Solving Common Spring JSF Integration Exceptions
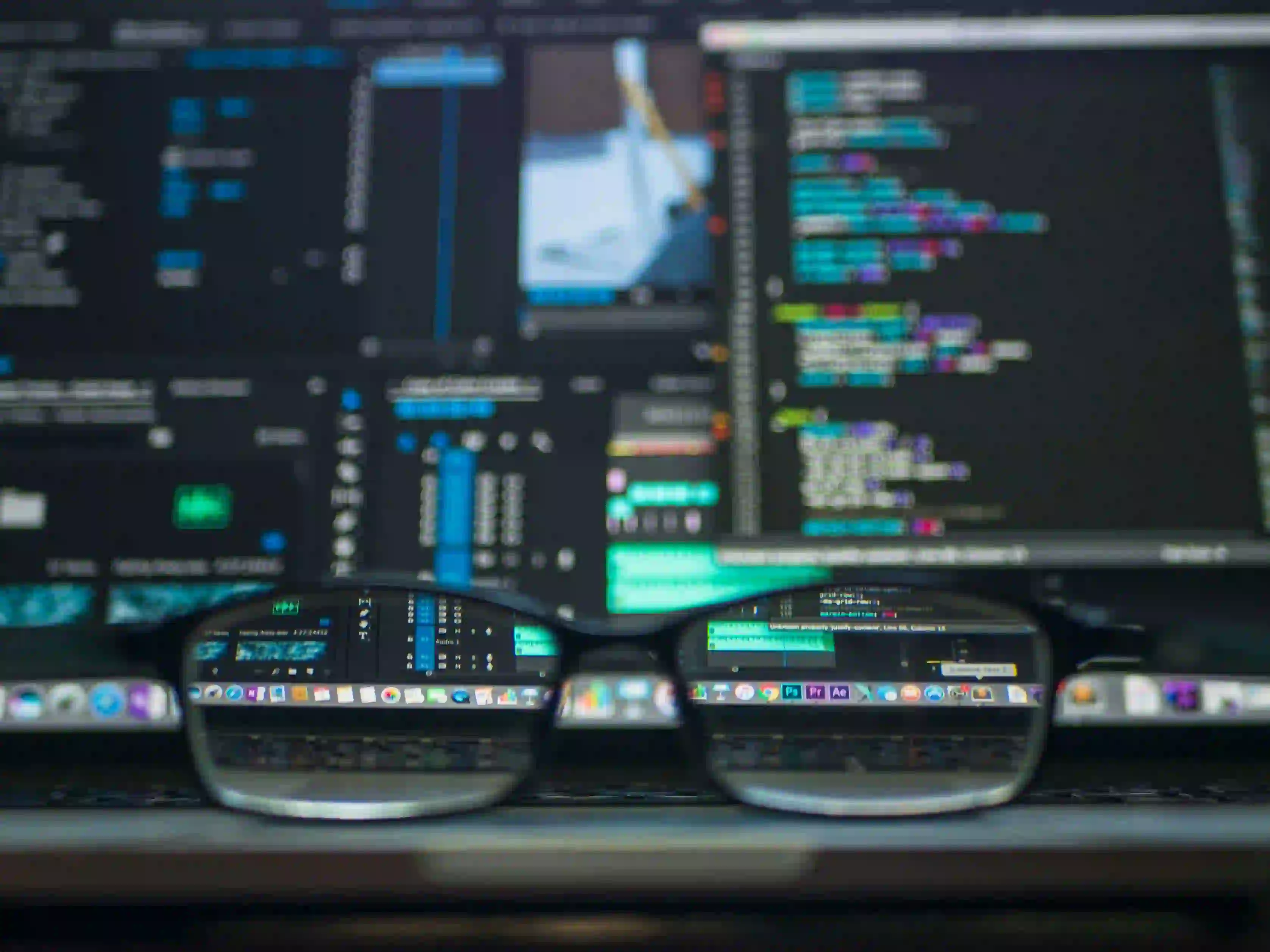
Solving Common Spring JSF Integration Exceptions
Integrating JavaServer Faces (JSF) with Spring can greatly enhance your web application's capabilities by leveraging Spring's powerful features such as Dependency Injection and Transaction Management. However, this integration is not without its challenges. In this blog post, we will explore some common Spring JSF integration exceptions and provide clear solutions to these problems.
Understanding Spring and JSF Integration
Before delving into the exceptions, it’s essential to understand what Spring and JSF bring to the table. JSF is a component-based web framework for building user interfaces for Java web applications, while Spring facilitates the development of Java applications through powerful and versatile infrastructure support.
When combined, Spring can manage JSF backing beans, improve manageability, and facilitate cleaner architecture.
<!-- Example of Spring's XML configuration -->
<bean id="myBean" class="com.example.MyBean" scope="request"/>
In the example above, we declare a Spring-managed bean with a request scope. This bit of configuration is the bedrock that allows Spring to work with JSF managed beans.
Common Integration Exceptions
Now let's dive into some common exceptions that developers encounter during the Spring and JSF integration:
1. NoSuchBeanDefinitionException
Issue Explanation: This exception occurs when Spring cannot find a bean definition for a requested bean.
Common Cause: It often arises because the bean is not annotated correctly or not scanned by the Spring context.
Solution: Ensure that your Spring configuration is set up for component scanning or that the bean is correctly defined in your configuration file.
@Configuration
@ComponentScan(basePackages = "com.example")
public class AppConfig {
}
This setup allows Spring to find and instantiate your beans.
2. NonUniqueBeanDefinitionException
Issue Explanation: This exception occurs when Spring finds multiple beans of the same type and doesn’t know which one to wire.
Common Cause: It can happen due to having multiple beans of the same type defined in the context.
Solution: You can either qualify your bean or change your configuration to avoid duplicates. For instance:
@Bean
@Primary
public MyBean myPrimaryBean() {
return new MyBean();
}
@Bean
public MyBean anotherBean() {
return new MyBean();
}
By marking one of the beans as @Primary
, you inform Spring which bean should be chosen when it encounters ambiguity.
3. FacesContextException
Issue Explanation: This exception is often thrown when there's an issue with the JSF lifecycle or when a FacesContext cannot be created.
Common Cause: A common cause could be an improper context initialization or issues with the web.xml configuration.
Solution:
Ensure that your faces-config.xml
and web.xml
files are correctly defined. An example of a typical web.xml
configuration for JSF might look like this:
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
Make sure that your servlet mapping and any other settings correctly align with your project structure.
4. ValueExpression Exception
Issue Explanation: This exception arises when JSF is unable to evaluate a value expression, often due to a missing bean or a property.
Common Cause: It typically happens if a JSF page references a property that is not available in the backing bean.
Solution: Check the backing bean and ensure that the property is correctly defined. For example:
@ManagedBean
public class UserBean {
private String username;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
}
If username
is referenced in a JSF page and doesn’t exist, the application will throw this exception.
5. Circular Dependency
Issue Explanation: Circular dependencies occur when two beans depend on each other, causing an infinite loop during instantiation.
Common Cause: It may arise from your wiring strategy or configuration, which can inadvertently create a loop.
Solution: To break the circular dependency, consider refactoring your code. One common approach is to redesign your beans to remove direct dependencies.
For instance, instead of directly referencing the bean:
@Autowired
private AnotherBean anotherBean; // This may cause circular dependency
Use setter injection instead:
private AnotherBean anotherBean;
@Autowired
public void setAnotherBean(AnotherBean anotherBean) {
this.anotherBean = anotherBean;
}
Setter injection allows for the circular reference to be resolved, as the beans can exist without being instantiated immediately.
6. Context Load Exceptions
Issue Explanation: Context load exceptions can occur if the application context cannot be initialized properly.
Common Cause: This often happens when there's an invalid configuration file or missing dependencies.
Solution:
Always double-check your configuration files and the dependencies added to your build configuration (pom.xml
for Maven or build.gradle
for Gradle). Make sure all necessary libraries are included.
Here’s a Maven dependency example:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.12</version>
</dependency>
Additional Tips for Troubleshooting
- Enable logging: Set log levels to debug for both Spring and JSF.
- Refer to documentation: The official Spring Framework and JSF Documentation are excellent resources.
- Clean and rebuild: Sometimes, simply cleaning the project and rebuilding can resolve classpath and dependency issues.
- Framework Versions: Ensure that you are using compatible versions of Spring and JSF, as mismatched versions often lead to unexpected behaviors.
My Closing Thoughts on the Matter
Integrating JSF with Spring can significantly enhance your Java web applications. However, understanding and troubleshooting common exceptions is crucial for a smooth development experience. By referring to this guide, you should be able to diagnose and solve many common integration issues with relative ease.
Consider it as an ongoing learning process—as you integrate these powerful frameworks, you will continuously find new challenges. But with the right strategies, you can overcome them and develop robust web applications.
For more detailed information, don’t forget to check the official Spring Reference Documentation and JSF Documentation. Happy coding!