Optimizing CRUD Operations with Spring Data Solr
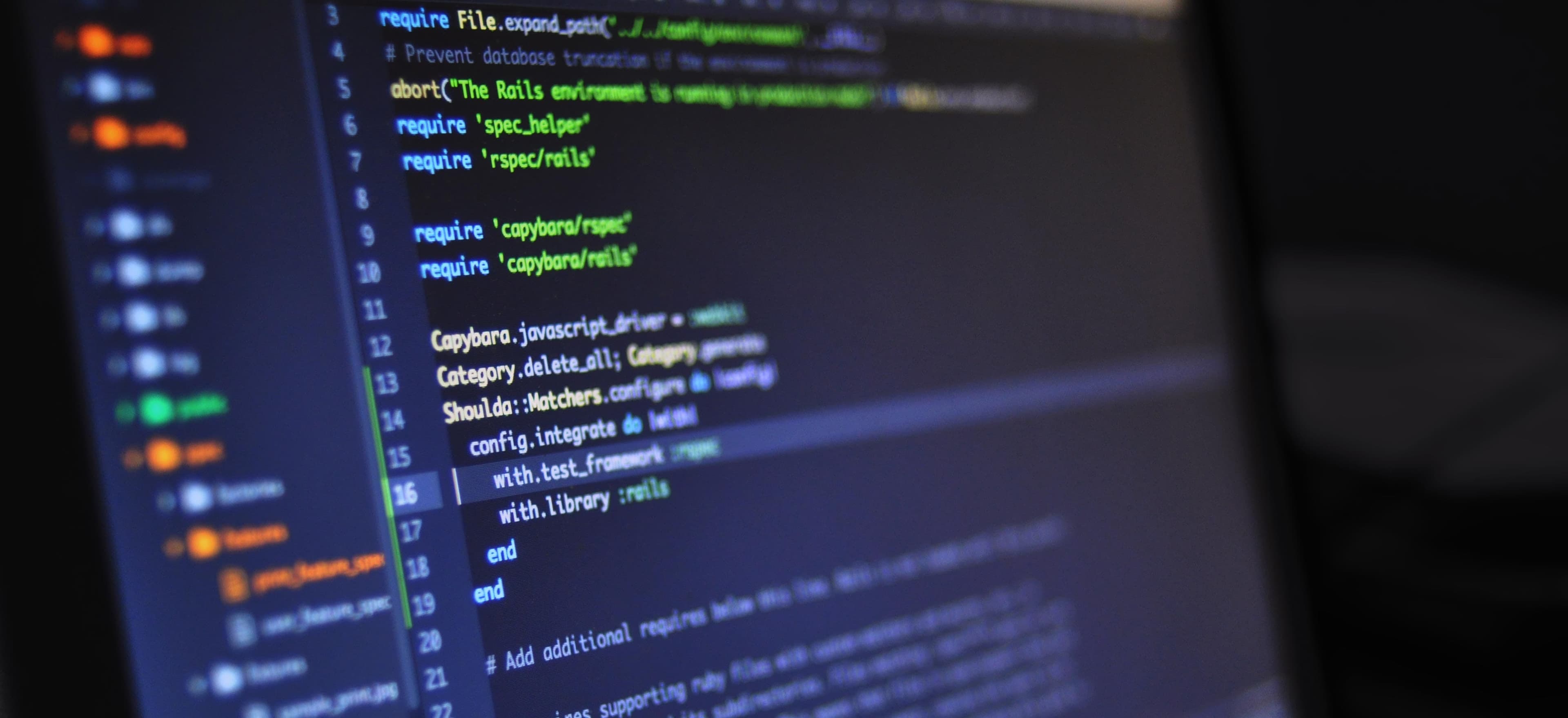
- Published on
Optimizing CRUD Operations with Spring Data Solr
In the world of modern web development, efficient management of data is critical. When dealing with large volumes of data, it becomes even more important to optimize CRUD (Create, Read, Update, Delete) operations. In this blog post, we will explore how to optimize CRUD operations using Spring Data Solr, a powerful tool for integrating Solr, the popular search platform, with Spring applications.
What is Spring Data Solr?
Spring Data Solr is part of the larger Spring Data project, which aims to simplify the development of data-centric applications. It provides a high-level abstraction for interacting with Solr, allowing developers to use familiar Spring patterns while working with Solr.
One of the key features of Spring Data Solr is its support for CRUD operations, making it easier to create, read, update, and delete documents in a Solr collection. However, to ensure optimal performance, it's essential to understand how to make the most of these CRUD operations.
Optimizing CRUD Operations in Spring Data Solr
1. Use Bulk Operations for Writing
When dealing with a large number of documents, it's more efficient to use bulk operations for writing data to Solr. Spring Data Solr provides support for bulk operations, allowing you to save or delete multiple documents in a single request.
// Example of using bulk operations to save documents
List<Product> products = // retrieve products from somewhere
solrTemplate.saveBeans(products);
solrTemplate.commit();
By using bulk operations, you can minimize the overhead of making multiple requests to the Solr server, thus improving the overall performance of write operations.
2. Leverage Spring Data Solr Repositories
Spring Data Solr provides repositories that offer a high-level, domain-specific interface for performing CRUD operations. By defining a repository interface and extending SolrCrudRepository
, you can take advantage of built-in methods for common operations such as save, delete, and findById.
public interface ProductRepository extends SolrCrudRepository<Product, String> {
// Additional custom query methods can be defined here
}
Using Spring Data Solr repositories not only simplifies the code but also allows for optimizations performed under the hood, such as batching and caching, to enhance the efficiency of CRUD operations.
3. Utilize Optimized Query Methods
When performing read operations, it's crucial to leverage optimized query methods provided by Spring Data Solr. These methods allow you to define complex query criteria using method names, which are then translated into efficient Solr queries.
// Example of using optimized query methods
List<Product> findByNameAndCategory(String name, String category);
By using optimized query methods, you can benefit from the automatic generation of query execution plans tailored for the specific search criteria, leading to faster and more efficient read operations.
4. Implement Partial Updates
In scenarios where you need to update only specific fields of a document, Spring Data Solr supports partial updates. This feature allows you to modify individual fields without having to reindex the entire document.
// Example of partial update using Spring Data Solr
Product product = // retrieve a product by id
product.setPrice(newPrice);
solrTemplate.saveBean(product);
solrTemplate.commit();
By implementing partial updates, you can minimize the overhead of updating large documents and reduce the indexing time, resulting in more efficient update operations.
A Final Look
Optimizing CRUD operations is essential for achieving the best possible performance when working with large datasets in Solr. By leveraging features such as bulk operations, Spring Data Solr repositories, optimized query methods, and partial updates, you can significantly improve the efficiency of CRUD operations in your Spring applications.
In this blog post, we have covered some of the key strategies for optimizing CRUD operations with Spring Data Solr. By implementing these best practices, you can ensure that your application interacts with Solr in the most efficient manner, delivering optimal performance for data management operations.
For further reading on Spring Data Solr, you may want to explore the official Spring Data Solr documentation and the Spring Data Solr GitHub repository for additional insights and updates.
Checkout our other articles