Integrating AWS Lambda with Java and DynamoDB
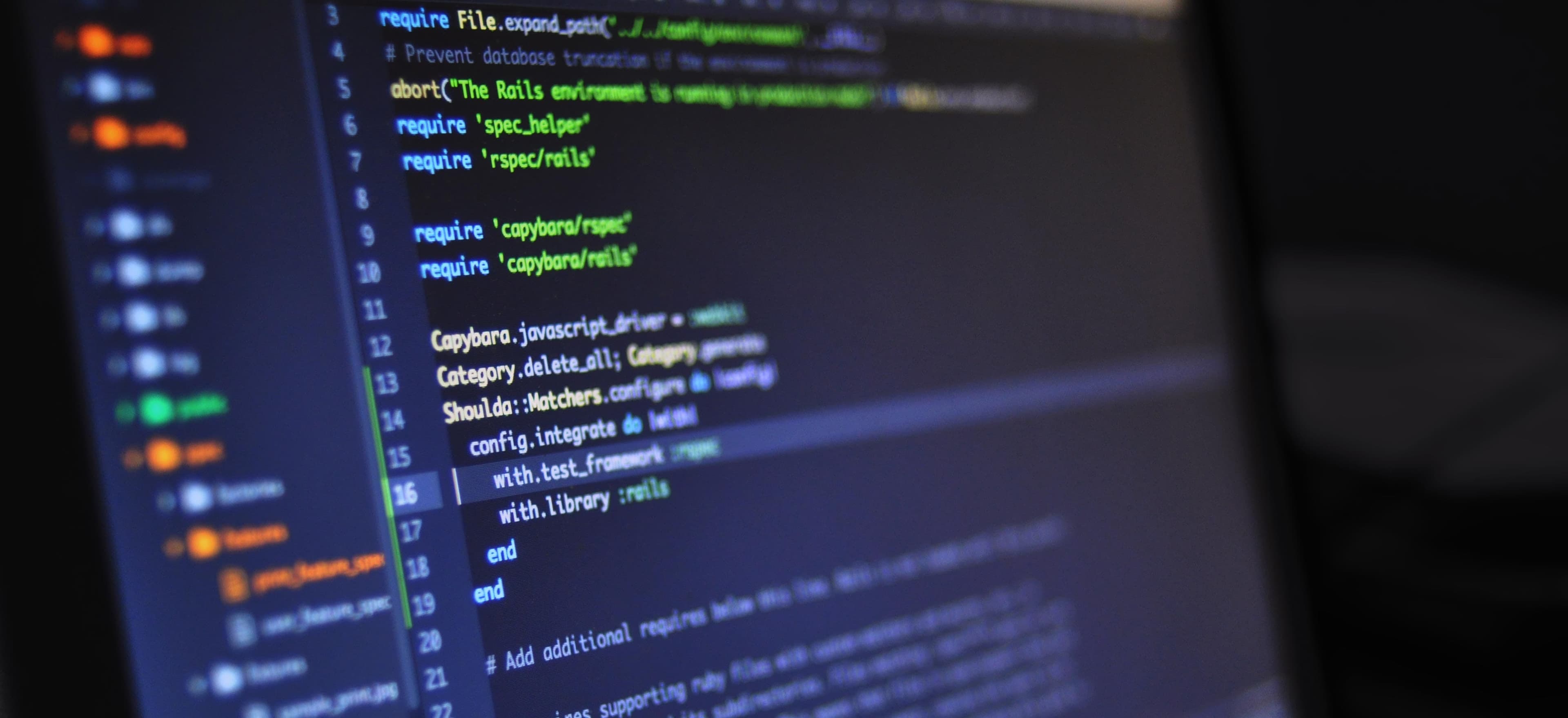
- Published on
Integrating AWS Lambda with Java and DynamoDB
In this blog post, we will explore how to leverage AWS Lambda, Java, and DynamoDB to build a serverless application. AWS Lambda is a serverless computing service that lets you run code without provisioning or managing servers. DynamoDB is a fully managed NoSQL database service provided by AWS. By integrating these services, we can create a scalable and cost-effective architecture for our applications.
Setting Up the Environment
Before we dive into the code, you'll need to have the following set up:
- AWS account
- AWS CLI installed and configured
- Java Development Kit (JDK) installed
- AWS SDK for Java added to your project
Creating the Lambda Function
Let's start by creating a simple Lambda function that interacts with DynamoDB. We'll create a function that reads data from a DynamoDB table.
First, we need to create a new Lambda function in the AWS Management Console or using the AWS CLI. Once the function is created, we can start writing the code.
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDB;
import com.amazonaws.services.dynamodbv2.AmazonDynamoDBClientBuilder;
import com.amazonaws.services.dynamodbv2.model.AttributeValue;
import com.amazonaws.services.dynamodbv2.model.GetItemRequest;
import com.amazonaws.services.dynamodbv2.model.GetItemResult;
public class ReadFromDynamoDB implements RequestHandler<String, String> {
private final AmazonDynamoDB dynamoDB = AmazonDynamoDBClientBuilder.defaultClient();
private final String tableName = "YourDynamoDBTableName";
@Override
public String handleRequest(String input, Context context) {
GetItemRequest getItemRequest = new GetItemRequest()
.withKey(Map.of("id", new AttributeValue().withS(input)))
.withTableName(tableName);
GetItemResult result = dynamoDB.getItem(getItemRequest);
// Process the result and return
return result.getItem().toString();
}
}
In this code, we have created a Lambda function that reads an item from a DynamoDB table using the AmazonDynamoDB
client provided by the AWS SDK for Java. We handle the request using the handleRequest
method, where we construct a GetItemRequest
and retrieve the result from DynamoDB. Finally, we process the result and return it.
Why This Code Works
- We use the
AmazonDynamoDBClientBuilder
to create an instance ofAmazonDynamoDB
, which represents the entry point to interact with DynamoDB. - The
handleRequest
method is the entry point of the Lambda function and is invoked when the Lambda function is triggered. - We construct a
GetItemRequest
with the necessary parameters to retrieve the item from DynamoDB. - Finally, we process the result and return it from the Lambda function.
Configuring Permissions and Triggers
Once the Lambda function is created, we need to configure the necessary permissions and triggers. For the Lambda function to access DynamoDB, it needs the appropriate permissions. This can be done by attaching an IAM role to the Lambda function with the required permissions to access DynamoDB.
Additionally, we can configure triggers for the Lambda function. For example, we can set up an API Gateway trigger to allow the Lambda function to be invoked via HTTP requests.
Testing the Integration
After the setup is complete, we can test the integration by invoking the Lambda function and observing the interaction with DynamoDB. We can use the AWS Management Console, AWS CLI, or SDKs to invoke the Lambda function and pass input to trigger the execution.
Closing Remarks
In this blog post, we explored how to integrate AWS Lambda with Java and DynamoDB to create a serverless application. We created a simple Lambda function that reads data from a DynamoDB table, and we discussed the necessary configuration and testing steps. By leveraging these AWS services, we can build scalable and cost-effective applications while focusing on the business logic rather than infrastructure management.
Integrating AWS Lambda with Java and DynamoDB provides a powerful and flexible platform for building serverless applications, and understanding the process is essential for modern application development.
Remember to check the AWS Lambda documentation and the DynamoDB developer guide for more in-depth information and best practices. Happy coding!