Maximizing IT Architecture Efficiency with Three Key Laws
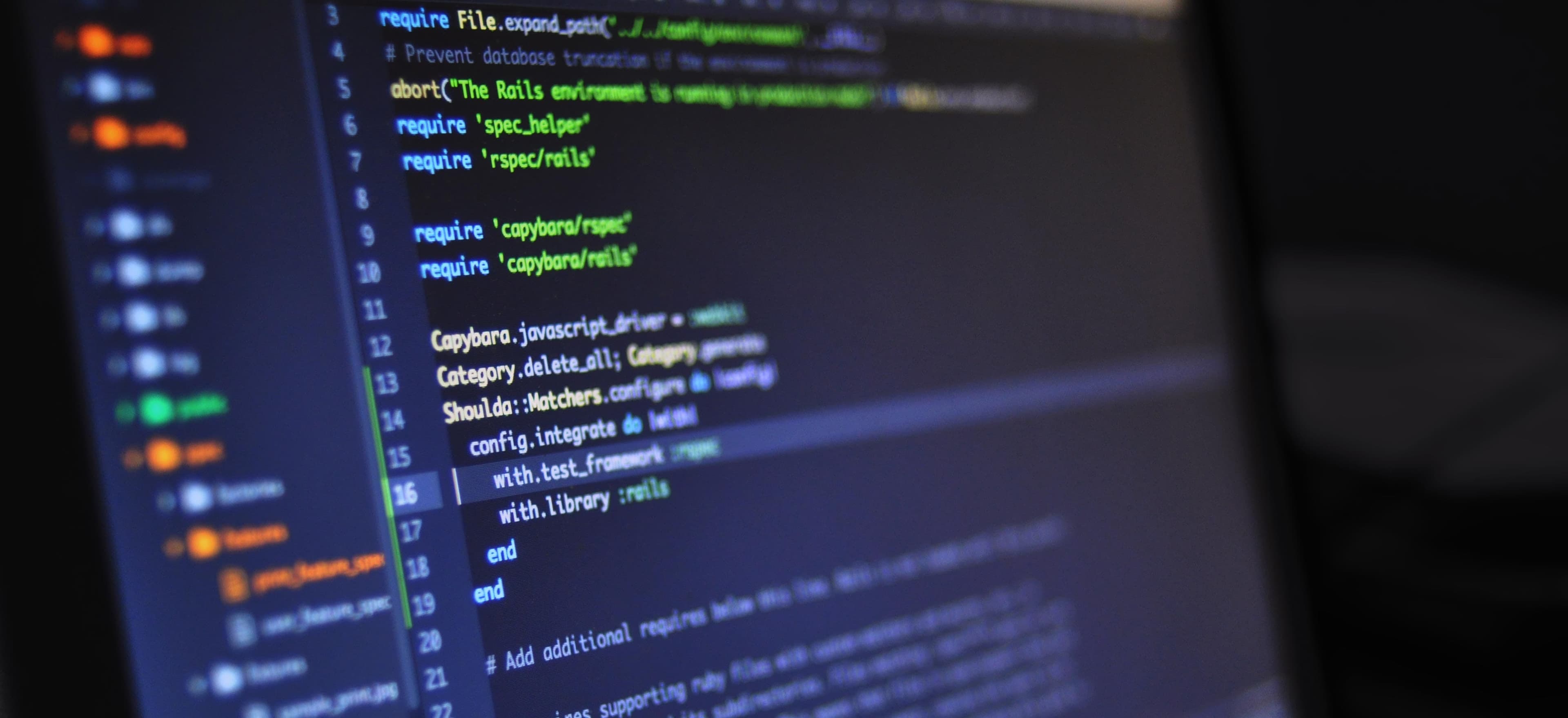
- Published on
Maximizing IT Architecture Efficiency with Three Key Laws
In the realm of IT architecture, efficiency is not just a buzzword; it's a crucial element that can make or break the success of a software system. To achieve optimal efficiency in IT architecture, it's essential to adhere to certain fundamental principles and laws. In this article, we'll explore three key laws that can significantly impact the efficiency of IT architecture and how Java, as a versatile programming language, can be leveraged to uphold these laws.
Law 1: Amdahl's Law
Amdahl's Law, formulated by computer architecture pioneer Gene Amdahl, states that the speedup of a program using multiple processors in parallel computing is limited by the time needed for the sequential fraction of the program. In simpler terms, it emphasizes the significance of identifying and optimizing the non-parallelizable portions of a program to enhance overall efficiency.
In the context of Java, leveraging multi-threading is a powerful technique to harness parallel computing capabilities. Let's consider a scenario where a Java application needs to process a large volume of data. By utilizing the java.util.concurrent
package to create multiple threads, the non-parallelizable tasks can be executed concurrently, effectively reducing the overall processing time.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class DataProcessor {
public void process() {
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
executor.execute(new DataProcessingTask());
}
executor.shutdown();
}
}
In this example, the DataProcessor
class utilizes an ExecutorService
to manage a pool of threads, enabling parallel execution of DataProcessingTask
instances. By adhering to Amdahl's Law and identifying the sequential bottlenecks, the Java application maximizes its efficiency by exploiting parallelism.
Law 2: Conway's Law
Conway's Law, coined by computer programmer Melvin Conway, posits that organizations design systems that mirror their own communication structure. This principle underscores the profound influence of organizational dynamics on the architecture of software systems. To maximize IT architecture efficiency in alignment with Conway's Law, it's imperative to foster cohesive collaboration and communication among cross-functional teams.
In the context of Java development, the application of Conway's Law involves adopting a modular and well-structured approach to software design. By employing Java's package and module system, developers can encapsulate related functionalities and promote clear interfaces between different modules, essentially mirroring effective communication within the organizational structure.
package com.example.module1;
public class Module1Class {
// Class implementation for module 1
}
package com.example.module2;
public class Module2Class {
// Class implementation for module 2
}
The use of Java packages to organize classes into cohesive units aligns with Conway's Law, fostering a reflective architecture that echoes effective communication structures within the organization.
Law 3: The Law of Demeter
The Law of Demeter, also known as the principle of least knowledge, advocates for minimizing the connections between different classes by restricting their direct interactions. This principle contributes to improved maintainability, reusability, and modularity of the software system, ultimately enhancing its efficiency.
In the realm of Java programming, adhering to the Law of Demeter involves promoting loose coupling between classes. By utilizing appropriate design patterns, such as the Facade pattern, Java developers can encapsulate complex subsystems and provide simplified interfaces, thereby adhering to the principle of least knowledge.
public class SubsystemA {
public void operationA() {
// Operation implementation
}
}
public class SubsystemB {
public void operationB() {
// Operation implementation
}
}
public class Facade {
private SubsystemA subsystemA;
private SubsystemB subsystemB;
public Facade() {
this.subsystemA = new SubsystemA();
this.subsystemB = new SubsystemB();
}
public void facadeOperation() {
// Complex operations using subsystemA and subsystemB
}
}
In this example, the Facade
class acts as a simplified interface to the complex subsystems (SubsystemA
and SubsystemB
), thus promoting adherence to the Law of Demeter and enhancing the efficiency of the IT architecture.
By incorporating Amdahl's Law, Conway's Law, and the Law of Demeter into the fabric of IT architecture, organizations can chart a course towards heightened efficiency, scalability, and maintainability of software systems. Java, with its versatile features and robust toolset, serves as a formidable ally in upholding these fundamental laws, paving the way for resilient and efficient IT architectures.