Uncovering Java 11's Expanded Offerings
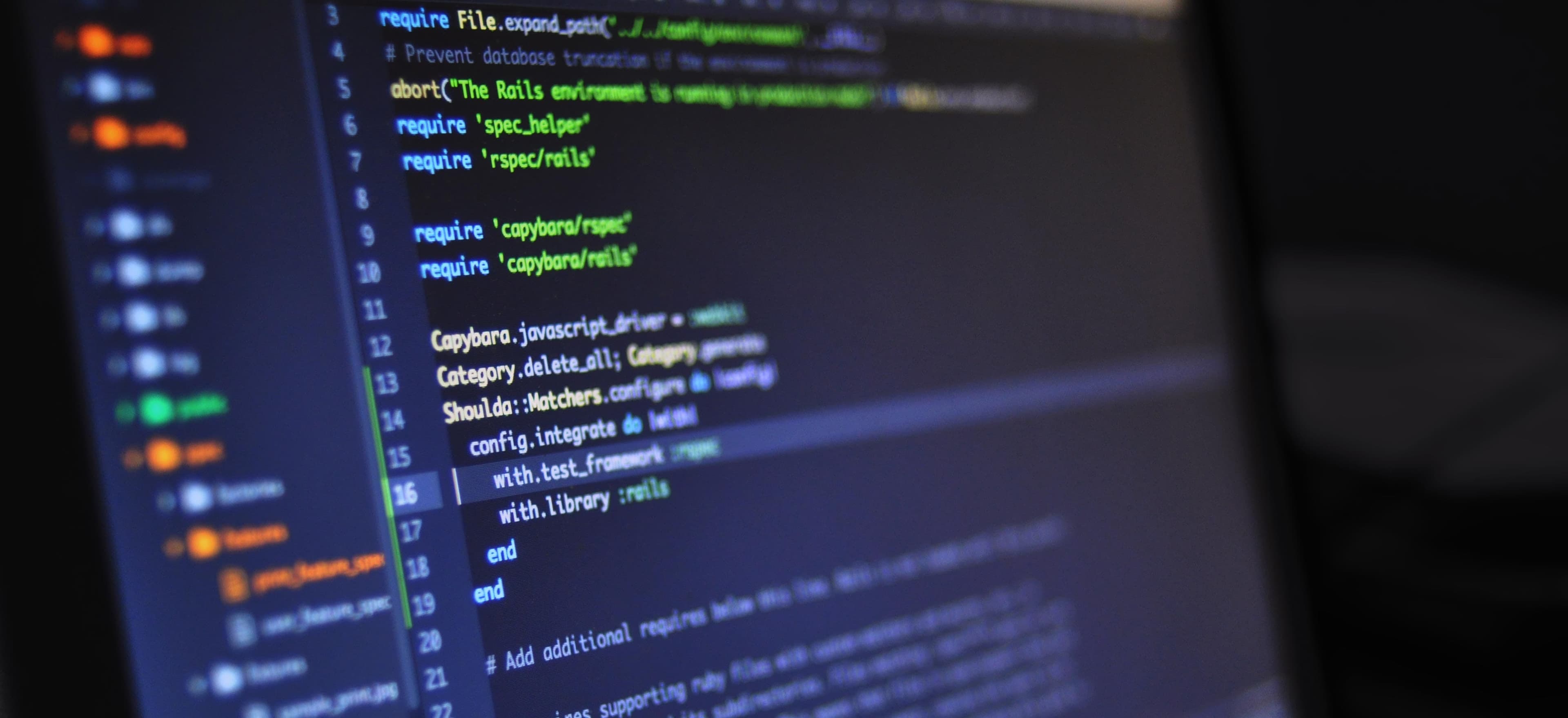
- Published on
Uncovering Java 11's Expanded Offerings
Java 11, released in September 2018, brought several new features, enhancements, and optimizations to the Java programming language. In this article, we will explore some of the most significant improvements introduced in Java 11.
Local-Variable Syntax for Lambda Parameters
One of the major enhancements in Java 11 is the introduction of the var
keyword for lambda parameters. This feature enhances the readability and conciseness of lambda expressions by allowing the use of var
in place of explicit parameter types.
// Before Java 11
someList.forEach((String s) -> System.out.println(s));
// In Java 11
someList.forEach(s -> System.out.println(s));
In the example above, using var
for lambda parameters simplifies the code by reducing unnecessary verbosity while still maintaining type safety.
HTTP Client Updates
Java 11 includes a new, standardized HTTP client in the java.net.http
package, which provides a more modern and flexible API for interacting with HTTP resources. With support for both synchronous and asynchronous requests, the new HTTP client simplifies the task of consuming RESTful web services and offers improved performance.
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.example.com/data"))
.GET()
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
The new HTTP client offers a concise and intuitive API for making HTTP requests, making it easier to work with web services in Java applications.
Epsilon Garbage Collector
Java 11 introduces the Epsilon garbage collector, a no-op garbage collector designed for performance testing and benchmarking. It is useful for scenarios where memory allocation and deallocation patterns need to be analyzed without incurring the overhead of a full-fledged garbage collector.
java -XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC MyApp
By using the Epsilon garbage collector, developers can gain insights into memory behavior and performance characteristics of their applications without the interference of an active garbage collector.
Nest-Based Access Control
With Java 11, the nestmates
attribute has been introduced to the bytecode, allowing classes to access each other's private members when they belong to the same nest. This feature improves the performance of nested classes and facilitates better encapsulation.
public class Outer {
private int outerPrivateVar;
public class Inner {
public void accessOuterPrivateVar() {
System.out.println("Outer's private variable: " + outerPrivateVar);
}
}
}
The nestmates
attribute simplifies the access control checks at runtime, leading to improved performance for nested classes.
Local-Variable Syntax for Lambda Parameters
Java 11 introduces a new HTTP client in the java.net.http
package, offering a modern and flexible API for interacting with HTTP resources.
Key Takeaways
Java 11 brought about significant improvements to the Java language, with enhancements such as the var
keyword for lambda parameters, the new HTTP client, the Epsilon garbage collector, and nest-based access control. These updates pave the way for more efficient and streamlined development of Java applications.
To make the most of Java 11's expanded offerings, it's crucial for developers to stay informed about its new features and enhancements, and to leverage them in their projects for improved productivity and performance.
In conclusion, Java 11 empowers developers with a range of new tools and features to tackle modern development challenges, making it an essential upgrade for Java projects.
With Java 11, developers can embrace improved readability and conciseness in their code, take advantage of a more advanced HTTP client for web interactions, and gain valuable insights into memory behavior and performance characteristics of their applications – all while improving the encapsulation and performance of nested classes.
Checkout our other articles