Troubleshooting Spring Security's Multiple Filter Chain Issues
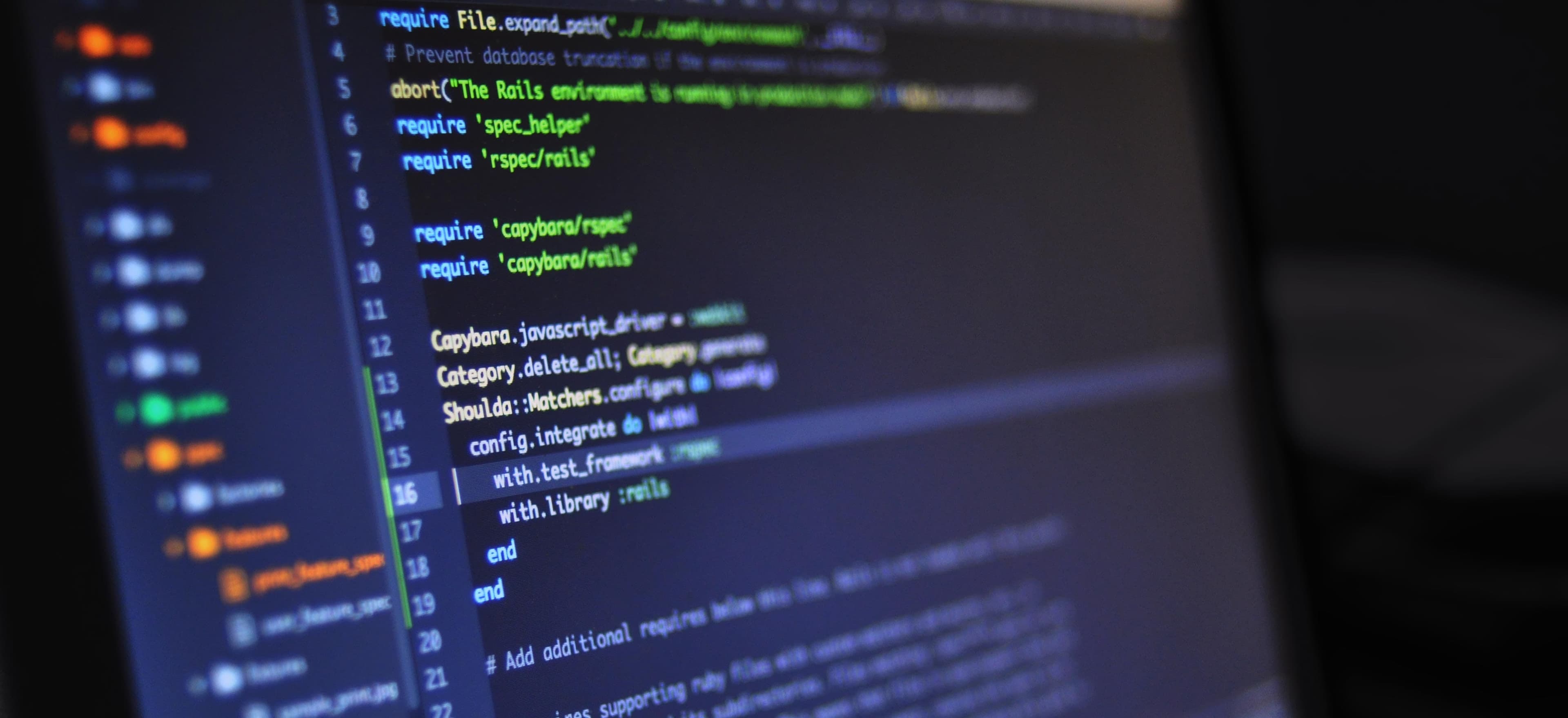
- Published on
Troubleshooting Spring Security's Multiple Filter Chain Issues
Spring Security is a powerful and customizable authentication and access-control framework for Java applications. However, when implementing multiple filter chains within a single application, developers often encounter various issues that can significantly complicate the security configuration. In this blog post, we will discuss common problems tied to multiple filter chains in Spring Security and provide targeted solutions to address these issues.
Understanding Filter Chains in Spring Security
Before diving into troubleshooting, let's briefly clarify what filter chains are. In Spring Security, a filter chain is a sequence of security filters that are invoked in a defined order to secure web requests. This allows developers to apply different security rules to different endpoints, which is particularly useful in applications that serve various functionalities or user roles.
Basic Configuration of Filter Chains
Here’s a simplified example of how multiple filter chains can be set up in a Spring Boot application:
@EnableWebSecurity
public class SecurityConfiguration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
// First filter chain: secure /admin paths
http
.requestMatchers()
.antMatchers("/admin/**")
.and()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
// Second filter chain: secure /user paths
http
.requestMatchers()
.antMatchers("/user/**")
.and()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
In this example, we define two different filter chains: one for admin access and another for user access. Each chain has custom security configurations that can cater to specific requirements.
Common Issues with Multiple Filter Chains
1. Configuration Overlaps
One of the most common issues arises when the configurations for multiple filter chains overlap, causing unpredictable behavior. For instance, rules defined in one filter chain can unintentionally affect another.
Solution: Ensure that each filter chain applies to distinct paths and that URL patterns are clearly defined to prevent overlaps. Use requestMatchers()
judiciously.
2. Incorrect Order of Filters
The order in which security filters are executed can change the desired outcome. Filters are executed in the order they are defined, and misconfiguring this order can lead to security loopholes.
Solution: Explicitly set the order of each filter chain using the order
attribute. This helps you control which filter chain should take precedence.
Here’s how you can specify the order:
@Bean
public SecurityFilterChain adminFilterChain(HttpSecurity http) throws Exception {
return http.requestMatchers().antMatchers("/admin/**")
.and()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.build();
}
@Bean
public SecurityFilterChain userFilterChain(HttpSecurity http) throws Exception {
return http.requestMatchers().antMatchers("/user/**")
.and()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.build();
}
Both methods utilized build()
to finalize the filter chain and prevent issues stemming from overlapping rules.
3. Authentication Issues
When using multiple filter chains, authentication issues often arise due to mismatched authentication providers. For example, one chain might expect token-based authentication, while another may rely on form-based authentication.
Solution: Ensure that all filter chains have the appropriate authentication mechanisms defined. Review each chain’s authentication provider configuration.
Example of Authentication Configuration
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin")
.password(passwordEncoder().encode("admin"))
.roles("ADMIN")
.and()
.withUser("user")
.password(passwordEncoder().encode("user"))
.roles("USER");
}
By defining the users and their roles clearly, you can avoid common pitfalls with authentication across multiple chains.
4. CSRF Protection Issues
Cross-Site Request Forgery (CSRF) protection might behave differently across filter chains when not configured properly. Consequentially, requests might be denied erroneously.
Solution: Configure CSRF protection per filter chain if necessary. Normally, a default CSRF configuration applies to all requests, but you can tailor it per path.
Example of CSRF Configuration
http.csrf().ignoringAntMatchers("/api/**");
In this example, requests to /api/**
are exempted from CSRF checks, which might be useful for REST APIs.
Debugging Tips
When troubleshooting filter chain issues, use the following strategies:
-
Log Levels: Increase the logging level of Spring Security to debug or trace. This can provide insights into which filters are being executed.
-
Debugging Breakpoints: Use IDE debugging capabilities to set breakpoints within the filter chain execution. This helps track how requests flow through the security filters.
-
Test with Postman: Use tools like Postman to manually send requests through different paths while observing the response and any security exceptions.
Additional Resources
For deeper insights into Spring Security and its extensive capabilities, consider checking out:
The Closing Argument
Troubleshooting multiple filter chain issues in Spring Security may seem daunting initially, but with a structured approach, it can be manageable. By understanding filter chain configurations, ensuring that they do not overlap, carefully ordering filters, and explicitly defining authentication and CSRF protections, you can create a secure and efficient Spring application.
Remember to regularly evaluate your security configuration to avoid vulnerabilities as your application evolves. With the insights and strategies provided in this blog post, you can effectively tackle multiple filter chain challenges with confidence. Happy coding!