Overcoming Imposter Syndrome in Automation Testing
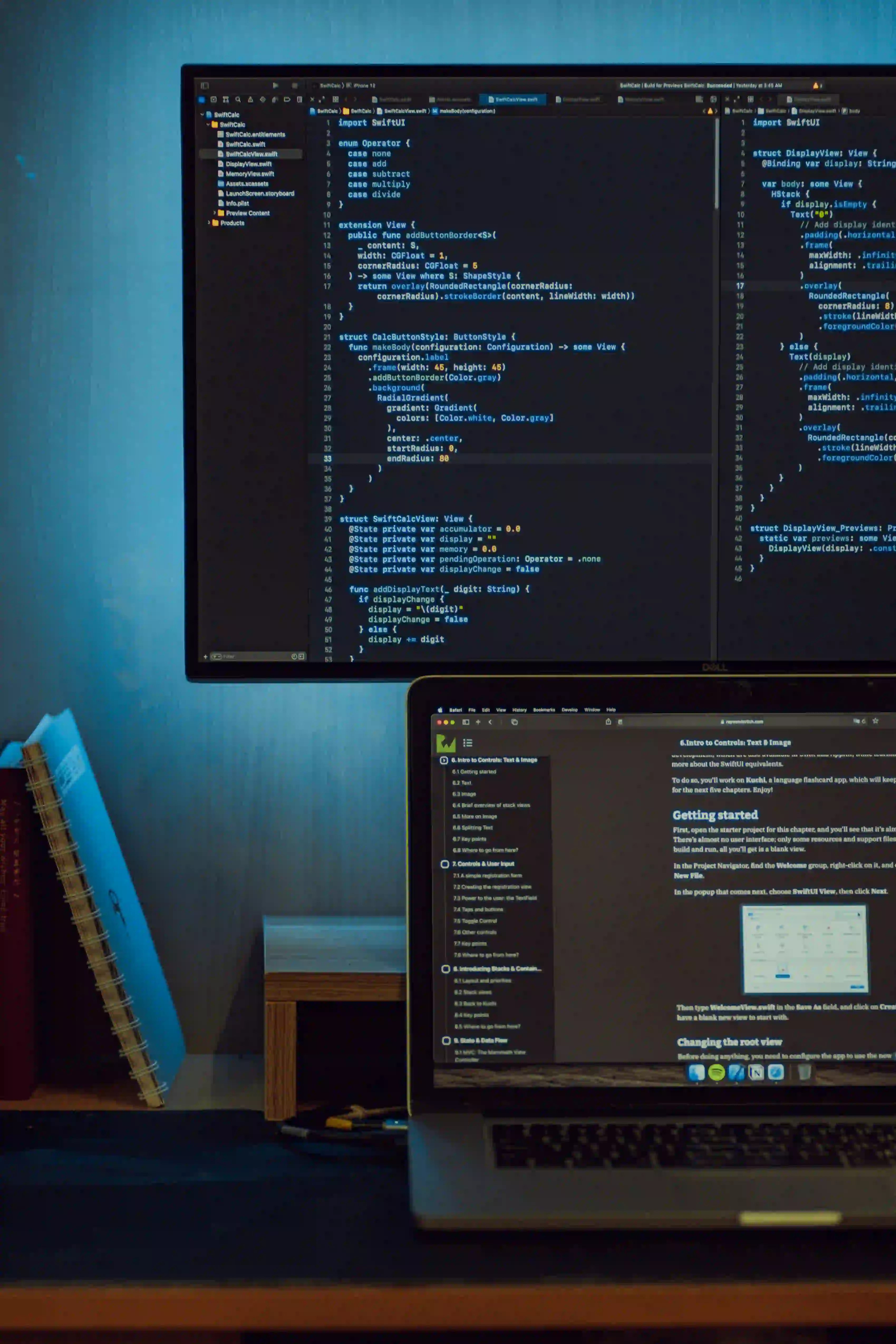
Overcoming Imposter Syndrome in Automation Testing
Imposter syndrome is a common feeling among programmers and automation testers. It's the sense that you're not as competent as others perceive you to be and that your success is down to luck rather than your own ability. This can be especially prevalent in the world of automation testing in Java due to the intricate nature of the language and the constant evolution of testing frameworks.
Embracing OOP in Java for Automation Testing
One way to overcome imposter syndrome in automation testing is to embrace the power of Object-Oriented Programming (OOP) in Java. OOP allows for the creation of reusable and maintainable code through concepts such as encapsulation, inheritance, and polymorphism.
public class LoginPageTests extends BaseTest {
@Test
public void successfulLoginTest() {
LoginPage loginPage = new LoginPage(driver);
HomePage homePage = loginPage.loginWithValidCredentials("username", "password");
Assert.assertTrue(homePage.isHomePageDisplayed());
}
}
In the above code snippet, the LoginPageTests class demonstrates the use of OOP by creating an instance of the LoginPage and HomePage classes to perform a successful login test. This approach fosters code reusability and reduces duplication.
Leveraging TestNG for Test Orchestration
TestNG is a testing framework that has gained popularity in the Java community due to its flexibility and ease of use. Leveraging TestNG can help automation testers organize and orchestrate their tests efficiently.
public class LoginTest {
@BeforeMethod
public void setUp() {
// Code for setting up the test environment
}
@Test
public void validLoginTest() {
// Test logic for valid login scenario
}
@AfterMethod
public void tearDown() {
// Code for tearing down the test environment
}
}
The above example showcases the use of TestNG's annotations like @BeforeMethod and @AfterMethod to set up and tear down the test environment, ensuring the tests are isolated and independent.
Embracing Continuous Integration with Jenkins
Continuous Integration (CI) is a software development practice where code changes are regularly integrated into a shared repository. Jenkins is a popular CI tool that can be used to automate the build, test, and deployment of Java automation tests.
pipeline {
agent any
stages {
stage('Build') {
steps {
// Code for building the project
}
}
stage('Test') {
steps {
// Code for running automated tests
}
}
stage('Deploy') {
steps {
// Code for deploying the application
}
}
}
}
In the Jenkins pipeline script above, the "Test" stage is responsible for running the automated tests as part of the CI process, ensuring that any code changes do not break existing functionality.
Seeking Learning Opportunities and Mentorship
Imposter syndrome often stems from a lack of confidence in one's abilities. Seeking out learning opportunities, whether through online courses, reading books, or attending workshops, can boost confidence and deepen understanding. Additionally, finding a mentor in the automation testing field can provide valuable guidance and support.
Embracing Failure as a Learning Opportunity
Failure is an inherent part of the learning process, especially in automation testing. Embracing failure as a learning opportunity can help alleviate imposter syndrome. Understanding that encountering challenges and making mistakes is a natural part of the learning journey can be empowering.
The Closing Argument
Imposter syndrome in automation testing is a common challenge that many professionals face. Embracing OOP, leveraging TestNG for test orchestration, embracing CI with Jenkins, seeking learning opportunities, mentorship, and embracing failure can all contribute to overcoming imposter syndrome and becoming a more confident and effective automation tester. Remember, it's okay to feel unsure at times, but it's essential to keep learning and growing in your automation testing journey.