Optimizing Battery Usage for Android Services
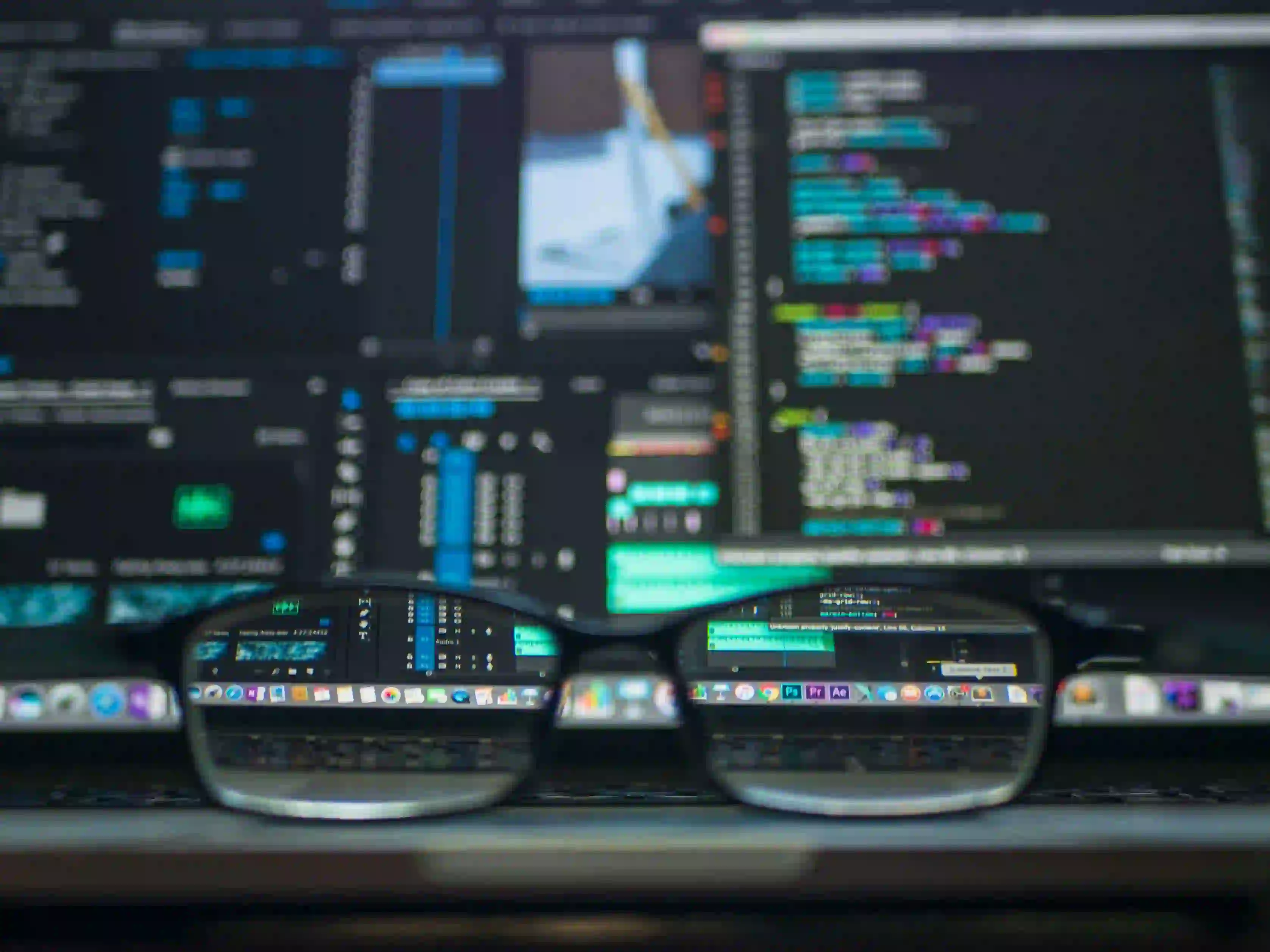
Optimizing Battery Usage for Android Services
In the world of mobile app development, optimizing battery usage is crucial for providing a smooth user experience. When it comes to Android app development, services play a vital role in performing background tasks. However, these services can potentially drain the device's battery if not optimized properly. In this article, we will explore some best practices for optimizing battery usage for Android services.
Understanding the Impact of Services on Battery Life
Before delving into optimization techniques, it's essential to understand how services can impact battery life. Android services, especially those running in the background, consume system resources such as CPU, memory, and network, which can lead to increased power consumption.
When services are not optimized, they can keep the device awake for prolonged periods, preventing it from entering low-power states. This continuous activity can significantly impact battery life and overall device performance.
Best Practices for Optimizing Battery Usage
1. Implementing Foreground Services When Necessary
While background services are essential for certain tasks, it's important to utilize foreground services when the user's attention is required. Foreground services display a notification to the user, indicating that the app is performing a task in the foreground, and they are less likely to be terminated by the system. By using foreground services judiciously, developers can ensure that the app consumes battery power only when it's truly necessary.
public class MyForegroundService extends Service {
// Implement foreground service logic here
// ...
}
2. Minimizing Wake Lock Usage
Wake locks are a common source of battery drain. When a wake lock is acquired and held, it prevents the device from entering deep sleep modes, thus consuming more power. It's crucial to minimize the use of wake locks and release them as soon as they are no longer needed.
3. Optimizing Network Operations
Services often require network connectivity for various tasks such as fetching data or performing updates. Optimizing network operations by using techniques like batching network requests, leveraging push notifications, and minimizing polling intervals can significantly reduce power consumption.
4. Implementing JobScheduler
JobScheduler is a powerful API introduced in Android Lollipop (API level 21) that allows developers to defer background tasks and optimize them based on various criteria such as network availability, charging status, and device idle state. By using JobScheduler, developers can schedule tasks to run at an appropriate time, thus minimizing the impact on battery life.
JobInfo jobInfo = new JobInfo.Builder(jobId, new ComponentName(context, MyJobService.class))
.setRequiredNetworkType(JobInfo.NETWORK_TYPE_ANY)
.setRequiresCharging(true)
.build();
5. Properly Managing Resources
It's essential to efficiently manage resources such as CPU, memory, and disk I/O within services. Resource-intensive tasks should be optimized to minimize their impact on battery life. For example, using efficient algorithms and data structures can reduce CPU utilization, while proper resource cleanup can prevent unnecessary memory and disk usage.
Monitoring Battery Usage
In addition to implementing optimization techniques, monitoring battery usage is essential for identifying potential issues. Android provides built-in tools for monitoring battery usage, such as Battery Historian and Battery Usage section in the device settings. By regularly monitoring battery usage, developers can identify any abnormal consumption patterns and fine-tune their optimization strategies accordingly.
Final Thoughts
Optimizing battery usage for Android services is a critical aspect of developing high-quality, user-friendly apps. By implementing best practices such as utilizing foreground services, minimizing wake lock usage, optimizing network operations, leveraging JobScheduler, and efficiently managing resources, developers can significantly reduce the app's impact on battery life. Additionally, monitoring battery usage helps in identifying and addressing any potential issues. By following these best practices and continuously optimizing battery usage, developers can ensure that their Android apps deliver a seamless user experience while minimizing battery drain.
In conclusion, optimizing battery usage for Android services is a multifaceted endeavor, requiring a combination of prudent coding practices, effective utilization of system APIs, and the ongoing scrutiny of battery consumption patterns. By incorporating these best practices and remaining vigilant in the monitoring of battery usage, developers can ensure their Android apps are optimized for minimal battery impact, thereby delivering a superior user experience.
Remember, batteries last longer when developers work smarter, not harder.