Interfacing Multiple Sensors with Raspberry Pi
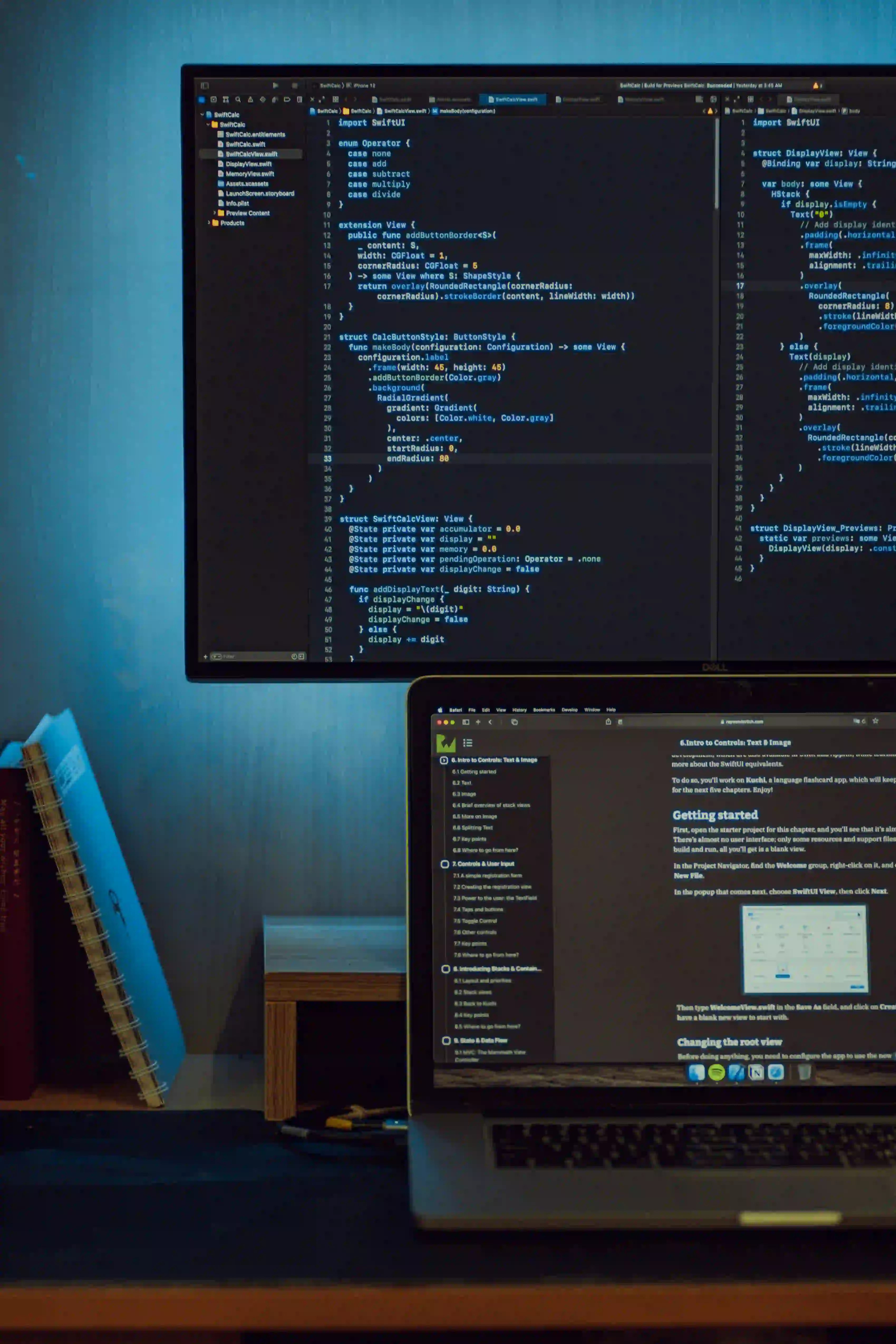
Harnessing the Power of Java for Integrating Multiple Sensors with Raspberry Pi
In the fast-evolving technological landscape, the concept of the Internet of Things (IoT) has gained immense traction. The Raspberry Pi, a versatile and cost-effective microcontroller, has become a preferred choice for IoT projects due to its feature-rich GPIO (General Purpose Input/Output) pins that enable effortless interfacing with various sensors. By leveraging Java's robustness, developers can seamlessly integrate multiple sensors with Raspberry Pi to create powerful IoT applications.
Why Java?
Java, renowned for its platform independence, and robust libraries, is an ideal choice for Raspberry Pi development. It offers a familiar and reliable environment for building IoT applications, with the added advantage of a vast ecosystem of libraries for sensor integration, data processing, and networking. With the ability to run Java on Raspberry Pi, developers can streamline the integration of multiple sensors and harness the full potential of this microcontroller.
Setting up the Development Environment
Before delving into sensor integration, ensure that Java is installed on the Raspberry Pi. The java -version
command can be used to verify the installation. If Java is not present, it can be installed using the following commands:
sudo apt update
sudo apt upgrade
sudo apt install default-jdk
Interfacing Sensors
1. Temperature and Humidity Sensor (DHT11)
The DHT11 sensor, used for monitoring temperature and humidity, can be interfaced with Raspberry Pi using Java. The Pi4J library provides seamless access to GPIO pins, facilitating easy sensor integration. The following Java code snippet illustrates the process of reading data from the DHT11 sensor:
import com.pi4j.component.temperature.TemperatureSensor;
import com.pi4j.io.gpio.Pin;
import com.pi4j.io.gpio.RaspiPin;
import com.pi4j.io.gpio.digital.DigitalInput;
import com.pi4j.io.gpio.digital.DigitalState;
import com.pi4j.platform.PlatformAlreadyAssignedException;
import com.pi4j.temperature.TemperatureScale;
import com.pi4j.temperature.impl.TemperatureSensorComponent;
public class DHT11Sensor {
public static void main(String[] args) throws InterruptedException, PlatformAlreadyAssignedException {
// Define the GPIO pin for sensor data
Pin sensorPin = RaspiPin.GPIO_04;
// Create digital input instance
DigitalInput sensor = gpio.provisionDigitalInputPin(sensorPin);
// Read sensor data
while(true){
if (sensor.getState().equals(DigitalState.HIGH)) {
// Read temperature and humidity from the sensor
TemperatureSensorComponent sensorComponent = new TemperatureSensorComponent(sensorPin, TemperatureScale.CELSIUS);
double temperature = sensorComponent.getTemperature();
double humidity = sensorComponent.getHumidity();
System.out.println("Temperature: " + temperature + "°C, Humidity: " + humidity + "%");
Thread.sleep(2000); // Delay
}
}
}
}
2. Motion Sensor (PIR)
The Passive Infrared (PIR) motion sensor, capable of detecting movement, can be interfaced with Raspberry Pi using Java and the Pi4J library. Below is a simple Java code snippet demonstrating the integration of a PIR motion sensor:
import com.pi4j.io.gpio.GpioPinDigitalInput;
import com.pi4j.io.gpio.GpioFactory;
import com.pi4j.io.gpio.PinPullResistance;
import com.pi4j.io.gpio.RaspiPin;
public class PIRMotionSensor {
public static void main(String[] args) throws InterruptedException {
// Initialize GPIO
final GpioController gpio = GpioFactory.getInstance();
// Define the GPIO pin for sensor data
GpioPinDigitalInput sensor = gpio.provisionDigitalInputPin(RaspiPin.GPIO_02, PinPullResistance.PULL_DOWN);
// Read sensor data
while(true) {
if(sensor.isHigh()) {
System.out.println("Motion Detected");
Thread.sleep(2000); // Delay
}
}
}
}
Data Processing and Networking
Java provides a plethora of libraries for data processing and networking, essential for IoT applications. When interfacing with multiple sensors, it's crucial to process the data efficiently and transmit it to a remote server or database. The following libraries can be leveraged for data processing and networking:
- JSON Processing (javax.json): Ideal for parsing and constructing JSON data, which is widely used for data exchange in IoT applications.
- MQTT (Eclipse Paho): A lightweight messaging protocol suitable for IoT communication, facilitating seamless integration with MQTT brokers.
- Apache Kafka: For scalable and distributed data streaming, essential for handling large volumes of sensor data.
Closing the Chapter
Java's versatility and extensive libraries make it a compelling choice for integrating multiple sensors with Raspberry Pi. By harnessing Java's power, developers can effortlessly interface with sensors, process data, and establish robust networking for IoT applications. The code snippets provided offer a glimpse into the seamless integration of sensors, thereby exemplifying the potential of Java for IoT projects. Embrace the power of Java to unlock the full potential of your Raspberry Pi-based IoT ventures.
In conclusion, the combination of Java and Raspberry Pi presents an exciting opportunity to delve into the realm of IoT with multiple sensors at your fingertips! Harness the power of Java and Raspberry Pi to embark on your IoT journey today.
So, are you ready to explore the endless possibilities of Java and Raspberry Pi in the world of IoT? Let's embark on this exciting journey together!