Troubleshooting Groovy Issue Tracker File System Integration
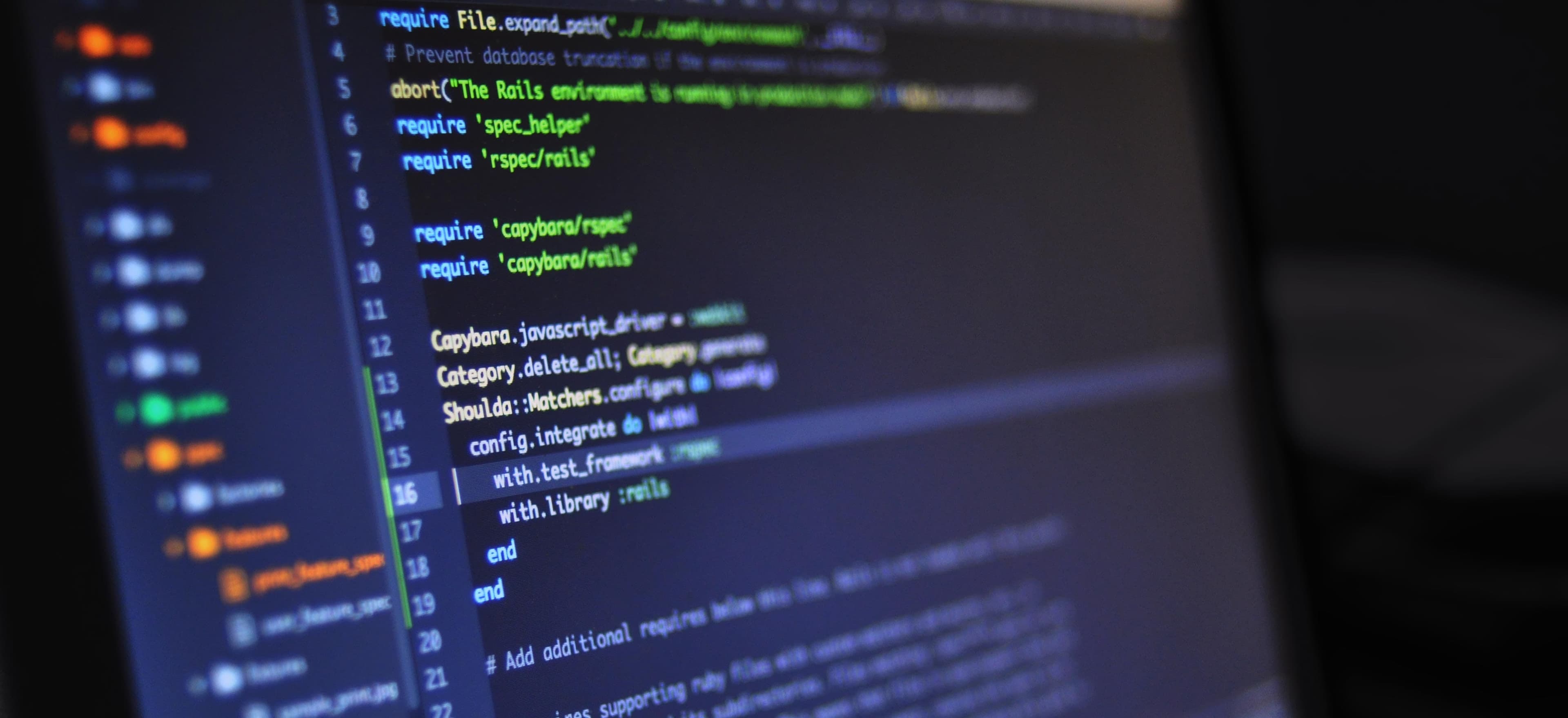
- Published on
Troubleshooting Groovy Issue Tracker File System Integration
In this blog post, we're going to dive into troubleshooting file system integration within a Groovy issue tracker application. We'll cover common issues, their possible causes, and how to resolve them using Java.
Problem 1: Unable to Read/Write Files
Possible Cause
One of the common issues in file system integration is the inability to read or write files. This can occur due to inadequate file permissions, incorrect file paths, or file locks.
Solution
Let's start by examining a simple Java code snippet that reads a file from the file system:
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class FileReader {
public static void main(String[] args) {
File file = new File("path_to_file.txt");
try {
String content = new String(Files.readAllBytes(Paths.get(file.toURI())));
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code snippet, we attempt to read the content of a file using Java's NIO package. If an exception is thrown, we print the stack trace to help identify the issue.
To write to a file, you can use a similar approach, replacing Files.readAllBytes
with Files.write
.
Problem 2: File System Path Resolution
Possible Cause
Incorrect file system paths can lead to issues in file system integration. This may involve using relative paths instead of absolute paths, resulting in files not being found or written to the expected location.
Solution
Java provides the Paths
class to help resolve file system paths. Let's consider an example to demonstrate the use of Paths
for resolving file paths:
import java.nio.file.Path;
import java.nio.file.Paths;
public class PathResolver {
public static void main(String[] args) {
Path path = Paths.get("src/main/resources/data.txt");
System.out.println(path.toAbsolutePath());
}
}
In this example, we create a Path
object using Paths.get
and then use toAbsolutePath
to get the absolute path. This can help in identifying and resolving path issues in file system integration.
Problem 3: File Locking
Possible Cause
File locking issues can arise when a file is already in use by another process or when a previous operation did not release the file lock properly.
Solution
Java provides a way to handle file locking using the FileChannel
class. Let's take a look at a code snippet that demonstrates file locking:
import java.io.RandomAccessFile;
import java.nio.channels.FileChannel;
import java.nio.channels.FileLock;
public class FileLocker {
public static void main(String[] args) {
try (RandomAccessFile file = new RandomAccessFile("file.txt", "rw");
FileChannel channel = file.getChannel()) {
FileLock lock = channel.lock();
System.out.println("File locked");
// Perform operations on the locked file
lock.release();
System.out.println("File unlocked");
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we create a FileChannel
and lock the file using channel.lock()
. After performing operations on the locked file, we release the lock using lock.release()
.
In Conclusion, Here is What Matters
In this blog post, we discussed common issues related to file system integration in a Groovy issue tracker application and provided Java-based solutions for troubleshooting these issues. By leveraging Java's file handling capabilities, such as NIO and file locking, developers can effectively address file system integration problems and ensure the smooth operation of their applications.
By carefully examining file reading/writing, resolving file system paths, and handling file locking, developers can overcome common challenges in file system integration within Groovy applications. These troubleshooting techniques empower developers to build robust and reliable file system integration within their applications, enhancing the overall user experience and application performance.
I hope this blog post helps you in troubleshooting file system integration issues within your Groovy issue tracker application. Happy coding!
Feel free to provide feedback or share your own experiences with file system integration in the comments below. If you have any questions or need further assistance, don't hesitate to reach out.
Further Reading: Java NIO Overview, FileChannel Documentation