Generating DDL using Hibernate in Play Framework
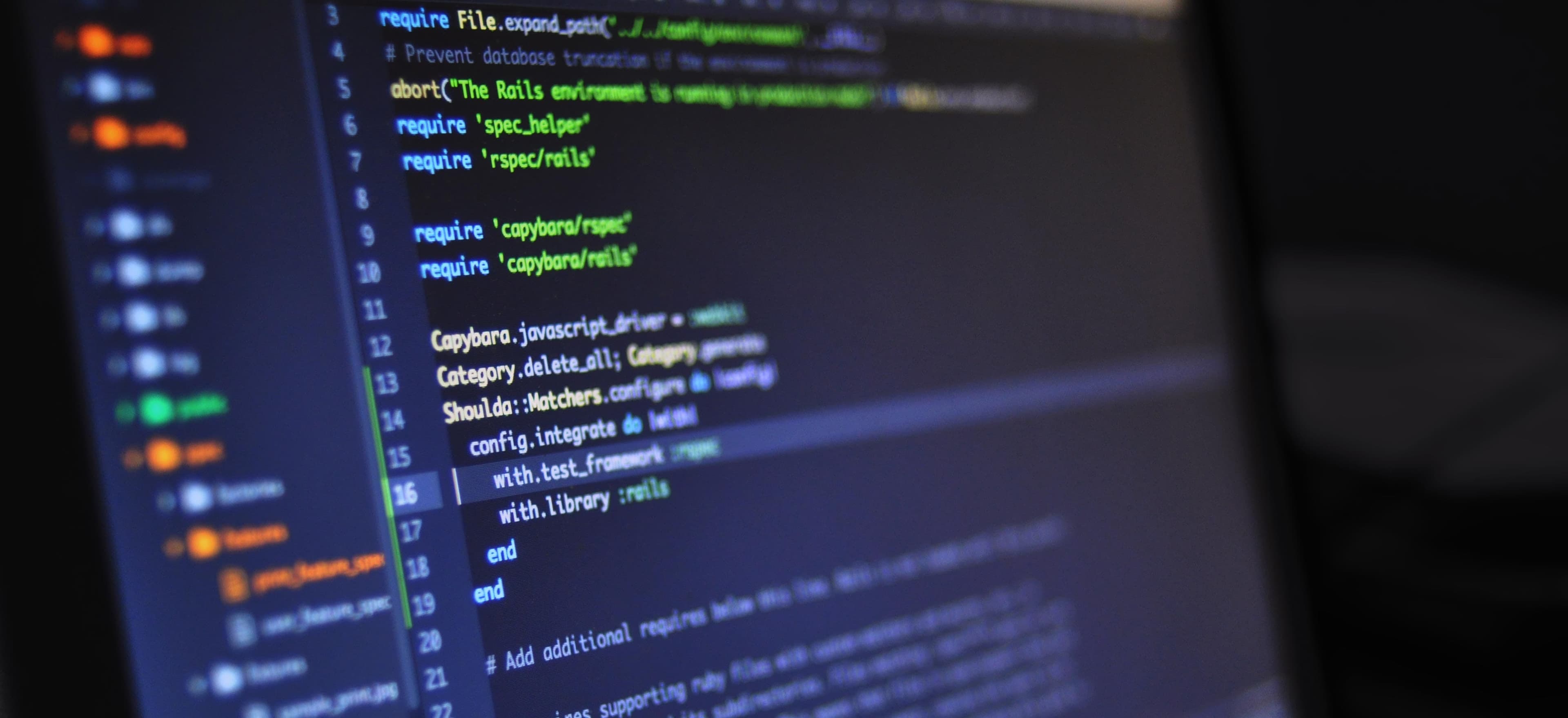
- Published on
Generating DDL using Hibernate in Play Framework
When working with Play Framework and Hibernate, you may often find the need to generate the database schema from your entity classes. This process, known as DDL (Data Definition Language) generation, can be quite useful, especially during development and testing phases. In this article, we'll explore how to generate DDL scripts using Hibernate in a Play Framework application.
Setting up Hibernate in Play Framework
Before we dive into DDL generation, let's ensure that Hibernate is properly configured within our Play Framework project. To use Hibernate in Play, you can add the hibernate-core
and hibernate-entitymanager
dependencies to your build.sbt
or build.gradle
file.
build.sbt
libraryDependencies += "org.hibernate" % "hibernate-core" % "5.4.10.Final"
libraryDependencies += "org.hibernate" % "hibernate-entitymanager" % "5.4.10.Final"
build.gradle
implementation 'org.hibernate:hibernate-core:5.4.10.Final'
implementation 'org.hibernate:hibernate-entitymanager:5.4.10.Final'
After adding the dependencies, you'll need to configure Hibernate by creating a persistence.xml
file in the conf/META-INF
directory. Here's a sample persistence.xml
file:
<?xml version="1.0"?>
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence
http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd"
version="2.1">
<persistence-unit name="defaultPersistenceUnit" transaction-type="RESOURCE_LOCAL">
<provider>org.hibernate.jpa.HibernatePersistenceProvider</provider>
<class>com.example.YourEntityClass</class>
<properties>
<property name="javax.persistence.jdbc.driver" value="org.h2.Driver"/>
<property name="javax.persistence.jdbc.url" value="jdbc:h2:mem:play"/>
<property name="javax.persistence.jdbc.user" value="sa"/>
<property name="javax.persistence.jdbc.password" value=""/>
<property name="hibernate.dialect" value="org.hibernate.dialect.H2Dialect"/>
<property name="hibernate.hbm2ddl.auto" value="create"/>
</properties>
</persistence-unit>
</persistence>
In this configuration, we specify the entity class com.example.YourEntityClass
and set the hibernate.hbm2ddl.auto
property to create
to generate DDL scripts. The hibernate.dialect
property specifies the database dialect, which can be adjusted based on your database vendor.
Generating DDL Scripts
Once Hibernate is set up and the entity classes are defined, you can generate DDL scripts by running the Play Framework application. When the application starts, Hibernate will automatically generate the DDL scripts based on the entity mappings and database dialect.
Benefits of DDL Generation
- Saves Time: DDL generation automates the process of creating database tables, saving you from writing SQL scripts manually.
- Version Control: DDL scripts can be version-controlled alongside your code, providing a comprehensive history of schema changes.
- Testing: DDL generation facilitates seamless integration with testing frameworks as it ensures that the database schema is consistent across environments.
Important Considerations
While DDL generation simplifies the initial setup and development process, it's essential to be mindful of the following considerations:
- Production Deployment: In a production environment, it's recommended to disable automatic DDL generation to prevent accidental data loss or schema inconsistencies. Instead, database changes should be managed through controlled migration scripts using tools like Flyway or Liquibase.
- Data Loss: Automatic DDL generation may lead to data loss if not handled carefully. Always back up your data before applying DDL changes in a production environment.
- Performance Impact: Generating DDL scripts at every application startup can introduce overhead, especially as the number of entities and complexity of mappings increase. Consider generating and validating DDL scripts separately in a controlled environment as the application matures.
Overriding DDL Generation Behavior
By default, Hibernate's hibernate.hbm2ddl.auto
property is set to create
, which generates DDL scripts by creating tables from scratch. However, you can override this behavior and choose from a range of options based on your requirements:
- validate: Validates the schema but does not make any changes to the database. This is useful for ensuring that the entity mappings are consistent with the database schema.
- update: Updates the schema by making changes to the database, such as adding or modifying tables/columns. Exercise caution when using this option in production, as it may result in data loss or unintended schema modifications.
- create-drop: Similar to
create
, but also drops the schema at the end of the session. This is primarily used for development and testing purposes.
Here’s an example of how you can override the default DDL generation behavior in the persistence.xml
file:
<property name="hibernate.hbm2ddl.auto" value="update"/>
The Bottom Line
In this article, we’ve explored the process of generating DDL scripts using Hibernate in a Play Framework application. By leveraging Hibernate’s DDL generation capabilities, you can streamline the database schema management, improve development efficiency, and ensure consistency across different environments. However, it’s crucial to exercise caution and consider the implications of automatic DDL generation, particularly in production scenarios. By understanding the benefits and considerations of DDL generation, you can make informed decisions when incorporating this approach into your Play Framework projects.
Now that you have a good understanding of DDL generation with Hibernate in Play Framework, you can confidently manage your database schema and streamline your development process.
Continue exploring DDL generation with Hibernate in Play Framework by checking out the official Hibernate documentation and the Play Framework documentation for more in-depth insights. Happy coding!