Handling Complex YAML Configurations in Spring Boot
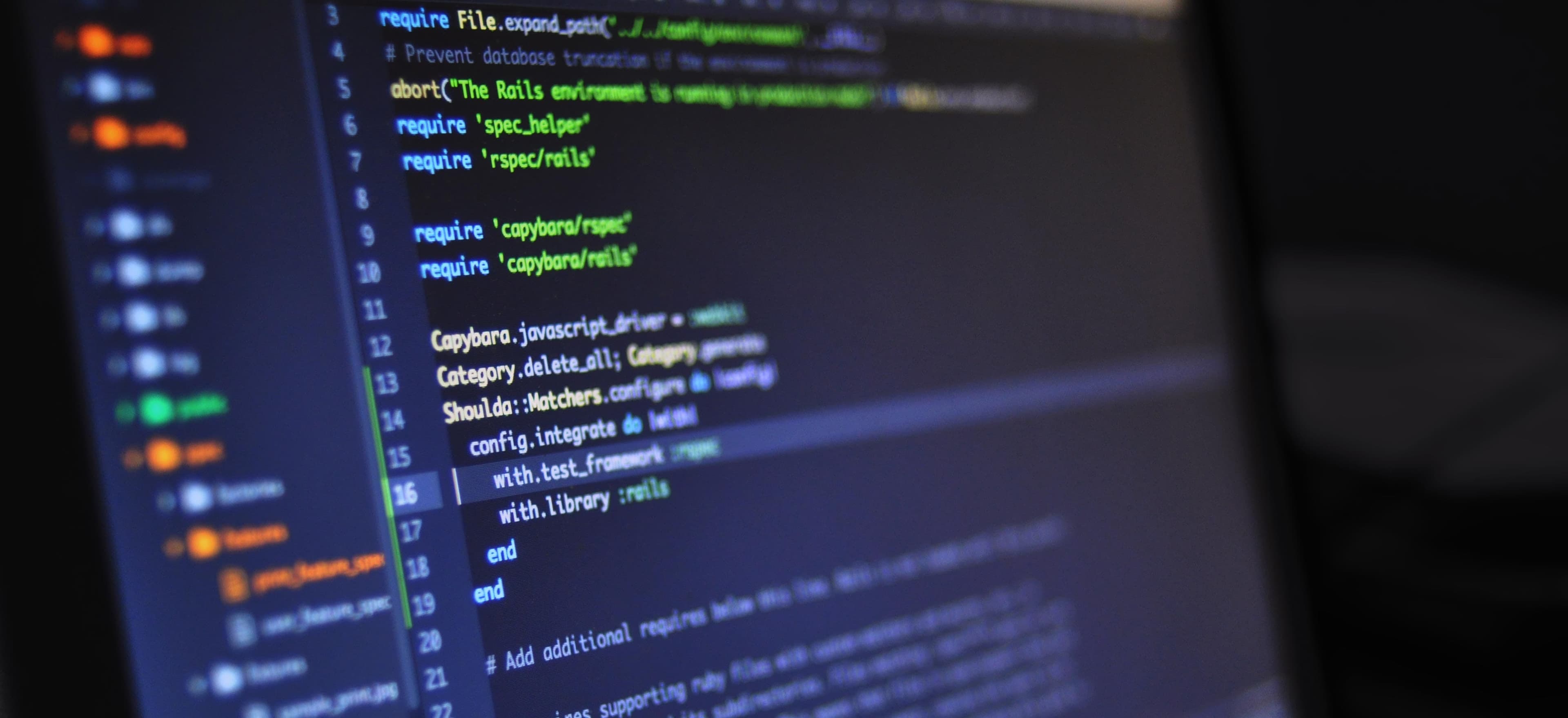
- Published on
Demystifying Complex YAML Configurations in Spring Boot
When working on a Spring Boot project, handling configuration is an essential aspect that demands precision. YAML (YAML Ain't Markup Language) configuration files have gained popularity owing to their human-readable format and structural simplicity, making them a preferred choice for many developers.
In this blog post, we will delve into handling complex YAML configurations in a Spring Boot application. We'll explore various scenarios and showcase best practices, guiding you through the process of effectively managing intricate configurations.
Why YAML?
YAML, with its clean and intuitive syntax, offers a compelling alternative to traditional property files. Its support for complex data structures, such as lists and maps, makes it an ideal choice for expressing application configurations. In the context of Spring Boot, YAML serves as a natural fit for defining properties and profiles.
Multi-Document Support
Let's start by discussing how to handle multi-document support in YAML. Spring Boot provides seamless integration for parsing multiple YAML documents in a single file. This feature is particularly handy when you want to encapsulate distinct configurations under a single umbrella.
Consider the following example:
# application.yml
server:
port: 8080
---
# additional-config.yml
sample:
key: value
In this scenario, server
configuration and sample
configuration are separated by ---
in the same file. Spring Boot effortlessly picks up these distinct documents, allowing the application to access them individually.
Profile-specific Configurations
Spring profiles enable environment-specific configurations, and YAML makes it easy to define and organize these profiles. By leveraging profiles, you can seamlessly manage configurations for different environments such as development, staging, and production.
Here's how you can structure your YAML file to accommodate profiles:
spring:
profiles:
active: dev
server:
port: 8080
---
spring:
profiles: prod
server:
port: 9090
In this example, we've defined configurations for both dev
and prod
profiles. When running the application with a specific profile, Spring Boot automatically picks up the corresponding configuration, providing a streamlined approach to managing environment-specific properties.
Property Hierarchy and Overriding
YAML supports a hierarchical structure that allows for nesting properties and overriding values at different levels. This feature becomes invaluable when dealing with a large set of configuration properties. Let's explore how property hierarchy and overriding work in a Spring Boot application.
Consider the following YAML configuration:
logging:
level:
com.example: DEBUG
server:
port: 8080
In this snippet, the application logs for com.example
are set to DEBUG
, and the server port is configured to 8080
. This illustrates a basic YAML structure with properties at the root level.
Now, let's introduce overriding:
logging:
level:
com.example: INFO
server:
port: 9090
In this updated configuration, the log level for com.example
has been overridden to INFO
, and the server port has been changed to 9090
. This simple yet powerful mechanism allows for easy customization and fine-grained control over configurations.
Using Lists and Maps
YAML's support for representing lists and maps simplifies the management of complex configurations. Spring Boot seamlessly integrates with YAML, enabling you to define and consume lists and maps within your application properties.
Let's consider an example where we define a list of values:
items:
- value1
- value2
- value3
In this configuration, the items
property encapsulates a list of values. When accessing these properties in your Spring Boot application, the list can be easily mapped to a corresponding Java data structure, such as a List
or an array.
Similarly, YAML's map representation facilitates the configuration of key-value pairs:
details:
name: John Doe
age: 30
address:
city: Example City
country: Example Country
In this snippet, the details
property comprises a map containing various key-value pairs. Spring Boot effortlessly transforms this structured data into corresponding Java objects, allowing for seamless consumption within the application.
Closing Remarks
In this post, we've explored the nuances of handling complex YAML configurations in a Spring Boot application. From multi-document support to profile-specific configurations, property hierarchy, and the use of lists and maps, YAML proves to be a robust choice for managing diverse sets of properties.
By leveraging YAML's expressive syntax and Spring Boot's seamless integration, you can effectively handle intricate configurations while maintaining clarity and conciseness in your application properties.
As you continue to refine your Spring Boot projects, mastering the art of YAML configurations will undoubtedly empower you to architect robust and adaptable systems.
For further insights on Spring Boot and YAML configurations, consider exploring the official Spring Boot documentation for comprehensive guidance.
Happy coding with Spring Boot and YAML!