Debugging Java and Scala Code: Tips for Developers
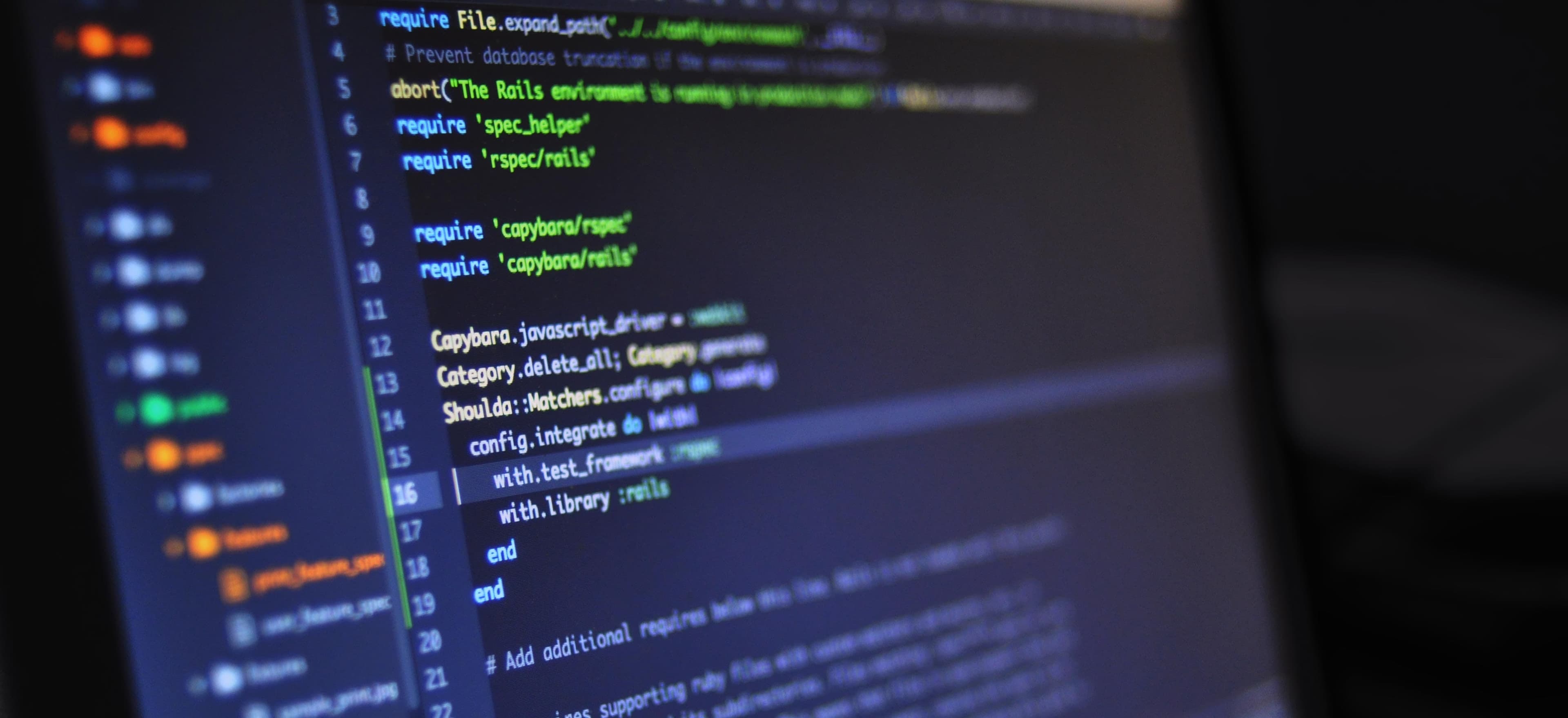
- Published on
Debugging Java and Scala Code: Tips for Developers
Debugging is an essential skill for developers, and it plays a crucial role in the software development process. Whether you are working with Java or Scala, effective debugging can save you time and effort while ensuring the quality and reliability of your code. In this article, we will explore some valuable tips and techniques for debugging Java and Scala code.
Understanding the Basics of Debugging
Before diving into specific debugging tips, let's review the fundamentals of debugging. At its core, debugging is the process of identifying and fixing errors, or bugs, within a software application. It involves tracing the execution of the code, inspecting variables, and analyzing the program's behavior to pinpoint and resolve issues.
Leveraging Integrated Development Environments (IDEs)
IDEs such as IntelliJ IDEA, Eclipse, and NetBeans provide powerful debugging tools that can significantly enhance your debugging experience. From setting breakpoints and stepping through code to inspecting variables and evaluating expressions, IDEs offer a rich set of features to streamline the debugging process.
public class DebugExample {
public static void main(String[] args) {
int x = 10;
int y = 5;
int z = x + y; // Set a breakpoint here
System.out.println("The sum is: " + z);
}
}
When using an IDE, setting a breakpoint at the indicated line allows you to pause the program's execution and examine the values of variables, gaining insight into the code's flow and behavior.
Utilizing Logging Frameworks
Logging frameworks, such as Log4j and Logback, are valuable tools for capturing runtime information and diagnosing issues in Java and Scala applications. By strategically placing log statements throughout your code, you can track the program's execution and gain visibility into the internal state of the application.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class LoggingExample {
private static final Logger LOGGER = LoggerFactory.getLogger(LoggingExample.class);
public static void main(String[] args) {
int dividend = 10;
int divisor = 0;
LOGGER.debug("Attempting division");
if (divisor == 0) {
LOGGER.error("Division by zero");
} else {
int result = dividend / divisor;
LOGGER.info("Result of division: " + result);
}
}
}
In the example above, the use of logging statements provides insight into the division operation, including any potential issues such as division by zero.
Employing Exception Handling
Exception handling is an integral part of writing robust and reliable code. By catching and handling exceptions, you can gracefully manage unexpected errors and prevent them from causing application failures. In Java and Scala, the try-catch-finally
construct allows you to capture exceptions and execute cleanup logic as needed.
public class ExceptionHandlingExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3};
int index = 3;
try {
int value = numbers[index]; // Potential IndexOutOfBoundsException
System.out.println("Value at index " + index + ": " + value);
} catch (IndexOutOfBoundsException e) {
System.err.println("Index out of bounds: " + e.getMessage());
} finally {
// Clean up resources, if necessary
}
}
}
In this code snippet, the try-catch
block captures the IndexOutOfBoundsException
that may occur when accessing an element beyond the array's bounds, ensuring a graceful handling of the error.
Using Debugging Tools
In addition to IDEs, logging frameworks, and exception handling, various debugging tools and utilities can aid in diagnosing and resolving issues within Java and Scala code. Tools like JDB (Java Debugger), VisualVM, and JConsole provide insights into memory usage, thread behavior, and performance metrics, enabling you to identify bottlenecks and optimize your applications.
Leveraging Unit Testing for Debugging
Unit testing not only validates the correctness of your code but also serves as an effective debugging technique. Writing comprehensive unit tests with frameworks like JUnit and ScalaTest allows you to systematically verify individual components of your code and uncover defects early in the development cycle.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MathUtilsTest {
@Test
public void testAddition() {
MathUtils mathUtils = new MathUtils();
int result = mathUtils.add(3, 5);
assertEquals(8, result);
}
}
By writing unit tests for critical functionality, you can proactively identify and address issues, ensuring the stability and correctness of your codebase.
Collaborating with Peers and Seeking Support
Debugging can be a collaborative effort, especially when dealing with complex issues. Leveraging the expertise of your colleagues, participating in code reviews, and seeking support from developer communities can provide valuable insights and fresh perspectives on debugging challenges.
Lessons Learned
Debugging Java and Scala code is an essential skill for developers, and mastering effective debugging techniques can greatly improve the quality and reliability of your software applications. By utilizing IDEs, logging frameworks, exception handling, debugging tools, unit testing, and collaborative approaches, developers can efficiently diagnose and resolve issues, ultimately delivering robust and maintainable code.
In closing, continuous improvement and honing of debugging skills are vital for every developer, as they contribute to the creation of higher-quality and more resilient software solutions.
For further insights on Java and Scala development, check out our comprehensive guide on advanced Java programming and an in-depth tutorial on functional programming with Scala.
Remember, effective debugging is not just about fixing bugs – it's about honing your problem-solving skills and enhancing the overall craftsmanship of your code. Happy debugging!