Troubleshooting Common Feign Client Configuration Issues
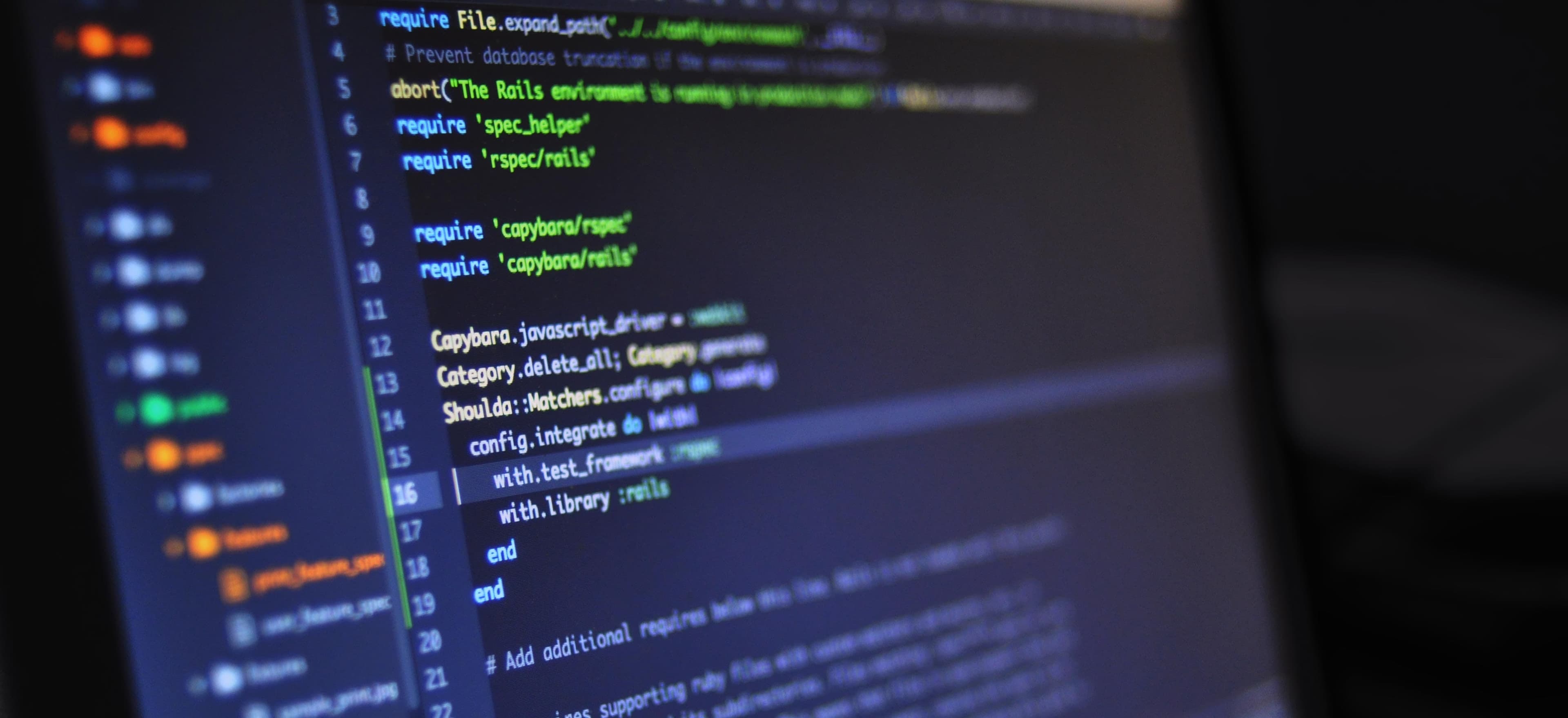
- Published on
Troubleshooting Common Feign Client Configuration Issues
Feign is a declarative web service client developed by Netflix. It simplifies the process of interacting with HTTP APIs and has gained popularity among Java developers. However, like any tool, Feign can encounter configuration issues that may impede its functionality. In this post, we will explore some common Feign client configuration issues and how to troubleshoot them.
Issue 1: Missing Feign Dependency
Problem
One of the common issues encountered when working with Feign is the absence of the Feign dependency in the project.
Solution
To resolve this, ensure that the Feign dependency is included in the project's Maven or Gradle configuration. Here's an example for Maven:
<dependency>
<groupId>io.github.openfeign</groupId>
<artifactId>feign-core</artifactId>
<version>INSERT_VERSION</version>
</dependency>
Replace INSERT_VERSION
with the appropriate version number. Then, update the project's dependencies by running mvn clean install
.
Issue 2: Incorrect Feign Client Interface
Problem
Another common issue is an incorrectly defined Feign client interface.
Solution
Ensure that the Feign client interface properly matches the structure of the target HTTP API. This includes the correct HTTP method annotations, request mappings, and method parameters. For example:
@FeignClient(name = "example", url = "https://api.example.com")
public interface ExampleClient {
@GetMapping("/resource")
String getResource();
}
Here, the @GetMapping
annotation is used to map the getResource()
method to an HTTP GET request for /resource
.
Issue 3: Missing or Incorrect Request Parameters
Problem
Issues may arise when working with request parameters in Feign client methods.
Solution
Ensure that request parameters are incorporated correctly into the Feign client method signature. This includes using the @RequestParam
annotation for query parameters and properly mapping them to method parameters. For example:
@FeignClient(name = "example", url = "https://api.example.com")
public interface ExampleClient {
@GetMapping("/resource")
String getResource(@RequestParam("id") Long id);
}
In this case, the id
parameter is defined using the @RequestParam
annotation to map it to the query parameter in the request.
Issue 4: SSL/TLS Configuration
Problem
SSL/TLS configuration issues can also impact Feign client functionality, especially when communicating with secure endpoints.
Solution
Ensure that the SSL/TLS configuration is set up correctly. This may involve configuring the appropriate truststore, keystore, or SSL certificates depending on the target endpoint. Additionally, consider using libraries like javax.net.ssl.HttpsURLConnection
to customize SSL/TLS configuration.
Issue 5: Connection Timeout and Retries
Problem
Feign client may encounter connectivity issues due to connection timeouts or the need for retry mechanisms.
Solution
Configure connection timeouts and retry mechanisms in the Feign client to handle connectivity issues gracefully. This can be achieved through Feign client configuration properties or custom error handling. For example, you can use the Feign.builder()
to customize the client with timeouts and retries:
ExampleClient exampleClient = Feign.builder()
.options(new Request.Options(5000, 3000))
.retryer(new Retryer.Default(100, 1000, 3))
.target(ExampleClient.class, "https://api.example.com");
In this snippet, we set the connection timeout to 5 seconds and configure a retry mechanism with a maximum of 3 attempts.
Issue 6: Error Decoding and Handling
Problem
Handling and decoding errors from the Feign client can be challenging when not properly configured.
Solution
Implement error decoding and handling mechanisms to interpret error responses from the server. This may involve creating custom error decoder implementations to parse error messages and HTTP status codes. Consider utilizing Feign's ErrorDecoder
to customize error handling behavior based on server responses.
Issue 7: Feign Client Logging
Problem
Troubleshooting issues with Feign client interactions can be difficult without proper logging.
Solution
Enable Feign client logging to capture detailed interactions between the client and the server. This can be achieved by setting the logging level for the Feign client interface, as well as configuring the underlying HTTP client for request and response logging.
Closing the Chapter
By addressing these common Feign client configuration issues and their respective solutions, developers can effectively troubleshoot and optimize their Feign client implementations. From resolving dependency problems to fine-tuning SSL/TLS configurations and enabling comprehensive logging, mastering these troubleshooting techniques is essential for leveraging the full potential of Feign in Java applications. For additional resources and best practices, refer to the Feign GitHub repository and the official Feign documentation.