Managing Dependency Injection in JPA Entity Listeners
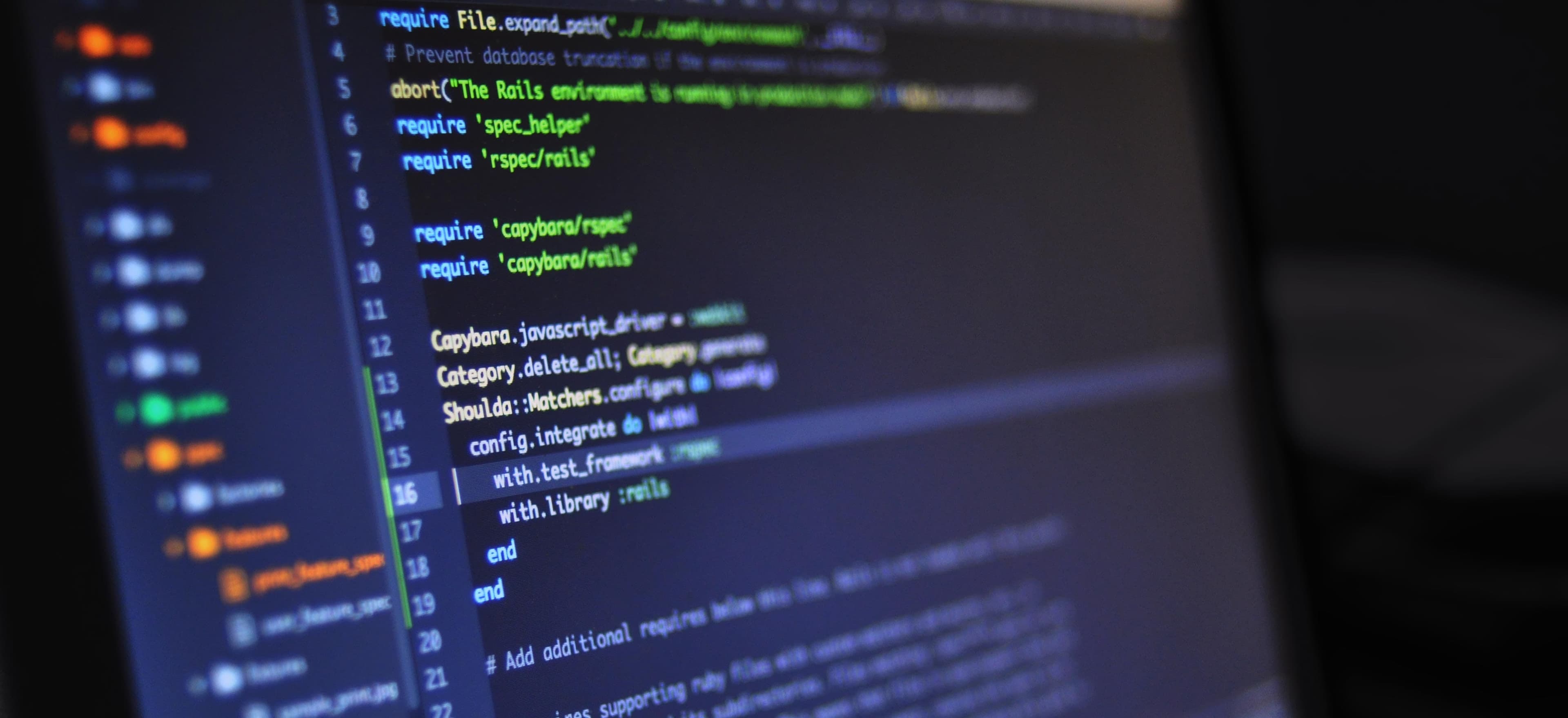
- Published on
Managing Dependency Injection in JPA Entity Listeners
In the world of Java Persistence API (JPA), entity listeners play a crucial role in capturing events related to entities, such as pre-persist, post-update, pre-remove, etc. They allow developers to execute custom logic before or after these events occur. However, managing dependency injection within JPA entity listeners can be a bit tricky, as they are not managed by the container or Spring framework by default.
In this article, we will explore how to effectively manage dependency injection in JPA entity listeners. We will discuss the challenges involved and provide solutions to ensure seamless integration of dependency injection within entity listeners.
Understanding the Challenge
JPA entity listeners are typically simple classes annotated with @EntityListeners
and registered to receive callback notifications for entity lifecycle events. While it is common to inject dependencies using @Autowired
or @Inject
annotations in other Spring-managed components, doing the same within entity listeners does not work out of the box. This is because JPA entity listeners are not Spring-managed beans, and thus, direct dependency injection is not supported.
So, the challenge lies in finding a way to inject dependencies into entity listeners without compromising the decoupling and reusability of the listener classes.
Solution 1: Using Spring's AutowireCapableBeanFactory
One approach to tackle this issue is by utilizing Spring's AutowireCapableBeanFactory
to programmatically perform dependency injection.
Let's consider an example where we have an entity listener that needs to make use of a service component for some business logic. First, we need to access the AutowireCapableBeanFactory
instance within the entity listener. We can achieve this by implementing ApplicationContextAware
in the entity listener class.
public class CustomEntityListener implements ApplicationContextAware {
private AutowireCapableBeanFactory beanFactory;
@Override
public void setApplicationContext(ApplicationContext context) throws BeansException {
beanFactory = context.getAutowireCapableBeanFactory();
}
@PrePersist
public void beforePersist(Object entity) {
// Perform dependency injection for required service
CustomService customService = beanFactory.getBean(CustomService.class);
// Use the customService for business logic
}
// Other lifecycle callback methods
}
In this example, the CustomEntityListener
class implements ApplicationContextAware
to gain access to the AutowireCapableBeanFactory
. With this, we can programmatically obtain the required dependency (CustomService
) and use it within the entity listener methods.
While this approach provides a way to achieve dependency injection within entity listeners, it introduces a tight coupling to the Spring framework, potentially impacting the portability and reusability of the entity listeners.
Solution 2: Using CDI (Context and Dependency Injection)
For applications leveraging Java EE or Jakarta EE, Context and Dependency Injection (CDI) can be utilized to manage dependency injection within JPA entity listeners. CDI brings the power of contextual lifecycle and dependency injection to various Java EE components, including JPA entity listeners.
To inject dependencies into an entity listener using CDI, we can simply use the @Inject
annotation on the fields for the dependencies.
public class CDICustomEntityListener {
@Inject
private CustomService customService;
@PrePersist
public void beforePersist(Object entity) {
// Use the customService for business logic
}
// Other lifecycle callback methods
}
By leveraging CDI, we can seamlessly inject dependencies into entity listeners without the need for additional configuration or programmatically accessing the bean factory. This leads to cleaner, more portable code and allows for easier testing and reuse of the entity listeners.
Closing Remarks
In the world of JPA, managing dependency injection within entity listeners requires careful consideration and approach. While Spring's AutowireCapableBeanFactory
can be used for programmatically injecting dependencies, it introduces tight coupling to the Spring framework. On the other hand, utilizing CDI in Java EE or Jakarta EE environments provides a more seamless and portable solution for dependency injection within entity listeners.
As you navigate the landscape of JPA entity listeners, remember to weigh the pros and cons of different approaches, and choose the one that best aligns with the architectural principles and requirements of your project.
By effectively managing dependency injection in JPA entity listeners, you can ensure that your entity lifecycle event handling remains robust, maintainable, and conducive to the overall design and structure of your application.
For further reading, check out Spring's documentation on managing beans and dependencies and Oracle's guide to Context and Dependency Injection to delve deeper into this topic.