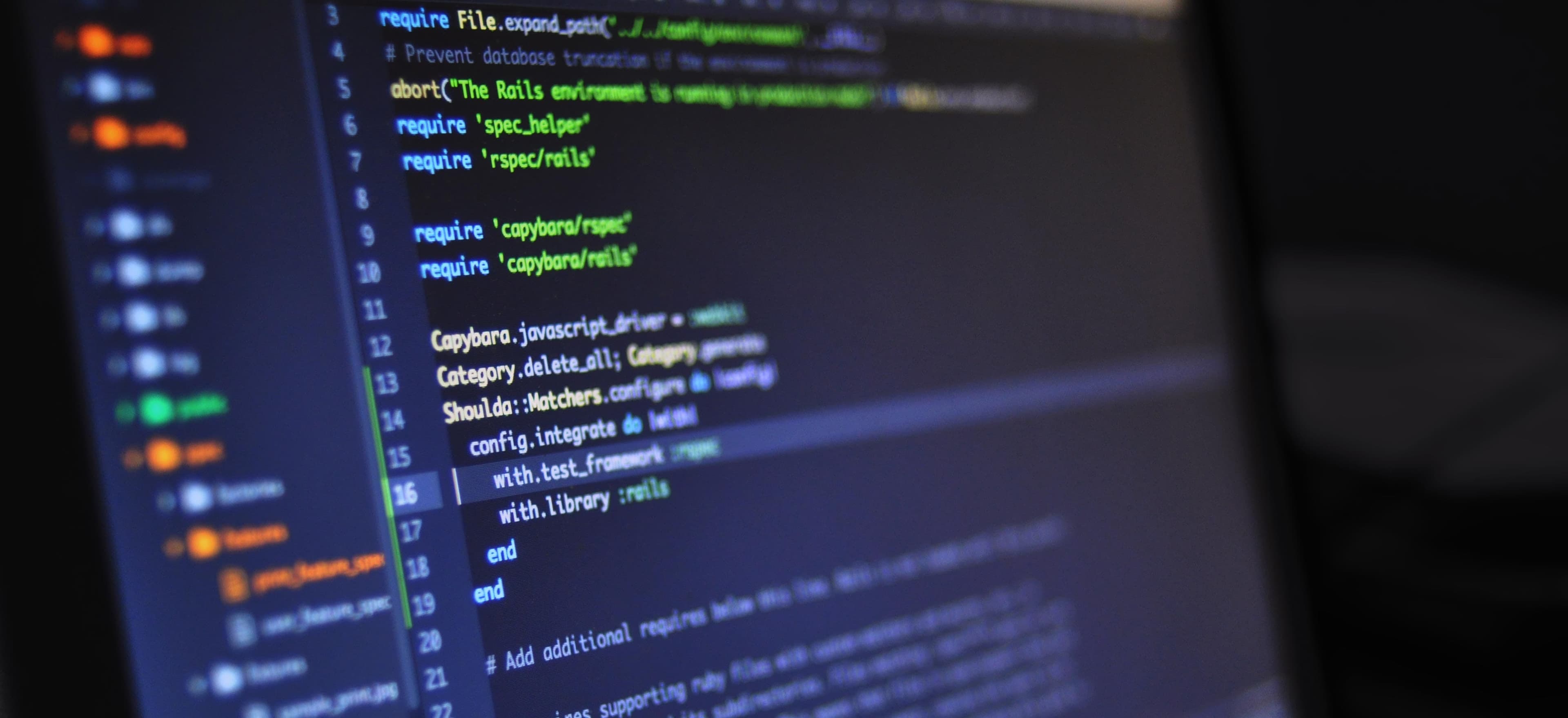
- Published on
Getting Started with Spring 3 MVC
If you are looking to develop web applications in Java, Spring MVC is a powerful framework that offers a flexible and organized way to build robust web applications. In this tutorial, we will walk through the process of setting up a simple "Hello World" web application using Spring 3 MVC.
Prerequisites
Before we begin, make sure you have the following:
- JDK installed on your system
- Apache Maven for building the project
- Your favorite IDE (Eclipse, IntelliJ, etc.)
Setting Up the Project
Create a new Maven project and add the necessary dependencies in the pom.xml
file. For this tutorial, we will be using Spring MVC, JSP for the view, and Apache Tomcat as the embedded servlet container.
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.2.18.RELEASE</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
</dependencies>
Creating the Controller
Next, let's create our controller class. This class will handle incoming requests and return the appropriate response. In this case, it will simply return the "Hello World" message.
package com.example.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class HelloWorldController {
@RequestMapping(value = "/hello", method = RequestMethod.GET)
public String helloWorld() {
return "hello";
}
}
In the code above, we've annotated the HelloWorldController
class with @Controller
to indicate that it's a controller component. The @RequestMapping
annotation specifies the URL at which the helloWorld
method will be invoked.
Creating the View
Now, let's create a simple JSP file that will display our "Hello World" message. Create a file named hello.jsp
in the WEB-INF/views
directory of your web application.
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>Hello World!</h1>
</body>
</html>
Configuring the Dispatcher Servlet
To configure the Dispatcher Servlet, create a file named spring-servlet.xml
in the WEB-INF
directory. This file will define the Spring MVC configuration and map the incoming requests to the appropriate controllers.
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/"/>
<property name="suffix" value=".jsp"/>
</bean>
<bean class="org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping"/>
<bean class="org.springframework.web.servlet.mvc.annotation.AnnotationMethodHandlerAdapter"/>
</beans>
In this configuration file, we've defined an InternalResourceViewResolver
to map the logical view names to the actual JSP files. We've also included the necessary handler mappings and handler adapters.
Configuring web.xml
Finally, we need to configure the Dispatcher Servlet in the web.xml
file. We'll also define the welcome file to be served when the application is accessed.
<web-app>
<display-name>HelloWorld</display-name>
<servlet>
<servlet-name>spring</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>spring</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
</web-app>
Build and Run the Application
With everything set up, you can now build the project using Maven and run it on an embedded Tomcat server. Once the application is running, access http://localhost:8080/hello
in your browser, and you should see the "Hello World" message displayed.
Congratulations! You have successfully created a simple web application using Spring 3 MVC.
Wrapping Up
In this tutorial, we covered the basics of setting up a Spring 3 MVC application and creating a simple "Hello World" web page. Spring MVC provides a powerful and organized framework for building web applications in Java, and with a solid understanding of its components, you can create robust and maintainable web applications.
To further enhance your understanding of Spring MVC, you can explore more advanced topics such as form handling, validation, and database integration. Additionally, consider exploring the official Spring Framework documentation for in-depth insights into Spring MVC best practices and advanced features.
Happy coding!