Transforming Your Business with Entity Services
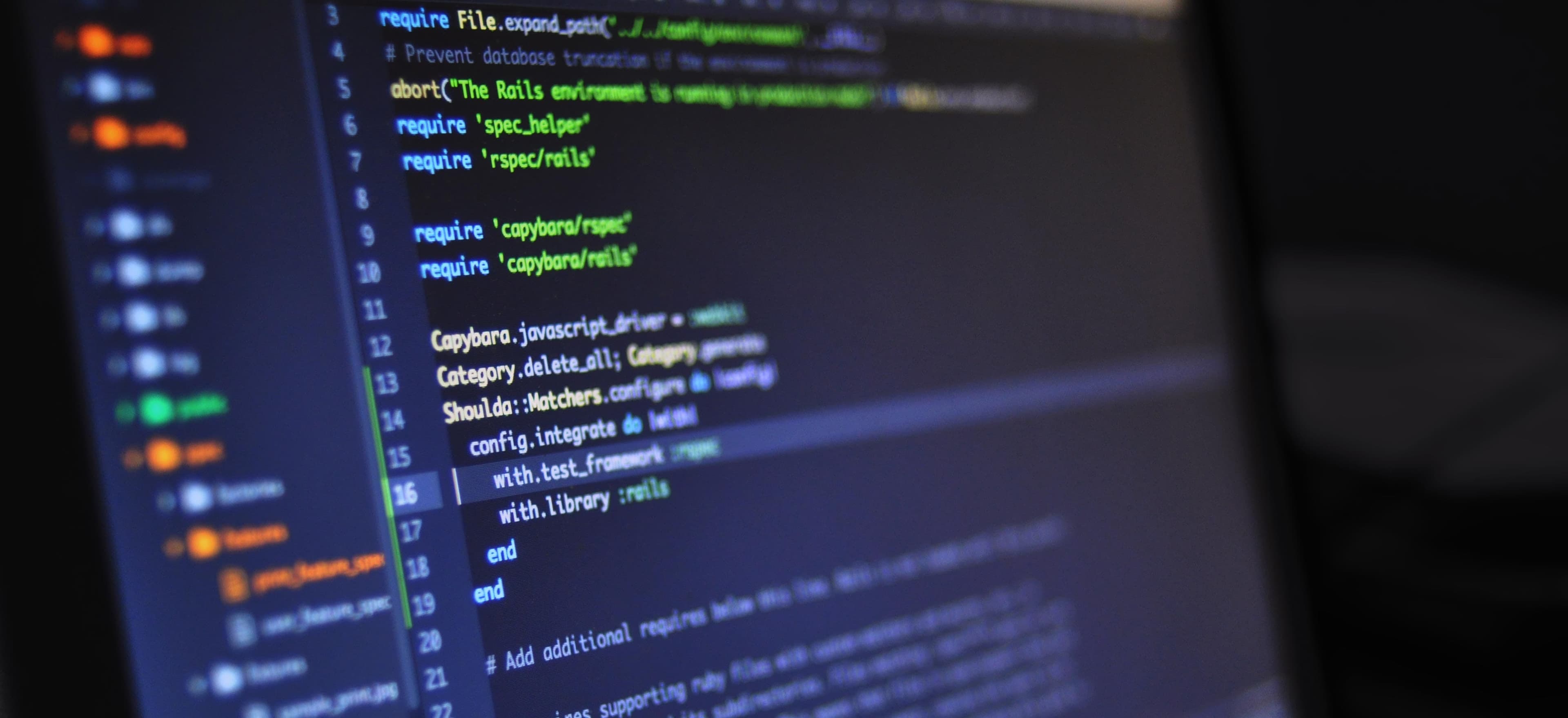
- Published on
Transforming Your Business with Entity Services
Are you looking to streamline your Java application by leveraging the power of Entity Services? In today's fast-paced business environment, it's crucial to optimize your data management to ensure seamless operations. The implementation of Entity Services in Java can revolutionize the way your business handles data, leading to improved efficiency and productivity.
What are Entity Services?
In the world of Java programming, an Entity Service is a concept that revolves around managing and manipulating data entities. These entities could be anything from customer information to product details in a business application. By encapsulating the logic for data access, modification, and retrieval, Entity Services provide a centralized and standardized approach to interact with the underlying data model.
The Power of Entity Services
1. Simplified Data Interaction
One of the key benefits of using Entity Services is the simplification of data interaction. Instead of scattering data access and manipulation logic across different parts of your codebase, Entity Services consolidate these operations into a cohesive layer. This not only reduces code redundancy but also makes the data-related code more maintainable and easier to understand.
2. Enhanced Reusability
By encapsulating data operations within Entity Services, you can reuse these services across multiple parts of your application. Whether it's a web frontend, a mobile app, or a backend process, the same Entity Service can be leveraged, promoting reusability and minimizing code duplication. This not only saves development time but also fosters consistency in data management.
3. Business Logic Separation
In a well-structured application, it's essential to separate the business logic from data access concerns. Entity Services play a pivotal role in achieving this separation by isolating the data access code from the core business logic. As a result, your application becomes more modular, facilitating easier maintenance and extensibility.
Now that we understand the significance of Entity Services, let's delve into a practical example showcasing the implementation of an Entity Service in Java.
Implementing an Entity Service in Java
Consider a simplified scenario where we have a Product
entity that encapsulates details about various products in an e-commerce application. We'll create an ProductService
class to handle all operations related to managing product data.
public class ProductService {
private ProductRepository productRepository;
public ProductService(ProductRepository productRepository) {
this.productRepository = productRepository;
}
public Product getProductById(long productId) {
// Business logic such as validation or additional operations can be performed here
return productRepository.findById(productId);
}
public void createProduct(Product product) {
// Additional business logic before creating the product
productRepository.save(product);
}
// Additional methods for updating, deleting, or querying products
}
In this example, the ProductService
encapsulates the logic for interacting with the Product
entity. It delegates the actual data access operations to a ProductRepository
, which could be an interface implemented by a specific data access technology such as JPA, Hibernate, or JDBC.
Why Use an Entity Service?
-
Centralized Business Logic: The
ProductService
consolidates all business logic related to products, providing a centralized location for managing product-related operations. -
Flexibility: By encapsulating the data access logic, the
ProductService
can adapt to changes in the underlying data source without affecting the rest of the application. For example, switching from a relational database to a NoSQL database would only require changes in theProductRepository
implementation. -
Testability: Separating the data access logic into a dedicated service makes it easier to test. You can mock the
ProductRepository
for unit testing theProductService
without actually interacting with the database.
By employing Entity Services, you can transform the way your business application handles data, bringing about a host of benefits ranging from improved maintainability to enhanced reusability.
Final Considerations
In the realm of Java application development, the adoption of Entity Services can be a game-changer for businesses aiming to optimize their data management strategies. By consolidating data access logic, promoting reusability, and separating business concerns, Entity Services pave the way for more robust and maintainable applications.
If you're eager to take your Java application to the next level, incorporating Entity Services should be high on your priority list. Embracing this concept not only aligns with best practices in software architecture but also equips your business with a competitive edge in the dynamic landscape of modern application development.
So, are you ready to revolutionize your business with Entity Services? The power to transform your data management lies at your fingertips – seize it with Java Entity Services!