Optimizing Performance of Java Spring Web Applications
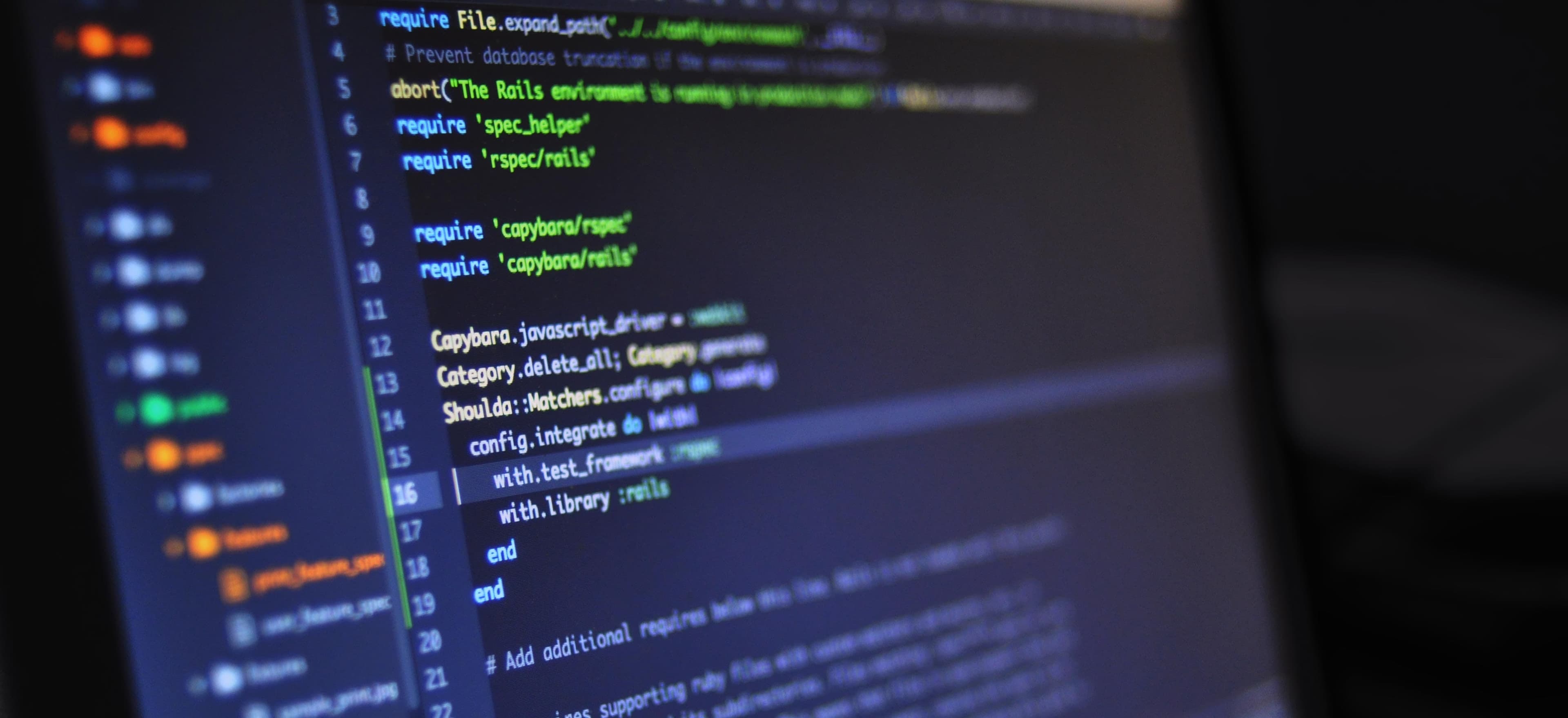
- Published on
Optimizing Performance of Java Spring Web Applications
In today's competitive digital landscape, the performance of web applications is crucial for providing users with a seamless experience. Java Spring is a popular framework for building web applications due to its flexibility, modularity, and ease of use. However, as your application grows, it's essential to ensure that it performs optimally. In this article, we'll explore various techniques and best practices for optimizing the performance of Java Spring web applications.
1. Use Efficient Data Structures and Algorithms
When working with large datasets or performing complex operations, the choice of data structures and algorithms can significantly impact performance. Utilizing efficient data structures such as HashMaps, ArrayLists, and LinkedLists can improve the speed and memory usage of your application.
Example:
Map<String, String> hashMap = new HashMap<>();
List<String> arrayList = new ArrayList<>();
Using a HashMap for fast key-value lookups or an ArrayList for constant-time random access can boost the performance of your application when dealing with data manipulation.
2. Implement Caching
Caching frequently accessed data can drastically reduce the response time of your web application. Java Spring provides built-in support for caching through annotations such as @Cacheable
and @CacheEvict
. By caching the results of expensive operations, you can avoid redundant computations and database queries, leading to improved performance.
Example:
@Cacheable("products")
public Product getProductById(Long id) {
// Fetch product from database
}
By annotating the getProductById
method with @Cacheable
, the result will be cached, and subsequent invocations with the same input will return the cached result, saving computation time.
3. Database Optimization
Efficient database queries are essential for the overall performance of a web application. Utilize indexing, query optimization, and database caching to enhance the speed of data retrieval. Additionally, consider using connection pooling to reduce the overhead of creating new database connections for each request.
Example:
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.email = ?1")
User findByEmail(String email);
}
Utilizing Spring Data JPA and writing custom query methods can optimize database interactions by fetching only the necessary data.
4. Asynchronous Processing
Performing time-consuming tasks synchronously can lead to bottlenecks in the application's responsiveness. Leveraging asynchronous processing using Java's CompletableFuture
or Spring's @Async
annotation can improve the throughput and responsiveness of the application by executing non-blocking tasks in the background.
Example:
@Async
public CompletableFuture<Order> processOrder(Order order) {
// Process order asynchronously
}
By annotating the method with @Async
, the processOrder
operation will be executed asynchronously, allowing the calling thread to continue without waiting for the result.
5. Implement Microservices Architecture
In a large-scale application, breaking down monolithic structures into smaller, independent services can distribute the load, improve scalability, and enhance performance. Java Spring provides support for building microservices through Spring Boot and Spring Cloud, facilitating the development of lightweight, independently deployable services.
Example:
@RestController
public class UserController {
@Autowired
private UserService userService;
// REST endpoints for user operations
}
By encapsulating specific functionalities into microservices, the application can achieve better performance through efficient resource utilization and targeted optimization.
6. Profiling and Monitoring
Regular profiling and monitoring of your Java Spring application using tools like JProfiler, VisualVM, or Spring Boot Actuator can help identify performance bottlenecks, memory leaks, and inefficient code. By analyzing performance metrics, you can make informed decisions on areas that require optimization.
Example:
management:
endpoints:
web:
exposure:
include: health,info,metrics
Enabling Spring Boot Actuator endpoints allows monitoring of application health, info, and metrics, providing valuable insights into the application's performance.
7. Utilize Content Delivery Networks (CDNs)
Offloading static content and assets to a Content Delivery Network (CDN) can reduce the load on the application server and improve the overall response time for users across different geographical locations. Integrating CDNs with your Java Spring web application can enhance the delivery of static resources such as images, stylesheets, and JavaScript files.
Example:
<link rel="stylesheet" href="https://cdn.example.com/style.css">
By referencing static resources from a CDN, the application can leverage the CDN's optimized delivery network, reducing latency and improving the user experience.
To Wrap Things Up
Optimizing the performance of Java Spring web applications is essential for delivering a responsive and efficient user experience. By employing best practices such as utilizing efficient data structures, implementing caching, optimizing database interactions, leveraging asynchronous processing, adopting microservices architecture, profiling and monitoring, and integrating CDNs, you can significantly improve the performance of your web application.
Incorporating these techniques ensures that your Java Spring web application remains scalable, responsive, and capable of handling increased loads, ultimately leading to enhanced user satisfaction and retention.
Remember, achieving peak performance is an ongoing process, and continuous evaluation and refinement are key to keeping your Java Spring web application at the top of its game.
Invest time in performance optimization to ensure your Java Spring web application delivers exceptional user experiences. Harness the potential of Java Spring, maximize efficiency, and revolutionize digital experiences!
Checkout our other articles