Understanding the Storage of Interned Strings
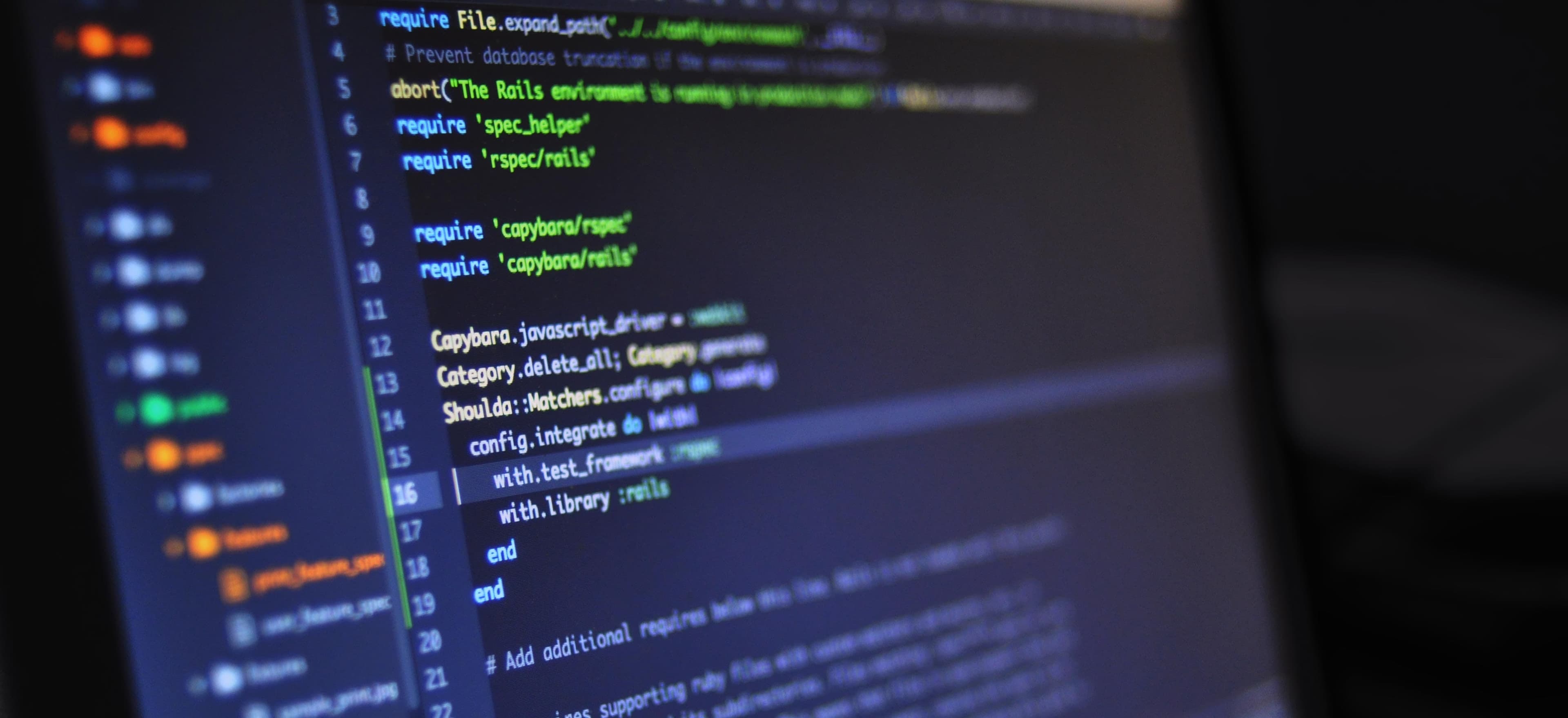
- Published on
When working with Java, especially in the realm of string manipulation, it's crucial to have a solid understanding of how interned strings are stored. Interned strings are a special case in which multiple references to the same string literal point to the same memory location. This has important implications for memory management and can impact the performance of your application.
What is String Interning?
In Java, when a string is created using double quotes, it is automatically interned by the JVM. For example:
String s1 = "hello";
String s2 = "hello";
In this case, both s1
and s2
point to the same memory location, as the string "hello" is interned. This means that when comparing s1
and s2
for equality, the result will be true
because they are referencing the same interned string.
Why Use String Interning?
One of the main reasons to use string interning is to conserve memory. By ensuring that only one copy of each distinct string value exists, interned strings can significantly reduce the memory footprint of an application.
Another benefit of string interning is performance optimization. Since interned strings refer to the same memory location, comparisons for equality can be done using reference equality (==
) rather than value equality (equals()
). This can lead to faster comparison operations, which is especially useful in scenarios where string comparisons are performed frequently.
How are Interned Strings Stored?
Interned strings in Java are stored in a special memory area called the "string pool." The string pool is a part of the heap memory and is maintained by the JVM. When a string is interned, the JVM first checks if the string already exists in the pool. If it does, the existing reference to the string is returned. If not, the string is added to the pool and its reference is returned.
Example of String Interning
Let's take a look at an example to illustrate the concept of string interning and how it affects memory usage:
String s1 = "hello";
String s2 = "hello";
String s3 = new String("hello").intern();
In this example, s1
and s2
both refer to the same interned string in the string pool. However, s3
is explicitly interned using the intern()
method. As a result, s1
, s2
, and s3
all refer to the same memory location, despite s3
being created using the new
keyword.
When to Use String Interning
String interning is particularly useful when dealing with a large number of string literals or when memory efficiency is a concern. It is commonly used in applications that involve heavy string manipulation, such as parsing large data sets or processing textual information.
However, it's important to exercise caution when interning strings, as it can lead to unexpected memory retention if not used judiciously. Additionally, the automatic interning of string literals by the JVM means that interned strings are effectively held in memory for the duration of the application, which may not be desirable in all scenarios.
Best Practices for String Interning
When using string interning, it's advisable to follow these best practices:
-
Be mindful of memory usage: While string interning can reduce memory usage, it's essential to monitor the size of the string pool, especially in long-running applications. Excessive string interning can lead to increased memory consumption if not managed carefully.
-
Consider the usage pattern: Evaluate the frequency of string comparisons and the potential benefits of using interned strings. If string comparisons are infrequent or if the strings being compared are mostly unique, the benefits of interning may be minimal.
-
Use
intern()
judiciously: Avoid indiscriminate use of theintern()
method, as it can lead to unnecessary string pooling and potential memory overhead. Only intern strings when there is a clear advantage in terms of memory or performance.
To Wrap Things Up
In conclusion, understanding how interned strings are stored in Java is essential for efficient memory management and performance optimization. By leveraging string interning judiciously, developers can mitigate memory overhead and improve the speed of string comparisons within their applications.
As with any optimization technique, it's crucial to weigh the benefits against the potential drawbacks and consider the specific requirements of the application at hand. String interning is a powerful tool, but it should be used with care and a clear understanding of its implications on memory usage and performance.
For further exploration of string management in Java, consider diving into the official Java String documentation and exploring how string interning fits into the broader context of string handling in Java.