Troubleshooting Java: Solving Common Programming Errors
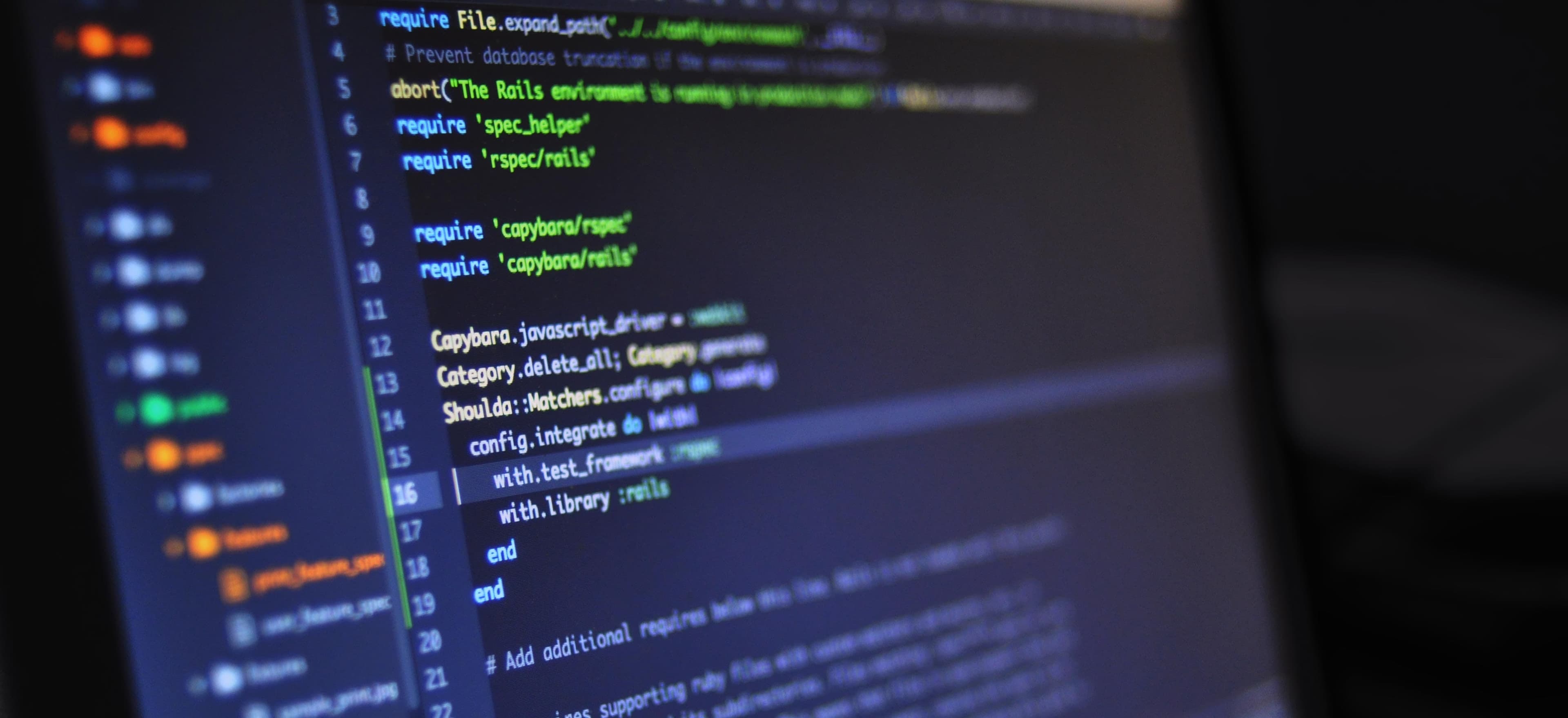
- Published on
Troubleshooting Java: Solving Common Programming Errors
Java is a powerful and versatile programming language, but like any language, it has its quirks and challenges. Even the most experienced Java developers encounter errors in their code. Understanding the common errors and knowing how to troubleshoot them is an essential skill for any Java programmer. In this article, we will explore some of the most common programming errors in Java and discuss how to solve them.
1. NullPointerException
The NullPointerException
is one of the most notorious errors in Java. It occurs when you try to access a method or property of a null object. This usually happens when you forget to initialize an object before using it, or when the object failed to initialize due to an exception.
Solution
To solve the NullPointerException
, you should always check if an object is null before attempting to access its methods or properties. You can use an if
statement or the Objects.requireNonNull()
method to ensure that the object is not null before using it.
String text = null;
if (text != null) {
// Do something with text
}
In situations where you expect an object not to be null, consider using assertions to catch unexpected null values during development.
2. ArrayIndexOutOfBoundsException
The ArrayIndexOutOfBoundsException
occurs when you try to access an index that is outside the bounds of an array. This error is common when iterating through arrays or collections.
Solution
To prevent the ArrayIndexOutOfBoundsException
, always ensure that the index is within the bounds of the array before accessing it.
int[] numbers = {1, 2, 3};
int index = 3;
if (index >= 0 && index < numbers.length) {
int value = numbers[index];
// Do something with value
}
When iterating through arrays or collections, use the enhanced for loop or Iterator
to avoid manual index management.
3. ClassCastException
The ClassCastException
occurs when you try to cast an object to a type that it is not compatible with. This error commonly arises when working with collections and inheritance.
Solution
To avoid the ClassCastException
, use the instanceof
operator to check the type of an object before casting it.
Object obj = "Hello";
if (obj instanceof String) {
String text = (String) obj;
// Do something with text
}
When working with collections, consider using parameterized types (generics) to enforce type safety and eliminate the need for explicit casting.
4. NoSuchMethodError
The NoSuchMethodError
occurs when a class references a method that does not exist in its runtime environment. This can happen when a class is compiled with a certain method and then linked against a version of the class that does not contain that method.
Solution
To resolve the NoSuchMethodError
, ensure that the method being called actually exists in the target class. Additionally, verify the classpath and dependencies to ensure that the correct versions of the classes are being used.
Using dependency management tools like Maven or Gradle can help prevent version mismatches and ensure that the correct versions of libraries are used in the project.
5. ConcurrentModificationException
The ConcurrentModificationException
occurs when an object, such as a collection, is modified concurrently while it is being iterated. This typically happens when trying to modify a collection directly while iterating through it with an iterator.
Solution
To avoid the ConcurrentModificationException
, use an explicit iterator or the Iterator
interface's remove()
method to modify the collection during iteration.
List<String> items = new ArrayList<>();
Iterator<String> iterator = items.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if (shouldRemove(item)) {
iterator.remove(); // Use iterator's remove method
}
}
Consider using thread-safe collections or synchronizing access to collections when dealing with concurrent modifications from multiple threads.
6. StackOverflowError
The StackOverflowError
occurs when the stack in the JVM runs out of space due to excessive recursion or deep method call chains.
Solution
To address the StackOverflowError
, optimize recursive algorithms to minimize unnecessary recursion and ensure that termination conditions are properly implemented.
In cases of deep method call chains, consider refactoring the code to reduce the depth of the call hierarchy or optimize the use of resources within the methods.
Closing Remarks
Encountering errors is an inevitable part of the programming process, and Java is no exception. By understanding the common errors and their solutions, you can become a more effective Java programmer. Remember to always test your code thoroughly and leverage the built-in tools and best practices to catch and prevent errors early in the development cycle.
In conclusion, being proactive in preventing errors, utilizing proper error-handling techniques, and staying informed about best practices in Java development can help you mitigate and solve common programming errors effectively.
Remember, troubleshooting errors is an opportunity to improve your problem-solving skills and enhance your understanding of the Java language and its nuances.
In this deep dive into common programming errors in Java, we explored practical solutions to prevalent issues encountered by Java developers. By understanding the root causes of these errors and implementing the recommended solutions, you can effectively troubleshoot and resolve common Java programming errors in your projects.
Do you have experiences with addressing common programming errors in Java? Share your insights and solutions with us!
Remember, effective troubleshooting skills are essential for every Java developer, and by mastering these skills, you can build robust and reliable Java applications.
Learn more about Java programming error handling at Java Error Handling Best Practices.
For further exploration, check out our guide on building optimized Java applications: Optimizing Java Applications for Performance.