared earlier, you can access its components as follows:
Point point = new Point(3, 5);
int x = point.x();
int y = point.y();
System.out.println("Coordinates: (" + x + ", " + y + ")");
When to Use Java Records?
Java records are particularly valuable in scenarios where you need a simple container for data, such as representing tuples, coordinate points, or data transfer objects (DTOs). They are also useful in domain-driven design where you have plain data carrying a specific meaning.
Pattern Matching with Java Records
One of the most exciting features associated with Java records is pattern matching. Pattern matching allows for more sophisticated and concise code when handling data. The 'instanceof' operator and type cast can be clunky, error-prone, and repetitive when working with classes. Records, however, provide a clean and concise way to destructure and match data.
Consider the following example of pattern matching with a Java record:
void processShape(Shape shape) {
if (shape instanceof Circle circle) {
System.out.println("Circle with radius " + circle.radius());
} else if (shape instanceof Rectangle rectangle) {
System.out.println("Rectangle with width " + rectangle.width() + " and height " + rectangle.height());
} else {
System.out.println("Unknown shape");
}
}
In this example, the 'instanceof' operator is used to check if the 'shape' is an instance of a 'Circle' or 'Rectangle' record. If it is, pattern variables 'circle' or 'rectangle' are introduced to capture the components of the record. This approach is more concise and safer compared to traditional type casting.
Compatibility and Adoption
Since Java 16, records are a standard feature and fully supported in the Java language. They are also supported in popular IDEs like IntelliJ IDEA, Eclipse, and NetBeans, making the adoption process seamless for developers.
The Closing Argument
Java records are a powerful addition to the language that greatly simplifies the code required for simple, immutable data carriers. They enable developers to write more concise, readable, and maintainable code while leveraging features like built-in methods and pattern matching. With their full integration into the Java language, records mark a significant milestone in modern Java development.
In summary, Java records:
- Provide a concise way to declare classes for immutable data
- Reduce boilerplate code and promote code readability
- Facilitate pattern matching for enhanced data handling
- Are fully supported in Java 16 and popular IDEs
In conclusion, Java records are a game-changer for modern Java development, and embracing them can lead to more efficient, maintainable, and expressive codebases. Embrace the power of Java records and elevate your development practices to new heights.
Checkout our other articles