Generating REST API CRUD Operations with Speedment: A How-to Guide
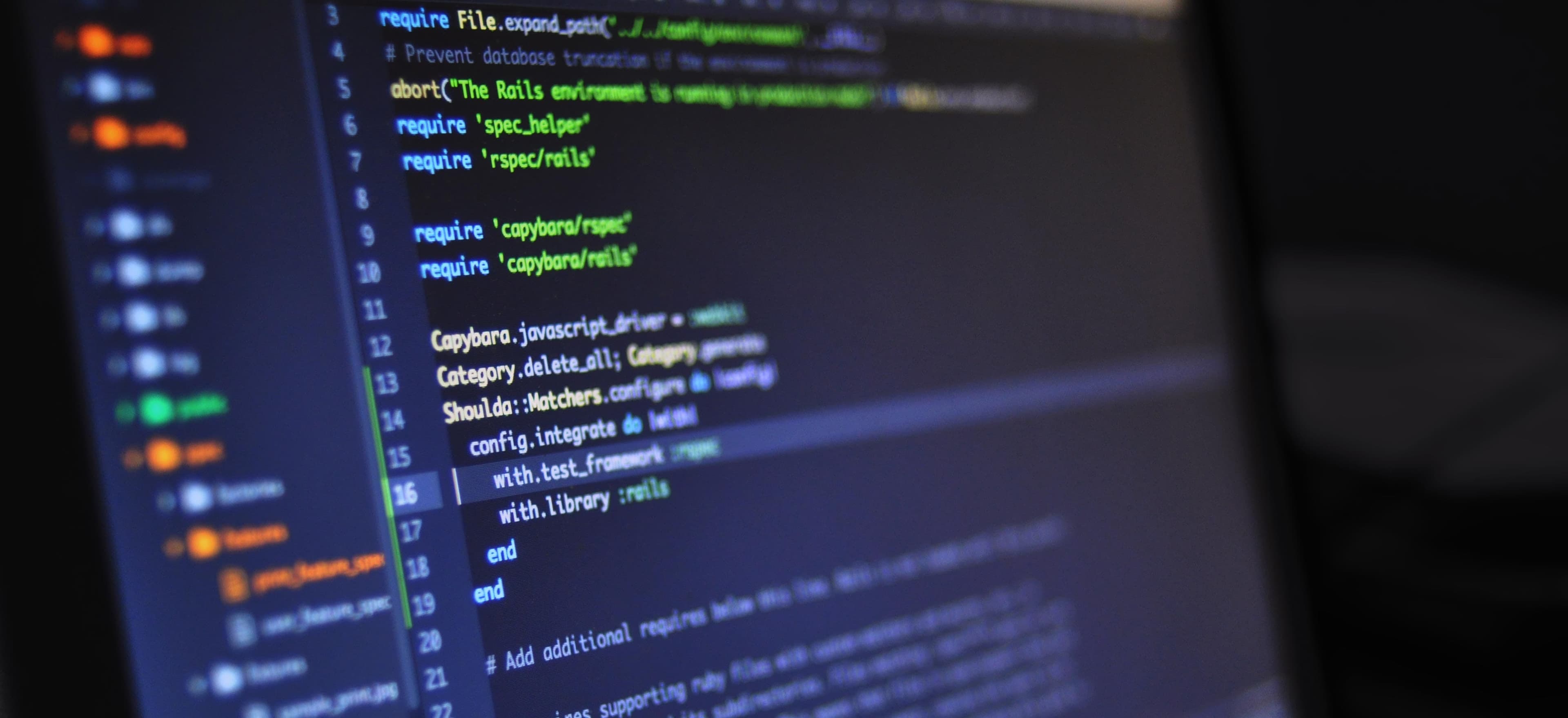
- Published on
Generating REST API CRUD Operations with Speedment: A How-to Guide
In the world of software development, building REST APIs and handling CRUD operations is a common task. Java, being one of the most popular programming languages, offers various tools and frameworks to simplify this process. One such tool is Speedment, which can significantly speed up the process of creating REST API CRUD operations. In this article, we will explore how to generate REST API CRUD operations using Speedment in a step-by-step manner.
What is Speedment?
Before delving into the specifics of generating REST API CRUD operations with Speedment, it's important to understand what Speedment is and how it can benefit Java developers.
Speedment is an open-source Java ORM (Object-Relational Mapping) tool that leverages the Java Stream API to provide a fluent and type-safe way to interact with databases. It allows developers to generate Java classes and interfaces directly from an existing database schema, eliminating the need to write boilerplate code for basic CRUD operations.
Now, let's move on to the step-by-step process of generating REST API CRUD operations using Speedment.
Step 1: Setting Up the Project
To get started with Speedment, you'll need to set up a new Java project. You can either create a new Maven or Gradle project or add Speedment as a dependency to an existing project.
Maven Dependency
Add the following dependency to your pom.xml
file:
<dependency>
<groupId=com.speedment</groupId.
<artifactId=<artifactId>
<version>3.2.10</version>
</dependency>
Gradle Dependency
Add the following dependency to your build.gradle
file:
dependencies {
implementation 'com.speedment:speedment:3.2.10'
}
Step 2: Database Configuration
After setting up the project, you need to configure Speedment to connect to your database. Speedment supports a variety of databases, including MySQL, PostgreSQL, and SQLite.
Configuration File
Create a configuration file (e.g., speedment.json
) in the root directory of your project. Here's an example of a basic Speedment configuration for a MySQL database:
{
"config" : {
"name" : "myDb"
},
"properties" : {
"dbms.name" : "mysql",
"dbms.host" : "localhost",
"dbms.port" : "3306",
"dbms.schema" : "my_schema",
"dbms.username" : "username",
"dbms.password" : "password"
}
}
Replace the placeholder values with your actual database configuration.
Step 3: Generating Speedment Code
Once the project is set up and the database is configured, you can use Speedment to generate Java code based on the database schema.
Speedment Tool
Speedment provides a command-line tool that can be used to generate code based on the database configuration. Execute the following command in the terminal:
java -jar speedment-tool-3.2.10.jar
Replace speedment-tool-3.2.10.jar
with the actual name of the Speedment tool JAR file.
Step 4: Implementing REST API Endpoints
With the generated Speedment code, you can now proceed to implement REST API endpoints for CRUD operations.
Example: Creating a REST Controller
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getAllUsers() {
return userRepository.findAll();
}
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
Optional<User> user = userRepository.findById(id);
return user.map(ResponseEntity::ok)
.orElse(ResponseEntity.notFound().build());
}
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
userRepository.save(user);
return ResponseEntity.status(HttpStatus.CREATED).body(user);
}
@PutMapping("/{id}")
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody User user) {
if (!userRepository.existsById(id)) {
return ResponseEntity.notFound().build();
}
user.setId(id);
userRepository.save(user);
return ResponseEntity.ok(user);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteUser(@PathVariable Long id) {
userRepository.deleteById(id);
return ResponseEntity.noContent().build();
}
}
In the above example, we have created a UserController
with endpoints for retrieving all users, retrieving a single user by ID, creating a new user, updating an existing user, and deleting a user. The UserRepository
is a Speedment-generated repository interface that provides methods for interacting with the User
entity.
Final Thoughts
In this guide, we've learned how to generate REST API CRUD operations using Speedment, an efficient ORM tool for Java. By leveraging Speedment, developers can quickly generate Java classes and interfaces based on an existing database schema, and seamlessly integrate them into RESTful APIs. This approach not only reduces the amount of boilerplate code but also ensures type safety and consistency with the database schema.
Integrating Speedment into your Java projects can streamline the process of building and maintaining RESTful APIs, ultimately improving productivity and code maintainability.
To learn more about Speedment, explore the official Speedment documentation.
Now that you have a solid understanding of generating REST API CRUD operations using Speedment, it's time to put this knowledge into practice and experience the benefits firsthand. Happy coding!