Converting Collections with JDK 8 Streams
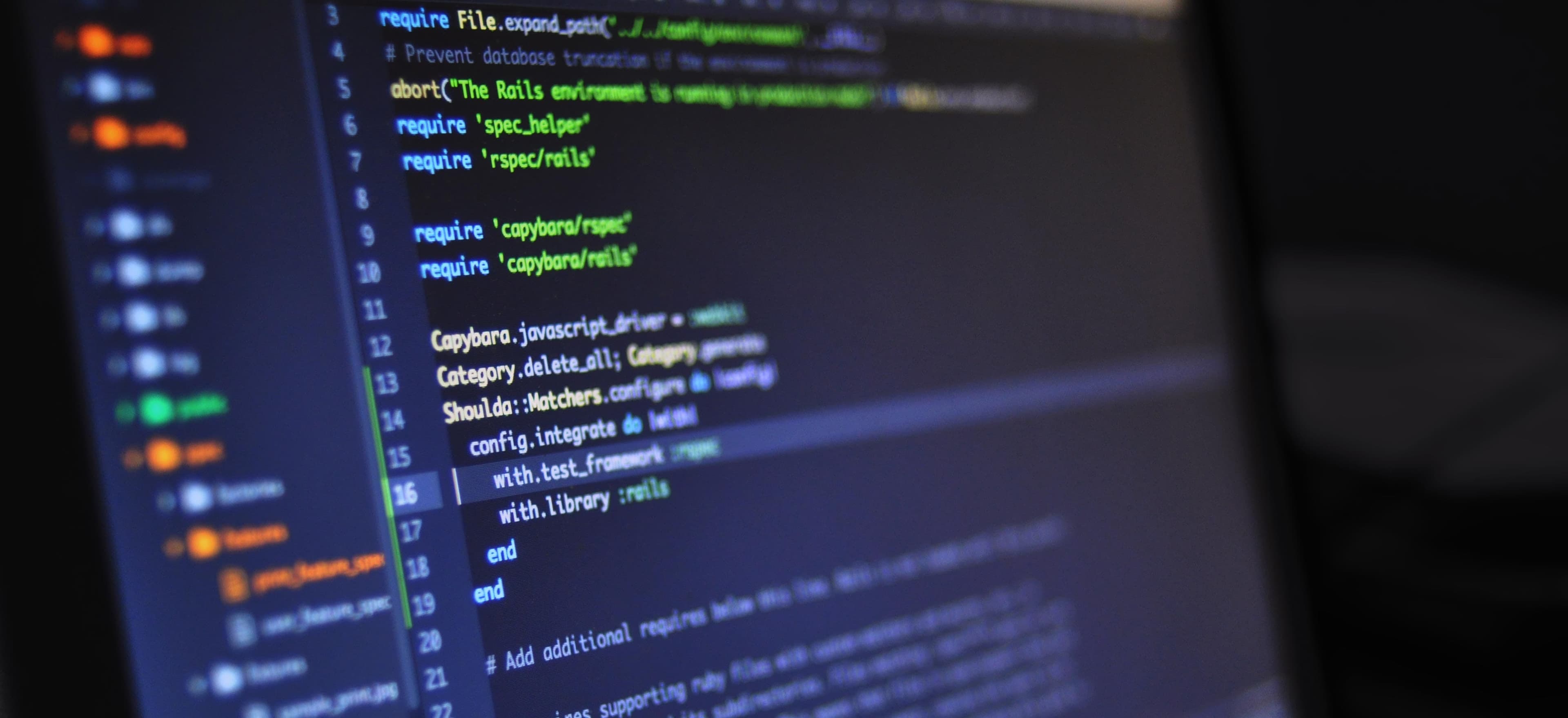
- Published on
Converting Collections with JDK 8 Streams
In Java programming, manipulating collections effectively is a critical skill. With the introduction of Java Development Kit (JDK) 8, a powerful feature called Streams was added to the Collections framework, allowing for functional-style operations on collections. This post aims to explore how to transform collections using JDK 8 Streams, providing insights, and code examples.
Understanding JDK 8 Streams
JDK 8 Streams provide a clean and concise way to perform operations such as filtering, mapping, and reducing on collections. This functional approach often leads to more readable and maintainable code compared to traditional for-each loops.
Benefits of JDK 8 Streams
- Conciseness: Streams allow for compact and expressive code.
- Readability: The functional approach improves code clarity.
- Parallelism: Streams offer easy parallelization of operations.
- Reusability: Stream operations can be composed in flexible ways.
Now, let's delve into examples that highlight how JDK 8 Streams can be used to convert collections efficiently.
Converting List to Map
One common operation is the transformation of a list into a map. Consider a scenario where you have a list of objects and want to create a map with each object's unique identifier as the key.
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class ListToMapExample {
public static void main(String[] args) {
List<User> userList = getUsers(); // Assume this method populates the list
Map<Long, User> userMap = userList.stream()
.collect(Collectors.toMap(User::getId, u -> u));
}
}
In the above snippet, the userList
is converted into a map using the Collectors.toMap
method. The User::getId
is used as the key, and each User
object itself is the value. This concise code demonstrates the power of Streams in transforming collections.
Why This Works
- Utilizing the
stream
method to begin the stream operation on the list. - Using the
collect
method along withCollectors.toMap
to construct the map.
Converting Map Keys to List
Conversely, it may be necessary to extract keys from a map and store them in a list. This can be achieved using Streams as depicted below:
import java.util.Map;
import java.util.List;
import java.util.stream.Collectors;
public class MapKeysToListExample {
public static void main(String[] args) {
Map<Integer, String> dataMap = getData(); // Assume this method provides the map
List<Integer> keyList = dataMap.keySet().stream()
.collect(Collectors.toList());
}
}
In this case, the keySet
of the map is transformed into a list using the Collectors.toList
collector. This concise and declarative approach showcases the elegance of Streams in manipulating collections.
Why This Works
- Accessing the
keySet
of the map via thekeySet
method. - Converting the Stream of keys into a list using the
collect
method.
Filtering and Collecting
Streams also facilitate filtering elements based on certain criteria and collecting the filtered results into a new collection. Let's consider an example where a list of users needs to be filtered based on their active status.
import java.util.List;
import java.util.stream.Collectors;
public class FilterAndCollectExample {
public static void main(String[] args) {
List<User> userList = getUsers(); // Assume this method returns a list of users
List<User> activeUsers = userList.stream()
.filter(User::isActive)
.collect(Collectors.toList());
}
}
In this scenario, the filter
operation is utilized to retain only the active users, and then, the collect
method is employed to gather the filtered results into a new list.
Why This Works
- Using the
filter
method to retain elements meeting the specified condition. - Employing the
collect
method to accumulate the filtered results into a new list.
Closing the Chapter
In conclusion, JDK 8 Streams offer a powerful and expressive way to transform collections in Java. The examples presented in this article demonstrate the versatility and elegance of Streams in performing common collection conversion tasks. By leveraging functional-style operations, code becomes more readable, maintainable, and concise. It is essential for developers to harness the capabilities of JDK 8 Streams for efficient collection manipulation.
For further understanding of Streams and Collections, refer to the official Java Documentation and the insightful article on Java Streams Best Practices.
Start incorporating JDK 8 Streams in your Java projects to unlock the potential of streamlined collection transformations!